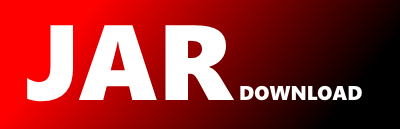
xdev.util.ConversionUtils Maven / Gradle / Ivy
Show all versions of xapi Show documentation
package xdev.util;
/*-
* #%L
* XDEV Application Framework
* %%
* Copyright (C) 2003 - 2020 XDEV Software
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.awt.Image;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.Reader;
import java.lang.reflect.Array;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URL;
import java.sql.Blob;
import java.sql.Clob;
import java.sql.SQLException;
import java.text.DateFormat;
import java.text.DecimalFormat;
import java.text.DecimalFormatSymbols;
import java.text.NumberFormat;
import java.text.ParseException;
import java.text.ParsePosition;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Collection;
import java.util.Date;
import java.util.Hashtable;
import java.util.Map;
import javax.swing.Icon;
import xdev.db.DBException;
import xdev.io.ByteHolder;
import xdev.io.CharHolder;
import xdev.io.IOUtils;
import xdev.io.XdevFile;
import xdev.lang.LibraryMember;
import xdev.lang.NotNull;
import xdev.ui.GraphicUtils;
import xdev.ui.XdevImage;
import xdev.vt.XdevBlob;
import xdev.vt.XdevClob;
/**
* A utility class which converts Object's into specific types.
*
* This is done via registered {@link ObjectConverter}'s. See
* {@link #setObjectConverter(Class, ObjectConverter)},
* {@link #getObjectConverter(Class)}.
*
* The {@link #convert(Object, Class)} method forwards the request to the
* registered {@link ObjectConverter} and returns the converted value, If the
* conversion fails a {@link ObjectConversionException} is thrown.
*
* Alternatively you can use {@link #convert(Object, Class, Object)} wich uses a
* fallback instead of the {@link ObjectConversionException}.
*
* Default converters are installed for the following types:
*
* - byte resp. Byte
* - short resp. Short
* - int resp. Integer
* - long resp. Long
* - float resp. Float
* - double resp. Double
* - boolean resp. Boolean
* - char resp. Character
* - String
* - Date
* - XdevDate
* - XdevBlob
* - byte[]
* - XdevClob
* - char[]
* - XdevList
* - XdevHashtable
* - XdevFile
* - XdevImage
*
* There are convenience methods for the preinstalled converters too, like:
* {@link #toint(Object)} or {@link #toInteger(Object)}.
*
* @author XDEV Software
* @since 3.1
*/
@LibraryMember
public final class ConversionUtils
{
private ConversionUtils()
{
}
private static Map objectConverter = new Hashtable();
static
{
setObjectConverter(Byte.class,new ByteConverter());
setObjectConverter(Short.class,new ShortConverter());
setObjectConverter(Integer.class,new IntegerConverter());
setObjectConverter(Long.class,new LongConverter());
setObjectConverter(Float.class,new FloatConverter());
setObjectConverter(Double.class,new DoubleConverter());
setObjectConverter(Boolean.class,new BooleanConverter());
setObjectConverter(Character.class,new CharacterConverter());
setObjectConverter(String.class,new StringConverter());
setObjectConverter(Date.class,new DateConverter());
setObjectConverter(XdevDate.class,new XdevDateConverter());
setObjectConverter(XdevBlob.class,new XdevBlobConverter());
setObjectConverter(byte[].class,new BytesConverter());
setObjectConverter(XdevClob.class,new XdevClobConverter());
setObjectConverter(char[].class,new CharsConverter());
setObjectConverter(XdevList.class,new XdevListConverter());
setObjectConverter(XdevHashtable.class,new XdevHashtableConverter());
setObjectConverter(XdevFile.class,new XdevFileConverter());
setObjectConverter(XdevImage.class,new XdevImageConverter());
}
/**
* Registeres an ObjectConverter for the type T.
*
* @param
* @param type
* the registered type
* @param converter
* the new converter
*/
public static void setObjectConverter(Class type, ObjectConverter converter)
{
objectConverter.put(type,converter);
}
/**
* Returns the registered ObjectConverter for type
, or
* null
if none is registered.
*
* @param
* @param type
* the conversion type
* @return the registered ObjectConverter or null
*/
public static ObjectConverter getObjectConverter(Class type)
{
return objectConverter.get(type);
}
/**
* Converts value
to type
via the registered
* {@link ObjectConverter}.
*
* If no converter is registered for type
or the conversion
* fails an {@link ObjectConversionException} is thrown.
*
* @param
* @param value
* the value to convert
* @param type
* the target type
* @return the converted value
* @throws ObjectConversionException
* if no converter is registered for type
or the
* conversion fails
*/
public static T convert(Object value, Class type) throws ObjectConversionException
{
ObjectConverter converter = getObjectConverter(type);
if(converter == null)
{
throw new ObjectConversionException("No converter found for type " + type.getName());
}
return converter.convert(value);
}
/**
* Converts value
to type
via the registered
* {@link ObjectConverter}.
*
* If no converter is registered for type
or the conversion
* fails fallback
is returned.
*
* @param
* @param value
* the value to convert
* @param type
* the target type
* @param fallback
* the fallback value
* @return the converted value or fallback
*/
public static T convert(Object value, Class type, T fallback)
{
try
{
return convert(value,type);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
// *******************************************************************************
// Convenience Methods
// *******************************************************************************
/**
* Converts value
to a byte primitive.
*
* If value is null
or the conversion fails an
* {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a byte primitive
* @throws ObjectConversionException
* If value is null
or the conversion fails
*/
public static byte tobyte(@NotNull Object value) throws ObjectConversionException
{
if(value == null)
{
throw new ObjectConversionException(new NullPointerException());
}
return toByte(value).byteValue();
}
/**
* Converts value
to a byte primitive.
*
* If value is null
or the conversion fails
* fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a byte primitive or fallback
*/
public static byte tobyte(Object value, byte fallback)
{
try
{
return tobyte(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a {@link Byte}.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a Byte, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static Byte toByte(Object value) throws ObjectConversionException
{
return convert(value,Byte.class);
}
/**
* Converts value
to a {@link Byte}.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a Byte, or fallback
if the conversion fails
*/
public static Byte toByte(Object value, Byte fallback)
{
try
{
return toByte(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a short primitive.
*
* If value is null
or the conversion fails an
* {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a short primitive
* @throws ObjectConversionException
* If value is null
or the conversion fails
*/
public static short toshort(@NotNull Object value) throws ObjectConversionException
{
if(value == null)
{
throw new ObjectConversionException(new NullPointerException());
}
return toShort(value).shortValue();
}
/**
* Converts value
to a short primitive.
*
* If value is null
or the conversion fails
* fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a short primitive or fallback
*/
public static short toshort(Object value, short fallback)
{
try
{
return toshort(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a {@link Short}.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a Short, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static Short toShort(Object value) throws ObjectConversionException
{
return convert(value,Short.class);
}
/**
* Converts value
to a {@link Short}.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a Short, or fallback
if the conversion fails
*/
public static Short toShort(Object value, Short fallback)
{
try
{
return toShort(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to an int primitive.
*
* If value is null
or the conversion fails an
* {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return an int primitive
* @throws ObjectConversionException
* If value is null
or the conversion fails
*/
public static int toint(@NotNull Object value) throws ObjectConversionException
{
if(value == null)
{
throw new ObjectConversionException(new NullPointerException());
}
return toInteger(value).intValue();
}
/**
* Converts value
to an int primitive.
*
* If value is null
or the conversion fails
* fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return an int primitive or fallback
*/
public static int toint(Object value, int fallback)
{
try
{
return toint(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to an {@link Integer}.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a Integer, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static Integer toInteger(Object value) throws ObjectConversionException
{
return convert(value,Integer.class);
}
/**
* Converts value
to an {@link Integer}.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return an Integer, or fallback
if the conversion fails
*/
public static Integer toInteger(Object value, Integer fallback)
{
try
{
return toInteger(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a long primitive.
*
* If value is null
or the conversion fails an
* {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a long primitive
* @throws ObjectConversionException
* If value is null
or the conversion fails
*/
public static long tolong(@NotNull Object value) throws ObjectConversionException
{
if(value == null)
{
throw new ObjectConversionException(new NullPointerException());
}
return toLong(value).longValue();
}
/**
* Converts value
to a long primitive.
*
* If value is null
or the conversion fails
* fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a long primitive or fallback
*/
public static long tolong(Object value, long fallback)
{
try
{
return tolong(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a {@link Long}.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a Long, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static Long toLong(Object value) throws ObjectConversionException
{
return convert(value,Long.class);
}
/**
* Converts value
to a {@link Long}.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a Long, or fallback
if the conversion fails
*/
public static Long toLong(Object value, Long fallback)
{
try
{
return toLong(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a float primitive.
*
* If value is null
or the conversion fails an
* {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a float primitive
* @throws ObjectConversionException
* If value is null
or the conversion fails
*/
public static float tofloat(@NotNull Object value) throws ObjectConversionException
{
if(value == null)
{
throw new ObjectConversionException(new NullPointerException());
}
return toFloat(value).floatValue();
}
/**
* Converts value
to a float primitive.
*
* If value is null
or the conversion fails
* fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a float primitive or fallback
*/
public static float tofloat(Object value, float fallback)
{
try
{
return tofloat(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a {@link Float}.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a Float, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static Float toFloat(Object value) throws ObjectConversionException
{
return convert(value,Float.class);
}
/**
* Converts value
to a {@link Float}.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a Float, or fallback
if the conversion fails
*/
public static Float toFloat(Object value, Float fallback)
{
try
{
return toFloat(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a double primitive.
*
* If value is null
or the conversion fails an
* {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a double primitive
* @throws ObjectConversionException
* If value is null
or the conversion fails
*/
public static double todouble(@NotNull Object value) throws ObjectConversionException
{
if(value == null)
{
throw new ObjectConversionException(new NullPointerException());
}
return toDouble(value).doubleValue();
}
/**
* Converts value
to a double primitive.
*
* If value is null
or the conversion fails
* fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a double primitive or fallback
*/
public static double todouble(Object value, double fallback)
{
try
{
return todouble(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a {@link Double}.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a Double, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static Double toDouble(Object value) throws ObjectConversionException
{
return convert(value,Double.class);
}
/**
* Converts value
to a {@link Double}.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a Double, or fallback
if the conversion fails
*/
public static Double toDouble(Object value, Double fallback)
{
try
{
return toDouble(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a boolean primitive.
*
* If value is null
or the conversion fails an
* {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a boolean primitive
* @throws ObjectConversionException
* If value is null
or the conversion fails
*/
public static boolean toboolean(@NotNull Object value) throws ObjectConversionException
{
if(value == null)
{
throw new ObjectConversionException(new NullPointerException());
}
return toBoolean(value).booleanValue();
}
/**
* Converts value
to a boolean primitive.
*
* If value is null
or the conversion fails
* fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a boolean primitive or fallback
*/
public static boolean toboolean(Object value, boolean fallback)
{
try
{
return toboolean(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a {@link Boolean}.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a Boolean, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static Boolean toBoolean(Object value) throws ObjectConversionException
{
return convert(value,Boolean.class);
}
/**
* Converts value
to a {@link Boolean}.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a Boolean, or fallback
if the conversion fails
*/
public static Boolean toBoolean(Object value, Boolean fallback)
{
try
{
return toBoolean(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a char primitive.
*
* If value is null
or the conversion fails an
* {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a char primitive
* @throws ObjectConversionException
* If value is null
or the conversion fails
*/
public static char tochar(@NotNull Object value) throws ObjectConversionException
{
if(value == null)
{
throw new ObjectConversionException(new NullPointerException());
}
return toCharacter(value).charValue();
}
/**
* Converts value
to a char primitive.
*
* If value is null
or the conversion fails
* fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a char primitive or fallback
*/
public static char tochar(Object value, char fallback)
{
try
{
return tochar(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a {@link Character}.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a Character, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static Character toCharacter(Object value) throws ObjectConversionException
{
return convert(value,Character.class);
}
/**
* Converts value
to a {@link Character}.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a Character, or fallback
if the conversion fails
*/
public static Character toCharacter(Object value, Character fallback)
{
try
{
return toCharacter(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a {@link String}.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a String, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static String toString(Object value) throws ObjectConversionException
{
return convert(value,String.class);
}
/**
* Converts value
to a {@link String}.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a String, or fallback
if the conversion fails
*/
public static String toString(Object value, String fallback)
{
try
{
return toString(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a {@link Date}.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a Date, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static Date toDate(Object value) throws ObjectConversionException
{
return convert(value,Date.class);
}
/**
* Converts value
to a {@link Date}.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a Date, or fallback
if the conversion fails
*/
public static Date toDate(Object value, Date fallback)
{
try
{
return toDate(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a {@link XdevDate}.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a XdevDate, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static XdevDate toXdevDate(Object value) throws ObjectConversionException
{
return convert(value,XdevDate.class);
}
/**
* Converts value
to a {@link XdevDate}.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a XdevDate, or fallback
if the conversion fails
*/
public static XdevDate toXdevDate(Object value, XdevDate fallback)
{
try
{
return toXdevDate(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a {@link XdevBlob}.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a XdevBlob, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static XdevBlob toXdevBlob(Object value) throws ObjectConversionException
{
return convert(value,XdevBlob.class);
}
/**
* Converts value
to a {@link XdevBlob}.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a XdevBlob, or fallback
if the conversion fails
*/
public static XdevBlob toXdevBlob(Object value, XdevBlob fallback)
{
try
{
return toXdevBlob(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a byte[] array.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a byte[] array, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static byte[] toBytes(Object value) throws ObjectConversionException
{
return convert(value,byte[].class);
}
/**
* Converts value
to a byte[] array.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a byte[] array, or fallback
if the conversion fails
*/
public static byte[] toBytes(Object value, byte[] fallback)
{
try
{
return toBytes(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a {@link XdevClob}.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a XdevClob, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static XdevClob toXdevClob(Object value) throws ObjectConversionException
{
return convert(value,XdevClob.class);
}
/**
* Converts value
to a {@link XdevClob}.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a XdevClob, or fallback
if the conversion fails
*/
public static XdevClob toXdevClob(Object value, XdevClob fallback)
{
try
{
return toXdevClob(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a char[] array.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a char[] array, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static char[] toChars(Object value) throws ObjectConversionException
{
return convert(value,char[].class);
}
/**
* Converts value
to a char[] array.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a char[] array, or fallback
if the conversion fails
*/
public static char[] toChars(Object value, char[] fallback)
{
try
{
return toChars(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a {@link XdevList}.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a XdevList, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static XdevList toXdevList(Object value) throws ObjectConversionException
{
return convert(value,XdevList.class);
}
/**
* Converts value
to a {@link XdevList}.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a XdevList, or fallback
if the conversion fails
*/
public static XdevList toXdevList(Object value, XdevList fallback)
{
try
{
return toXdevList(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a {@link XdevHashtable}.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a XdevHashtable, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static XdevHashtable toXdevHashtable(Object value) throws ObjectConversionException
{
return convert(value,XdevHashtable.class);
}
/**
* Converts value
to a {@link XdevHashtable}.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a XdevHashtable, or fallback
if the conversion fails
*/
public static XdevHashtable toXdevHashtable(Object value, XdevHashtable fallback)
{
try
{
return toXdevHashtable(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a {@link XdevFile}.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a XdevFile, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static XdevFile toXdevFile(Object value) throws ObjectConversionException
{
return convert(value,XdevFile.class);
}
/**
* Converts value
to a {@link XdevFile}.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a XdevFile, or fallback
if the conversion fails
*/
public static XdevFile toXdevFile(Object value, XdevFile fallback)
{
try
{
return toXdevFile(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
/**
* Converts value
to a {@link XdevImage}.
*
* If the conversion fails an {@link ObjectConversionException} is thrown.
*
* @param value
* the value to convert
* @return a XdevImage, or null
if value
is
* null
* @throws ObjectConversionException
* If the conversion fails
*/
public static XdevImage toXdevImage(Object value) throws ObjectConversionException
{
return convert(value,XdevImage.class);
}
/**
* Converts value
to a {@link XdevImage}.
*
* If the conversion fails fallback
is returned.
*
* @param value
* the value to convert
* @param fallback
* the fallback value
* @return a XdevImage, or fallback
if the conversion fails
*/
public static XdevImage toXdevImage(Object value, XdevImage fallback)
{
try
{
return toXdevImage(value);
}
catch(ObjectConversionException e)
{
return fallback;
}
}
// *******************************************************************************
// Default Implementations
// *******************************************************************************
private final static NumberFormat integerFormat = NumberFormat.getIntegerInstance();
private final static NumberFormat decimalFormat = NumberFormat.getNumberInstance();
/**
* Workaround to avoid {@link NumberFormat}'s parsing issues.
*
* @param input
* the string to parse
* @param formats
* given formats
* @return parsed number or null
if parse failed
*
* @see http://www.ibm.com/developerworks/library/j-numberformat/
* @see https://www.xdevissues.com/browse/XDEVAPI-215
*/
private static Number parseNumber(String input, boolean decimal)
{
char separator = '\0';
loop: for(int i = 0, c = input.length(); i < c; i++)
{
char ch = input.charAt(i);
switch(ch)
{
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
case '8':
case '9':
case '-':
break;
case '.':
case ',':
if(separator == '\0')
{
separator = ch;
}
else
{
separator = '\0';
break loop;
}
break;
default:
separator = '\0';
break loop;
}
}
NumberFormat format;
if(separator != '\0' && decimal)
{
// one separator char
DecimalFormatSymbols symbols = new DecimalFormatSymbols();
symbols.setDecimalSeparator(separator);
DecimalFormat decimalFormat = new DecimalFormat("#.#;-#.#",symbols);
decimalFormat.setGroupingUsed(false);
format = decimalFormat;
}
else if(decimal)
{
format = decimalFormat;
}
else
{
format = integerFormat;
}
ParsePosition pos = new ParsePosition(0);
Number result = format.parse(input,pos);
if(result != null && input.length() == pos.getIndex())
{
return result;
}
return null;
}
private static class ByteConverter implements ObjectConverter
{
@Override
public Byte convert(Object value) throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value instanceof Byte)
{
return (Byte)value;
}
if(value instanceof Number)
{
return ((Number)value).byteValue();
}
Number number = parseNumber(value.toString(),false);
if(number != null)
{
long longVal = number.longValue();
if(longVal < Byte.MIN_VALUE || longVal > Byte.MAX_VALUE)
{
throw new ObjectConversionException("Value out of range: " + longVal);
}
return number.byteValue();
}
throw new ObjectConversionException(value,Byte.class);
}
}
private static class ShortConverter implements ObjectConverter
{
@Override
public Short convert(Object value) throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value instanceof Short)
{
return (Short)value;
}
if(value instanceof Number)
{
return ((Number)value).shortValue();
}
Number number = parseNumber(value.toString(),false);
if(number != null)
{
long longVal = number.longValue();
if(longVal < Short.MIN_VALUE || longVal > Short.MAX_VALUE)
{
throw new ObjectConversionException("Value out of range: " + longVal);
}
return number.shortValue();
}
throw new ObjectConversionException(value,Short.class);
}
}
private static class IntegerConverter implements ObjectConverter
{
@Override
public Integer convert(Object value) throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value instanceof Integer)
{
return (Integer)value;
}
if(value instanceof Number)
{
return ((Number)value).intValue();
}
Number number = parseNumber(value.toString(),false);
if(number != null)
{
long longVal = number.longValue();
if(longVal < Integer.MIN_VALUE || longVal > Integer.MAX_VALUE)
{
throw new ObjectConversionException("Value out of range: " + longVal);
}
return number.intValue();
}
throw new ObjectConversionException(value,Integer.class);
}
}
private static class LongConverter implements ObjectConverter
{
@Override
public Long convert(Object value) throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value instanceof Long)
{
return (Long)value;
}
if(value instanceof Number)
{
return ((Number)value).longValue();
}
if(value instanceof Date)
{
return ((Date)value).getTime();
}
Number number = parseNumber(value.toString(),false);
if(number != null)
{
return number.longValue();
}
throw new ObjectConversionException(value,Long.class);
}
}
private static class FloatConverter implements ObjectConverter
{
@Override
public Float convert(Object value) throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value instanceof Float)
{
return (Float)value;
}
if(value instanceof Number)
{
return ((Number)value).floatValue();
}
String string = value.toString();
Number number = parseNumber(string,true);
if(number != null)
{
float floatVal = number.floatValue();
if(floatVal == Float.NaN || floatVal == Float.POSITIVE_INFINITY
|| floatVal == Float.NEGATIVE_INFINITY)
{
throw new ObjectConversionException("Value out of range: " + string);
}
return floatVal;
}
throw new ObjectConversionException(value,Float.class);
}
}
private static class DoubleConverter implements ObjectConverter
{
@Override
public Double convert(Object value) throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value instanceof Double)
{
return (Double)value;
}
if(value instanceof Number)
{
return ((Number)value).doubleValue();
}
if(value instanceof Date)
{
return (double)((Date)value).getTime();
}
String string = value.toString();
Number number = parseNumber(string,true);
if(number != null)
{
double doubleVal = number.doubleValue();
if(doubleVal == Double.NaN || doubleVal == Double.POSITIVE_INFINITY
|| doubleVal == Double.NEGATIVE_INFINITY)
{
throw new ObjectConversionException("Value out of range: " + string);
}
return doubleVal;
}
throw new ObjectConversionException(value,Double.class);
}
}
private static class BooleanConverter implements ObjectConverter
{
@Override
public Boolean convert(Object value) throws ObjectConversionException
{
return toBoolean(value,true);
}
}
/**
* Used by number converters with tryNumberConversion=false to avoid stack
* overflow
*/
private static Boolean toBoolean(Object value, boolean tryNumberConversion)
throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value instanceof Boolean)
{
return (Boolean)value;
}
else
{
String s = value.toString();
if("TRUE".equalsIgnoreCase(s))
{
return Boolean.TRUE;
}
if("FALSE".equalsIgnoreCase(s))
{
return Boolean.FALSE;
}
if(tryNumberConversion)
{
try
{
long number = tolong(value);
return number != 0;
}
catch(ObjectConversionException e)
{
}
}
}
throw new ObjectConversionException(value,Boolean.class);
}
private static class CharacterConverter implements ObjectConverter
{
@Override
public Character convert(Object value) throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value instanceof Character)
{
return (Character)value;
}
if(value instanceof String)
{
String str = (String)value;
if(str.length() == 1)
{
return str.charAt(0);
}
}
else
{
try
{
int number = ConversionUtils.toint(value);
return (char)number;
}
catch(ObjectConversionException e)
{
}
}
throw new ObjectConversionException(value,Character.class);
}
}
private static class StringConverter implements ObjectConverter
{
@Override
public String convert(Object value) throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value.getClass() == String.class)
{
return (String)value;
}
if(value instanceof char[])
{
return new String((char[])value);
}
if(value instanceof byte[])
{
return new String((byte[])value);
}
if(value instanceof Reader)
{
try
{
return IOUtils.readString((Reader)value,true);
}
catch(IOException e)
{
throw new ObjectConversionException(e);
}
}
if(value instanceof InputStream)
{
try
{
return IOUtils.readString((InputStream)value,true);
}
catch(IOException e)
{
throw new ObjectConversionException(e);
}
}
if(value instanceof CharHolder)
{
try
{
return new String(((CharHolder)value).toCharArray());
}
catch(Exception e)
{
throw new ObjectConversionException(e);
}
}
if(value instanceof ByteHolder)
{
try
{
return new String(((ByteHolder)value).toByteArray());
}
catch(Exception e)
{
throw new ObjectConversionException(e);
}
}
if(value instanceof Clob)
{
try
{
return IOUtils.readString(((Clob)value).getCharacterStream(),true);
}
catch(Exception e)
{
throw new ObjectConversionException(e);
}
}
if(value instanceof Blob)
{
try
{
return IOUtils.readString(((Blob)value).getBinaryStream(),true);
}
catch(Exception e)
{
throw new ObjectConversionException(e);
}
}
return value.toString();
}
}
private static class DateConverter implements ObjectConverter
{
final SimpleDateFormat SIMPLE_DATETIME_FORMAT;
final SimpleDateFormat SIMPLE_DATE_FORMAT;
final SimpleDateFormat SIMPLE_TIME_FORMAT;
final int[] DATE_STYLE_CHECK_ORDER;
{
SIMPLE_DATETIME_FORMAT = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
SIMPLE_DATE_FORMAT = new SimpleDateFormat("yyyy-MM-dd");
SIMPLE_TIME_FORMAT = new SimpleDateFormat("HH:mm:ss");
DATE_STYLE_CHECK_ORDER = new int[]{DateFormat.MEDIUM,DateFormat.SHORT,DateFormat.LONG,
DateFormat.FULL};
}
@Override
public Date convert(Object value) throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value instanceof Date)
{
return (Date)value;
}
if(value instanceof Calendar)
{
return ((Calendar)value).getTime();
}
if(value instanceof Number)
{
return new Date(((Number)value).longValue());
}
String s = value.toString();
try
{
return SIMPLE_DATETIME_FORMAT.parse(s);
}
catch(ParseException e)
{
}
try
{
return SIMPLE_DATE_FORMAT.parse(s);
}
catch(ParseException e)
{
}
try
{
return SIMPLE_TIME_FORMAT.parse(s);
}
catch(ParseException e)
{
}
for(int date : DATE_STYLE_CHECK_ORDER)
{
for(int time : DATE_STYLE_CHECK_ORDER)
{
try
{
return DateFormat.getDateTimeInstance(date,time).parse(s);
}
catch(ParseException e)
{
}
}
}
throw new ObjectConversionException(value,XdevDate.class);
}
}
private static class XdevDateConverter implements ObjectConverter
{
@Override
public XdevDate convert(Object value) throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value instanceof XdevDate)
{
return (XdevDate)value;
}
if(value instanceof Date)
{
return new XdevDate((Date)value);
}
if(value instanceof Calendar)
{
return new XdevDate((Calendar)value);
}
if(value instanceof Number)
{
return new XdevDate(new Date(((Number)value).longValue()));
}
try
{
Date date = ConversionUtils.toDate(value);
return new XdevDate(date);
}
catch(ObjectConversionException e)
{
}
throw new ObjectConversionException(value,XdevDate.class);
}
}
private static class XdevBlobConverter implements ObjectConverter
{
@Override
public XdevBlob convert(Object value) throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value instanceof XdevBlob)
{
return (XdevBlob)value;
}
if(value instanceof Blob)
{
try
{
return new XdevBlob((Blob)value);
}
catch(DBException e)
{
throw new ObjectConversionException(e);
}
}
if(value instanceof byte[])
{
return new XdevBlob((byte[])value);
}
if(value instanceof ByteHolder)
{
return new XdevBlob(((ByteHolder)value).toByteArray());
}
if(value instanceof InputStream)
{
try
{
InputStream in = (InputStream)value;
try
{
return new XdevBlob(IOUtils.readData(in));
}
finally
{
IOUtils.closeSilent(in);
}
}
catch(IOException e)
{
throw new ObjectConversionException(e);
}
}
throw new ObjectConversionException(value,XdevBlob.class);
}
}
private static class BytesConverter implements ObjectConverter
{
@Override
public byte[] convert(Object value) throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value instanceof byte[])
{
return (byte[])value;
}
else if(value instanceof Blob)
{
try
{
Blob blob = (Blob)value;
return blob.getBytes(1,(int)blob.length());
}
catch(SQLException e)
{
throw new ObjectConversionException(e);
}
}
else if(value instanceof InputStream)
{
try
{
InputStream in = (InputStream)value;
try
{
return IOUtils.readData(in);
}
finally
{
IOUtils.closeSilent(in);
}
}
catch(IOException e)
{
throw new ObjectConversionException(e);
}
}
else if(value instanceof ByteHolder)
{
return ((ByteHolder)value).toByteArray();
}
throw new ObjectConversionException(value,byte[].class);
}
}
private static class XdevClobConverter implements ObjectConverter
{
@Override
public XdevClob convert(Object value) throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value instanceof XdevClob)
{
return (XdevClob)value;
}
if(value instanceof Clob)
{
try
{
return new XdevClob((Clob)value);
}
catch(DBException e)
{
throw new ObjectConversionException(e);
}
}
if(value instanceof char[])
{
return new XdevClob((char[])value);
}
if(value instanceof CharHolder)
{
return new XdevClob(((CharHolder)value).toCharArray());
}
if(value instanceof String)
{
return new XdevClob(((String)value).toCharArray());
}
if(value instanceof Reader)
{
try
{
return new XdevClob(IOUtils.readChars((Reader)value,true));
}
catch(IOException e)
{
throw new ObjectConversionException(e);
}
}
if(value instanceof InputStream)
{
try
{
return new XdevClob(IOUtils.readString((InputStream)value,true).toCharArray());
}
catch(IOException e)
{
throw new ObjectConversionException(e);
}
}
throw new ObjectConversionException(value,XdevClob.class);
}
}
private static class CharsConverter implements ObjectConverter
{
@Override
public char[] convert(Object value) throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value instanceof char[])
{
return (char[])value;
}
try
{
String string = ConversionUtils.toString(value);
return string.toCharArray();
}
catch(ObjectConversionException e)
{
}
throw new ObjectConversionException(value,char[].class);
}
}
private static class XdevListConverter implements ObjectConverter
{
@Override
public XdevList convert(Object value) throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value instanceof XdevList)
{
return (XdevList)value;
}
if(value instanceof Collection)
{
return new XdevList((Collection)value);
}
if(value.getClass().isArray())
{
return new XdevList((Object[])value);
}
throw new ObjectConversionException(value,XdevList.class);
}
}
private static class XdevHashtableConverter implements ObjectConverter
{
@Override
public XdevHashtable convert(Object value) throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value instanceof XdevHashtable)
{
return (XdevHashtable)value;
}
if(value instanceof Map)
{
return new XdevHashtable((Map)value);
}
if(value.getClass().isArray() && Array.getLength(value) % 2 == 0)
{
return new XdevHashtable((Object[])value);
}
throw new ObjectConversionException(value,XdevHashtable.class);
}
}
private static class XdevFileConverter implements ObjectConverter
{
@Override
public XdevFile convert(Object value) throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value instanceof XdevFile)
{
return (XdevFile)value;
}
if(value instanceof File)
{
return new XdevFile((File)value);
}
if(value instanceof URI)
{
try
{
return new XdevFile((URI)value);
}
catch(IllegalArgumentException e)
{
}
}
if(value instanceof URL)
{
try
{
return new XdevFile(((URL)value).toURI());
}
catch(URISyntaxException e)
{
}
catch(IllegalArgumentException e)
{
}
}
throw new ObjectConversionException(value,XdevFile.class);
}
}
private static class XdevImageConverter implements ObjectConverter
{
@Override
public XdevImage convert(Object value) throws ObjectConversionException
{
if(value == null)
{
return null;
}
if(value instanceof XdevImage)
{
return (XdevImage)value;
}
if(value instanceof Image)
{
return new XdevImage((Image)value);
}
else if(value instanceof Icon)
{
return new XdevImage(GraphicUtils.createImageFromIcon((Icon)value));
}
throw new ObjectConversionException(value,XdevImage.class);
}
}
}