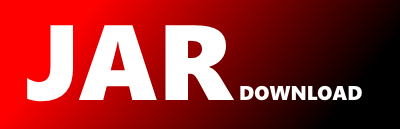
com.xdev.jadoth.sqlengine.internal.AssignmentList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xapi Show documentation
Show all versions of xapi Show documentation
XDEV Application Framework
/**
*
*/
package com.xdev.jadoth.sqlengine.internal;
/*-
* #%L
* XDEV Application Framework
* %%
* Copyright (C) 2003 - 2020 XDEV Software
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.ListIterator;
import com.xdev.jadoth.collections.GrowList;
import com.xdev.jadoth.lang.Copyable;
import com.xdev.jadoth.sqlengine.dbms.DbmsDMLAssembler;
/**
* @author Thomas Muenz
*
*/
public final class AssignmentList implements List, Copyable
{
///////////////////////////////////////////////////////////////////////////
// instance fields //
////////////////////
private final GrowList assignments;
private final Values valueIterable;
private final Columns columnIterable;
//caching fields, not relevant for anything special (serialisation, copiing)
private transient SqlColumn[] cachedColumnArray = null;
private transient Object[] cachedValueArray = null;
///////////////////////////////////////////////////////////////////////////
// constructors //
/////////////////
public AssignmentList()
{
super();
this.assignments = new GrowList(100);
this.valueIterable = new Values();
this.columnIterable = new Columns();
}
protected AssignmentList(final AssignmentList copySource)
{
super();
this.assignments = new GrowList(copySource.assignments);
//these two don't have to be copied as they are just stateless iterator providers
this.valueIterable = new Values();
this.columnIterable = new Columns();
}
@Override
public int size()
{
return this.assignments.size();
}
public void addColumn(final SqlColumn column)
{
if(column == null) {
throw new NullPointerException("column is null");
}
this.assign(column, null);
}
public void assign(final ColumnValueTuple columnValueAssignment)
{
this.clearCacheArray();
this.assignments.add(new Assignment(columnValueAssignment.getColumn(), columnValueAssignment.getValue()));
}
public void assign(final SqlColumn column, final Object value)
{
this.assign(new Assignment(column, value));
}
private final class ColumnIterator implements Iterator
{
private int currentIndex = 0;
/**
* @return
* @see java.util.Iterator#hasNext()
*/
@Override
public boolean hasNext()
{
return this.currentIndex < AssignmentList.this.assignments.size();
}
/**
* @return
* @see java.util.Iterator#next()
*/
@Override
public SqlColumn next()
{
return AssignmentList.this.assignments.get(this.currentIndex++).getColumn();
}
/**
* Not supported.
*
* @see java.util.Iterator#remove()
*/
@Override
public void remove()
{
//empty
}
}
private final class Columns implements Iterable
{
/**
* @return
* @see java.lang.Iterable#iterator()
*/
@Override
public Iterator iterator()
{
return new ColumnIterator();
}
}
private final class Values implements Iterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy