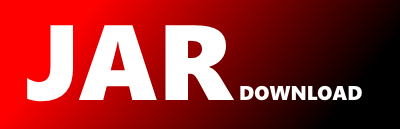
xdev.ui.XdevSplitPane Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xapi Show documentation
Show all versions of xapi Show documentation
XDEV Application Framework
package xdev.ui;
/*-
* #%L
* XDEV Application Framework
* %%
* Copyright (C) 2003 - 2020 XDEV Software
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.awt.Component;
import javax.swing.JSplitPane;
import javax.swing.UIManager;
import xdev.ui.persistence.Persistable;
/**
* The standard split pane in XDEV. Based on {@link JSplitPane}.
*
* @see JSplitPane
*
* @author XDEV Software
*
* @since 2.0
*/
@BeanSettings(acceptChildren = true, useXdevCustomizer = true)
public class XdevSplitPane extends JSplitPane implements Persistable
{
/**
*
*/
private static final long serialVersionUID = 491725401915117145L;
/**
* Should the gui state be persisted? Defaults to {@code true}.
*/
private boolean persistenceEnabled = true;
/**
* Creates a new {@link XdevSplitPane} with the default orientation
* {@link JSplitPane#HORIZONTAL_SPLIT}.
*/
public XdevSplitPane()
{
this(HORIZONTAL_SPLIT);
}
/**
* Creates a new {@link XdevSplitPane} with the specified orientation.
*
* @param orientation
* JSplitPane.HORIZONTAL_SPLIT
or
* JSplitPane.VERTICAL_SPLIT
*
* @throws IllegalArgumentException
* if orientation
is not one of HORIZONTAL_SPLIT or
* VERTICAL_SPLIT
*/
public XdevSplitPane(int orientation) throws IllegalArgumentException
{
this(orientation,UIManager.getBoolean("SplitPane.continuousLayout"));
}
/**
* Creates a new {@link XdevSplitPane} with the specified orientation and
* redrawing style.
*
* @param newOrientation
* JSplitPane.HORIZONTAL_SPLIT
or
* JSplitPane.VERTICAL_SPLIT
*
* @param newContinuousLayout
* a boolean, true
for the components to redraw
* continuously as the divider changes position,
* false
to wait until the divider position stops
* changing to redraw
*
* @throws IllegalArgumentException
* if orientation
is not one of HORIZONTAL_SPLIT or
* VERTICAL_SPLIT
*/
public XdevSplitPane(int newOrientation, boolean newContinuousLayout)
throws IllegalArgumentException
{
this(newOrientation,newContinuousLayout,null,null);
}
/**
* Creates a new {@link XdevSplitPane} with the specified orientation and
* components.
*
* @param newOrientation
* JSplitPane.HORIZONTAL_SPLIT
or
* JSplitPane.VERTICAL_SPLIT
*
* @param newLeftComponent
* the Component
that will appear on the left of a
* horizontally-split pane, or at the top of a vertically-split
* pane
*
* @param newRightComponent
* the Component
that will appear on the right of a
* horizontally-split pane, or at the bottom of a
* vertically-split pane
*
* @throws IllegalArgumentException
* if orientation
is not one of HORIZONTAL_SPLIT or
* VERTICAL_SPLIT
*/
public XdevSplitPane(int newOrientation, Component newLeftComponent, Component newRightComponent)
throws IllegalArgumentException
{
this(newOrientation,UIManager.getBoolean("SplitPane.continuousLayout"),newLeftComponent,
newRightComponent);
}
/**
* Creates a new {@link XdevSplitPane} with the specified orientation and
* redrawing style, and with the specified components.
*
* @param newOrientation
* JSplitPane.HORIZONTAL_SPLIT
or
* JSplitPane.VERTICAL_SPLIT
*
* @param newContinuousLayout
* a boolean, true
for the components to redraw
* continuously as the divider changes position,
* false
to wait until the divider position stops
* changing to redraw
*
* @param newLeftComponent
* the Component
that will appear on the left of a
* horizontally-split pane, or at the top of a vertically-split
* pane
*
* @param newRightComponent
* the Component
that will appear on the right of a
* horizontally-split pane, or at the bottom of a
* vertically-split pane
*
* @throws IllegalArgumentException
* if orientation
is not one of HORIZONTAL_SPLIT or
* VERTICAL_SPLIT
*/
public XdevSplitPane(int newOrientation, boolean newContinuousLayout,
Component newLeftComponent, Component newRightComponent)
throws IllegalArgumentException
{
super(newOrientation,newContinuousLayout,newLeftComponent,newRightComponent);
}
/**
* {@inheritDoc}
*/
@Override
public String toString()
{
String toString = UIUtils.toString(this);
if(toString != null)
{
return toString;
}
return super.toString();
}
/**
* {@inheritDoc}
*
* @see #savePersistentState()
*/
@Override
public void loadPersistentState(String persistentState)
{
this.setDividerLocation(Integer.parseInt(persistentState));
}
/**
* {@inheritDoc}
*
* Persisted properties:
*
* - divider location
*
*
*/
@Override
public String savePersistentState()
{
return Integer.toString(this.getDividerLocation());
}
/**
* Uses the name of the component as a persistent id.
*
* If no name is specified the name of the class will be used. This will
* work only for one persistent instance of the class!
*
{@inheritDoc}
*/
@Override
public String getPersistentId()
{
return (this.getName() != null) ? this.getName() : this.getClass().getSimpleName();
}
/**
* {@inheritDoc}
*/
@Override
public boolean isPersistenceEnabled()
{
return persistenceEnabled;
}
/**
* Sets the persistenceEnabled flag.
*
* @param persistenceEnabled
* the state for this instance
*/
public void setPersistenceEnabled(boolean persistenceEnabled)
{
this.persistenceEnabled = persistenceEnabled;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy