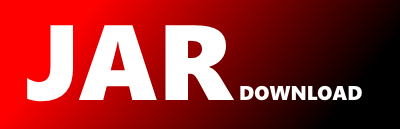
xdev.ui.XdevDialog Maven / Gradle / Ivy
Show all versions of xapi Show documentation
package xdev.ui;
/*-
* #%L
* XDEV Application Framework
* %%
* Copyright (C) 2003 - 2020 XDEV Software
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.awt.Component;
import java.awt.Dialog;
import java.awt.Frame;
import java.awt.HeadlessException;
import java.awt.Window;
import javax.swing.JDialog;
import javax.swing.SwingUtilities;
/**
* The {@link XdevDialog} is a top-level window with a title and a border in
* XDEV. Based on {@link JDialog}.
*
*
* @see JDialog
* @see XdevRootPaneContainer
*
* @author XDEV Software
*
* @since 2.0
*/
public class XdevDialog extends JDialog implements XdevRootPaneContainer
{
private Component owner;
private XdevWindow window;
/**
* Creates a new {@link XdevDialog} with the specified title, owner
* Window
and modality.
*
* This constructor sets the component's locale property to the value
* returned by JComponent.getDefaultLocale
.
*
* @param owner
* Component to get Window ancestor of
*
* @param modal
* specifies whether dialog blocks user input to other top-level
* windows when shown. If true
, the modality type
* property is set to APPLICATION_MODAL
otherwise
* the dialog is MODELESS
*
* @param window
* the {@link XdevWindow} for this {@link XdevDialog}
*
* @throws java.awt.HeadlessException
* when GraphicsEnvironment.isHeadless()
returns
* true
*
* @see JDialog
*/
public XdevDialog(Component owner, boolean modal, XdevWindow window)
throws java.awt.HeadlessException
{
this(getWindowFor(owner),modal,window);
}
static Window getWindowFor(Component owner)
{
if(owner instanceof Window)
{
return (Window)owner;
}
if(owner != null)
{
Window w = SwingUtilities.getWindowAncestor(owner);
if(w != null)
{
return w;
}
}
return UIUtils.getActiveWindow();
}
/**
* Creates a new {@link XdevDialog} with the specified title, owner
* Window
and modality.
*
* This constructor sets the component's locale property to the value
* returned by JComponent.getDefaultLocale
.
*
* @param owner
* the Window
from which the dialog is displayed or
* null
if this dialog has no owner
*
* @param modal
* specifies whether dialog blocks user input to other top-level
* windows when shown. If true
, the modality type
* property is set to APPLICATION_MODAL
otherwise
* the dialog is MODELESS
*
* @param window
* the {@link XdevWindow} for this {@link XdevDialog}
*
* @throws java.awt.HeadlessException
* when GraphicsEnvironment.isHeadless()
returns
* true
*
* @see JDialog
*/
public XdevDialog(Window owner, boolean modal, XdevWindow window)
throws java.awt.HeadlessException
{
super(owner,window.getTitle(),modal ? ModalityType.APPLICATION_MODAL
: ModalityType.MODELESS);
init(owner);
setXdevWindow(window);
}
/**
* Creates a new {@link XdevDialog} with the specified title, owner
* Frame
and modality. If owner
is
* null
, a shared, hidden frame will be set as the owner of
* this dialog.
*
* This constructor sets the component's locale property to the value
* returned by JComponent.getDefaultLocale
.
*
* NOTE: Any popup components (JComboBox
,
* JPopupMenu
, JMenuBar
) created within a modal
* dialog will be forced to be lightweight.
*
* NOTE: This constructor does not allow you to create an unowned
* XdevDialog
.
*
* @param owner
* the Frame
from which the dialog is displayed
*
* @param title
* the String
to display in the dialog's title bar
*
* @param modal
* specifies whether dialog blocks user input to other top-level
* windows when shown. If true
, the modality type
* property is set to DEFAULT_MODALITY_TYPE
* otherwise the dialog is modeless
*
* @throws HeadlessException
* if GraphicsEnvironment.isHeadless()
returns
* true
.
*
* @see JDialog
*/
public XdevDialog(Frame owner, String title, boolean modal) throws HeadlessException
{
super(owner,title,modal);
init(owner);
}
/**
* Creates a new {@link XdevDialog} with the specified title, modality and
* the specified owner Dialog
.
*
* @param owner
* the owner Dialog
from which the dialog is
* displayed or null
if this dialog has no owner
*
* @param title
* the String
to display in the dialog's title bar
*
* @param modal
* specifies whether dialog blocks user input to other top-level
* windows when shown. If true
, the modality type
* property is set to DEFAULT_MODALITY_TYPE
,
* otherwise the dialog is modeless
*
* @throws HeadlessException
* if GraphicsEnvironment.isHeadless()
returns
* true
.
* @see JDialog
*/
public XdevDialog(Dialog owner, String title, boolean modal) throws HeadlessException
{
super(owner,title,modal);
init(owner);
}
private void init(Component owner)
{
this.owner = owner;
setDefaultCloseOperation(DO_NOTHING_ON_CLOSE);
setFocusTraversalPolicy(new XdevFocusTraversalPolicy(this));
}
/**
* {@inheritDoc}
*/
@Override
public final void setXdevWindow(XdevWindow window)
{
cleanup();
this.window = window;
window.setOwner(this);
setTitle(window.getTitle());
setBounds(window.getX(),window.getY(),window.getWidth(),window.getHeight());
setResizable(window.isResizable());
setIconImages(Util.createImageList(window));
setJMenuBar(window.getJMenuBar());
getRootPane().setDefaultButton(window.getDefaultButton());
setContentPane(Util.createContainer(this,window));
if(window.isMinimumSizeSet())
{
setMinimumSize(window.getMinimumSize());
}
}
/**
* {@inheritDoc}
*/
@Override
public XdevWindow getXdevWindow()
{
return window;
}
/**
* {@inheritDoc}
*/
@Override
public Window getWindow()
{
return this;
}
/**
* {@inheritDoc}
*/
@Override
public void setVisible(boolean b)
{
// if(b)
// {
// XdevRootPaneContainer.Util.containers.put(id,this);
// }
super.setVisible(b);
if(!b)
{
SwingUtilities.invokeLater(new Runnable()
{
public void run()
{
cleanup();
// XdevRootPaneContainer.Util.containers.remove(id);
}
});
if(owner instanceof Window)
{
((Window)owner).toFront();
}
}
}
private void cleanup()
{
if(window != null)
{
window.setOwner(null);
window = null;
}
}
/**
* Implemented with no action for {@link XdevApplicationContainer}.
*/
@Override
public void setExtendedState(int extendedState)
{
// no op
}
/**
* Implemented with no action for {@link XdevApplicationContainer}.
*/
@Override
public int getExtendedState()
{
return NORMAL;
}
/**
* {@inheritDoc}
*/
@Override
public void close()
{
setVisible(false);
dispose();
}
}