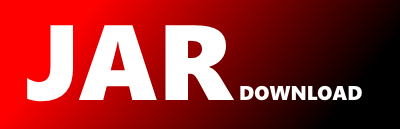
com.xdev.jadoth.sqlengine.interfaces.SqlExecutor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xapi Show documentation
Show all versions of xapi Show documentation
XDEV Application Framework
package com.xdev.jadoth.sqlengine.interfaces;
/*-
* #%L
* XDEV Application Framework
* %%
* Copyright (C) 2003 - 2020 XDEV Software
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import com.xdev.jadoth.sqlengine.internal.DatabaseGateway;
public interface SqlExecutor
{
public R execute(DatabaseGateway> dbc, Statement statement, String sqlStatment) throws SQLException;
public static final SqlExecutor update = new SqlExecutor() {
@Override
public Integer execute(DatabaseGateway> dbc, Statement statement, String sqlStatment) throws SQLException
{
if(statement != null) {
return statement.executeUpdate(sqlStatment);
}
try(Statement createdStatement = dbc.getConnection().createStatement()) {
return createdStatement.executeUpdate(sqlStatment);
}
}
};
public static final SqlExecutor query = new SqlExecutor() {
@Override
public ResultSet execute(DatabaseGateway> dbc, Statement statement, String sqlStatment) throws SQLException
{
if(statement == null){
statement = dbc.getConnection().createStatement();
}
return statement.executeQuery(sqlStatment);
}
};
public static final SqlExecutor
© 2015 - 2025 Weber Informatics LLC | Privacy Policy