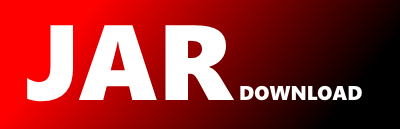
com.tecacet.finance.service.asset.BarchartAssetService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jfapi Show documentation
Show all versions of jfapi Show documentation
JFApi connects to various Web Services that provide financial data such as stock prices,
splits, dividends, and FX rates.
package com.tecacet.finance.service.asset;
import com.tecacet.finance.io.parser.BarchartAssetParser;
import com.tecacet.finance.model.Asset;
import com.tecacet.finance.model.AssetType;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Comparator;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
public class BarchartAssetService implements AssetService {
private static final String[] URLS =
{"http://datasrv.ddfplus.com/names/amex.txt", "http://datasrv.ddfplus.com/names/etf.txt", "http://datasrv.ddfplus.com/names/funds_fullname.txt",
"http://datasrv.ddfplus.com/names/nasd.txt", "http://datasrv.ddfplus.com/names/nyse.txt"};
private static final String[] FILENAMES = {"amex.txt", "etf.txt", "funds_fullname.txt", "nasd.txt", "nyse.txt"};
private static final boolean[] MUTUAL_FUND = {false, false, true, false, false};
private final BarchartAssetParser assetParser = new BarchartAssetParser();
private final boolean useOnline;
public BarchartAssetService() {
this(false);
}
public BarchartAssetService(boolean useOnline) {
super();
this.useOnline = useOnline;
}
@Override
public Set getAssets() throws IOException {
Set assets = new TreeSet<>(Comparator.comparing(Asset::getSymbol));
for (int i = 0; i < FILENAMES.length; i++) {
InputStream is = getStream(i);
List assetSet = assetParser.parse(is, getAssetType(i));
assetSet.stream().filter(a -> a.getSymbol() != null).forEach(assets::add);
is.close();
}
return assets;
}
private InputStream getStream(int i) throws IOException {
try {
return useOnline ? new URL(URLS[i]).openStream() : this.getClass().getClassLoader().getResourceAsStream("barchart" + File.separator + FILENAMES[i]);
} catch (MalformedURLException e) {
throw new IOException(e);
}
}
private AssetType getAssetType(int i) {
return MUTUAL_FUND[i] ? AssetType.MUTUAL_FUND : AssetType.STOCK;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy