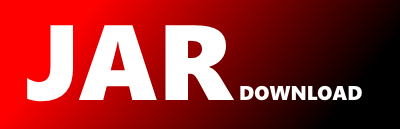
de.charite.compbio.jannovar.data.ReferenceDictionaryBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jannovar-core Show documentation
Show all versions of jannovar-core Show documentation
jannovar-core is a library for the functional annotation of genomic variants
package de.charite.compbio.jannovar.data;
import java.util.HashMap;
import com.google.common.collect.ImmutableMap;
// NOTE(holtgrem): Part of the public interface of the Jannovar library.
/**
* Builder class for incremental building of immutable {@link ReferenceDictionary} objects.
*
* @author Manuel Holtgrewe
*/
public final class ReferenceDictionaryBuilder {
/** interim map for {@link #contigID} */
private final HashMap tmpContigID = new HashMap();
/** builder for {@link ReferenceDictionary#contigID} */
private final ImmutableMap.Builder contigID = new ImmutableMap.Builder();
/** interim map for {@link #contigName} */
private final HashMap tmpContigName = new HashMap();
/** builder for {@link ReferenceDictionary#contigName} */
private final ImmutableMap.Builder contigName = new ImmutableMap.Builder();
/** interim map for {@link #contigLength} */
private final HashMap tmpContigLength = new HashMap();
/** builder for {@link ReferenceDictionary#contigLength} */
private final ImmutableMap.Builder contigLength = new ImmutableMap.Builder();
/**
* Allows contig length retrieval before final construction of the {@link ReferenceDictionary}.
*
* @param id
* id of the contig to get the length for
* @return length of the contig or null
if it could not be found
*/
public Integer getContigLength(int id) {
return tmpContigLength.get(id);
}
/**
* Add a contig id to length mapping.
*
* @param id
* numeric contig ID
* @param length
* contig length
*/
public void putContigLength(int id, int length) {
tmpContigLength.put(id, length);
contigLength.put(id, length);
}
/**
* Allows get contig name from a contig id.
*
* @param id
* numeric contig ID
* @return primary contig name or null
if none could be found
*/
public String getContigName(Integer id) {
return tmpContigName.get(id);
}
/**
* Set primary contig ID to name mapping.
*
* @param id
* numeric contig ID
* @param name
* contig name
*/
public void putContigName(int id, String name) {
tmpContigName.put(id, name);
contigName.put(id, name);
}
/**
* Allows contig name to numeric ID translation before final construction of the {@link ReferenceDictionary}.
*
* @param name
* name of contig to get numeric ID for
* @return canonical numeric ID for the contig with given name
or null
if none.
*/
public Integer getContigID(String name) {
return tmpContigID.get(name);
}
/**
* Add a contig name to numeric ID mapping to builder.
*
* @param name
* contig name
* @param id
* numeric contig ID
*/
public void putContigID(String name, int id) {
if (getContigID(name) != null)
return;
tmpContigID.put(name, id);
contigID.put(name, id);
}
/**
* @return instance of immutable ReferenceDictionary object
*/
public ReferenceDictionary build() {
return new ReferenceDictionary(contigID.build(), contigName.build(), contigLength.build());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy