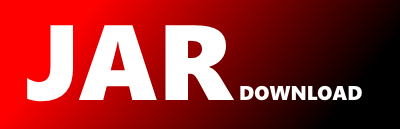
com.vectorprint.configuration.generated.parser.JavaCharStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Config Show documentation
Show all versions of Config Show documentation
A library for settings and parameterization of objects. Key features are support for data types,
help for settings and parameters, annotations for ease of use. Settings and parameters both are Clonable and Serializable.
More features for settings such as parsing
a settingsfile, being observable, readonliness, caching etc. are available. The library contains javacc generated
parsers for syntax support for properties, multi valued properties, parameterized objects and multi valued parameters.
/* Generated by: ParserGeneratorCC: Do not edit this line. JavaCharStream.java Version 1.1 */
/* ParserGeneratorCCOptions:SUPPORT_CLASS_VISIBILITY_PUBLIC=true */
package com.vectorprint.configuration.generated.parser;
/**
* An implementation of interface CharStream, where the stream is assumed to
* contain only ASCII characters (with java-like unicode escape processing).
*/
public
class JavaCharStream extends AbstractCharStream
{
/** Predefined buffer size */
protected static final int NEXTCHAR_BUF_SIZE = 4096;
private char[] m_aNextCharBuf;
private int nextCharInd = -1;
private java.io.Reader m_aIS;
@Override
protected int streamRead(final char[] buffer, final int offset, final int len) throws java.io.IOException
{
return m_aIS.read (buffer, offset, len);
}
@Override
protected void streamClose() throws java.io.IOException
{
m_aIS.close ();
}
@Override
protected void fillBuff() throws java.io.IOException
{
if (maxNextCharInd == NEXTCHAR_BUF_SIZE)
{
maxNextCharInd = 0;
nextCharInd = 0;
}
try
{
final int nCharsRead = streamRead (m_aNextCharBuf, maxNextCharInd, NEXTCHAR_BUF_SIZE - maxNextCharInd);
if (nCharsRead == -1)
{
streamClose ();
throw new java.io.IOException ();
}
maxNextCharInd += nCharsRead;
}
catch (final java.io.IOException ex)
{
if (bufpos != 0)
{
--bufpos;
backup(0);
}
else
internalSetBufLineColumn (getLine (), getColumn ());
throw ex;
}
}
private char readByte() throws java.io.IOException
{
++nextCharInd;
if (nextCharInd >= maxNextCharInd)
fillBuff();
return m_aNextCharBuf[nextCharInd];
}
@Override
public char beginToken() throws java.io.IOException
{
if (inBuf > 0)
{
--inBuf;
if (++bufpos == bufsize)
bufpos = 0;
tokenBegin = bufpos;
return buffer[bufpos];
}
tokenBegin = 0;
bufpos = -1;
return readChar();
}
@Override
public char readChar() throws java.io.IOException
{
if (inBuf > 0)
{
--inBuf;
++bufpos;
if (bufpos == bufsize)
{
// Buffer overflow
bufpos = 0;
}
return buffer[bufpos];
}
++bufpos;
if (bufpos == available)
internalAdjustBuffSize();
char c = readByte();
buffer[bufpos] = c;
if (c != '\\')
{
// Not a backslash
if (isTrackLineColumn())
internalUpdateLineColumn(c);
return c;
}
if (isTrackLineColumn())
internalUpdateLineColumn(c);
int backSlashCnt = 1;
// Read all the backslashes
for (;;)
{
++bufpos;
if (bufpos == available)
internalAdjustBuffSize();
try
{
c = readByte();
buffer[bufpos] = c;
if (c != '\\')
{
// found a non-backslash char.
if (isTrackLineColumn())
internalUpdateLineColumn(c);
if (c == 'u' && (backSlashCnt & 1) == 1)
{
--bufpos;
if (bufpos < 0)
{
// Buffer underflow
bufpos = bufsize - 1;
}
// Found the start of a Unicode sequence
break;
}
// Unsupported escape sequence
backup(backSlashCnt);
return '\\';
}
}
catch (final java.io.IOException e)
{
// We are returning one backslash so we should only backup (count-1)
if (backSlashCnt > 1)
backup (backSlashCnt - 1);
return '\\';
}
// We read another backslash
if (isTrackLineColumn())
internalUpdateLineColumn (c);
backSlashCnt++;
}
// Here, we have seen an odd number of backslash's followed by a 'u'
try
{
// Skip all 'u' as in '\uuuuuu1234'
while ((c = readByte()) == 'u')
{
if (isTrackLineColumn())
internalUpdateLineColumn(c);
}
final char c1 = c;
final char c2 = readByte();
final char c3 = readByte();
final char c4 = readByte();
c = (char) (hexval(c1) << 12 |
hexval(c2) << 8 |
hexval(c3) << 4 |
hexval(c4));
buffer[bufpos] = c;
if (isTrackLineColumn())
{
internalUpdateLineColumn(c1);
internalUpdateLineColumn(c2);
internalUpdateLineColumn(c3);
internalUpdateLineColumn(c4);
}
}
catch (final java.io.IOException ex)
{
throw new IllegalStateException("Invalid escape character at line " + getLine () + " column " + getColumn () + ".");
}
if (backSlashCnt == 1)
return c;
backup(backSlashCnt - 1);
return '\\';
}
/** Constructor. */
public JavaCharStream(final java.io.Reader dstream,
final int startline,
final int startcolumn,
final int buffersize)
{
super (startline, startcolumn, buffersize);
m_aNextCharBuf = new char[NEXTCHAR_BUF_SIZE];
m_aIS = dstream;
}
/** Constructor. */
public JavaCharStream(final java.io.Reader dstream,
final int startline,
final int startcolumn)
{
this(dstream, startline, startcolumn, DEFAULT_BUF_SIZE);
}
/** Constructor. */
public JavaCharStream(final java.io.Reader dstream)
{
this(dstream, 1, 1, DEFAULT_BUF_SIZE);
}
/** Reinitialise. */
public void reInit(final java.io.Reader dstream)
{
reInit(dstream, 1, 1, DEFAULT_BUF_SIZE);
}
/** Reinitialise. */
public void reInit(final java.io.Reader dstream,
final int startline,
final int startcolumn)
{
reInit(dstream, startline, startcolumn, DEFAULT_BUF_SIZE);
}
/** Reinitialise. */
public void reInit(final java.io.Reader dstream,
final int startline,
final int startcolumn,
final int buffersize)
{
m_aNextCharBuf = new char[NEXTCHAR_BUF_SIZE];
nextCharInd = -1;
m_aIS = dstream;
super.reInit (startline, startcolumn, buffersize);
}
/** Constructor. */
public JavaCharStream(final java.io.InputStream dstream,
final String encoding,
final int startline,
final int startcolumn,
final int buffersize) throws java.io.UnsupportedEncodingException
{
this(new java.io.InputStreamReader(dstream, encoding), startline, startcolumn, buffersize);
}
/** Constructor. */
public JavaCharStream(final java.io.InputStream dstream,
final String encoding,
final int startline,
final int startcolumn) throws java.io.UnsupportedEncodingException
{
this(dstream, encoding, startline, startcolumn, DEFAULT_BUF_SIZE);
}
/** Constructor. */
public JavaCharStream(final java.io.InputStream dstream,
final String encoding) throws java.io.UnsupportedEncodingException
{
this(dstream, encoding, 1, 1, DEFAULT_BUF_SIZE);
}
/** Reinitialise. */
public void reInit(final java.io.InputStream dstream,
final String encoding) throws java.io.UnsupportedEncodingException
{
reInit(dstream, encoding, 1, 1, DEFAULT_BUF_SIZE);
}
/** Reinitialise. */
public void reInit(final java.io.InputStream dstream,
final String encoding,
final int startline,
final int startcolumn) throws java.io.UnsupportedEncodingException
{
reInit(dstream, encoding, startline, startcolumn, DEFAULT_BUF_SIZE);
}
/** Reinitialise. */
public void reInit(final java.io.InputStream dstream,
final String encoding,
final int startline,
final int startcolumn,
final int buffersize) throws java.io.UnsupportedEncodingException
{
reInit(new java.io.InputStreamReader(dstream, encoding), startline, startcolumn, buffersize);
}
@Override
public void done ()
{
m_aNextCharBuf = null;
super.done ();
}
}
/* ParserGeneratorCC - OriginalChecksum=dd300c75ab21e48f29f86c7cae0e4d4e (do not edit this line) */
© 2015 - 2025 Weber Informatics LLC | Privacy Policy