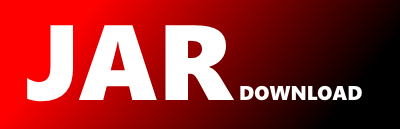
de.charite.compbio.jannovar.data.Chromosome Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jannovar-core Show documentation
Show all versions of jannovar-core Show documentation
jannovar-core is a library for the functional annotation of genomic variants
package de.charite.compbio.jannovar.data;
import java.io.Serializable;
import de.charite.compbio.jannovar.impl.intervals.IntervalArray;
import de.charite.compbio.jannovar.reference.GenomeInterval;
import de.charite.compbio.jannovar.reference.TranscriptModel;
/**
* This class encapsulates a chromosome and all of the genes its contains. It is intended to be used together with the
* {@link TranscriptModel} class to make a list of gene models that will be used to annotate chromosomal variants. We
* use an {@link IntervalArray} to store all of the {@link TranscriptModel} objects that belong to this Chromosome and
* to search for all transcripts that overlap with any given variant. Note that the IntervalTree class has functionality
* also to find the neighbors (5' and 3') of the closest gene in order to find the right and left genes of intergenic
* variants and to find the correct gene in the cases of complex regions of the chromosome with one gene located in the
* intron of the next or with overlapping genes.
*
* Note that the {@link GenomeInterval} objects in the interval tree are defined by the transcription start and stop
* sites of the isoform.
*
* @author Peter N Robinson
* @author Marten Jaeger
* @author Manuel Holtgrewe
*/
public final class Chromosome implements Serializable {
/** serial version ID */
private static final long serialVersionUID = 1L;
/** reference dictionary to use */
private final ReferenceDictionary refDict;
/** numeric chromsome ID */
private final int chrID;
/**
* An {@link IntervalArray} that contains all of the {@link TranscriptModel} objects for transcripts located on this
* chromosome.
*/
private final IntervalArray tmIntervalTree;
/**
* Initialize object.
*
* @param refDict
* the {@link ReferenceDictionary} to use
* @param chrID
* the chromosome
* @param tmIntervalTree
* An interval tree with all transcripts on this chromosome.
*/
public Chromosome(ReferenceDictionary refDict, int chrID, IntervalArray tmIntervalTree) {
this.refDict = refDict;
this.chrID = chrID;
this.tmIntervalTree = tmIntervalTree;
}
/** @return reference dictionary to use */
public ReferenceDictionary getRefDict() {
return refDict;
}
/** @return numeric chromsome ID */
public int getChrID() {
return chrID;
}
/**
* @return String representation of name of chromosome, e.g., "chr2"
*/
public String getChromosomeName() {
return refDict.getContigIDToName().get(chrID);
}
/**
* @return Number of genes contained in this chromosome.
*/
public int getNumberOfGenes() {
return this.tmIntervalTree.size();
}
/**
* @return the {@link IntervalArray} of the chromosome.
*/
public IntervalArray getTMIntervalTree() {
return tmIntervalTree;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy