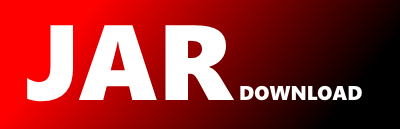
de.charite.compbio.jannovar.pedigree.Pedigree Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jannovar-core Show documentation
Show all versions of jannovar-core Show documentation
jannovar-core is a library for the functional annotation of genomic variants
package de.charite.compbio.jannovar.pedigree;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import de.charite.compbio.jannovar.Immutable;
// TODO(holtgrem): Test me!
/**
* Represent one pedigree from a PED file.
*
* @author Peter N Robinson
* @author Manuel Holtgrewe
*/
@Immutable
public final class Pedigree {
/** the pedigree's name */
private final String name;
/** the pedigree's members */
private final ImmutableList members;
/** mapping from member name to member */
private final ImmutableMap nameToMember;
/**
* Initialize the object with the given values
*
* @param name
* the name of the pedigree/family
* @param members
* list of the members
*/
public Pedigree(String name, Collection members) {
this.name = name;
this.members = ImmutableList.copyOf(members);
ImmutableMap.Builder mapBuilder = new ImmutableMap.Builder();
int i = 0;
for (Person person : members)
mapBuilder.put(person.getName(), new IndexedPerson(i++, person));
this.nameToMember = mapBuilder.build();
}
/**
* Initialize the object with the members of contents
that have the pedigree name equal to
* pedigreeName
.
*
* @param contents
* contents from the pedigree file
* @param pedigreeName
* name of the pedigree to extract
* @throws PedParseException
* in the case of problems with references to individuals for mother and father
*/
public Pedigree(PedFileContents contents, String pedigreeName) throws PedParseException {
this(pedigreeName, new PedigreeExtractor(pedigreeName, contents).run());
}
/** @return number of members in pedigree */
public int getNMembers() {
return members.size();
}
/** @return the pedigree's name */
public String getName() {
return name;
}
/** @return the pedigree's members */
public ImmutableList getMembers() {
return members;
}
/** @return mapping from member name to member */
public ImmutableMap getNameToMember() {
return nameToMember;
}
/**
* Obtain subset of members in a pedigree or change order.
*
* If a {@link Person} is selected that has parents in this
but the parent's name is not in
* name
then the {@link Person} will have null
as the parent object.
*
* @return Pedigree
with the members from names
in the given order
*/
public Pedigree subsetOfMembers(Collection names) {
HashSet nameSet = new HashSet();
nameSet.addAll(names);
ArrayList tmpMembers = new ArrayList();
for (String name : names)
if (hasPerson(name)) {
Person p = nameToMember.get(name).getPerson();
Person father = nameSet.contains(p.getFather().getName()) ? p.getFather() : null;
Person mother = nameSet.contains(p.getMother().getName()) ? p.getMother() : null;
tmpMembers.add(new Person(p.getName(), father, mother, p.getSex(), p.getDisease(), p.getExtraFields()));
}
return new Pedigree(name, tmpMembers);
}
/**
* @return a pedigree with one affected sample
*/
public static Pedigree constructSingleSamplePedigree(String sampleName) {
final Person person = new Person(sampleName, null, null, Sex.UNKNOWN, Disease.AFFECTED);
return new Pedigree("pedigree", ImmutableList.of(person));
}
/**
* @return true
if the pedigree contains a sample with the given name
.
*/
public boolean hasPerson(String name) {
return nameToMember.containsKey(name);
}
/**
* @return list of members, in the same order as in {@link #members}.
*/
public ImmutableList getNames() {
ImmutableList.Builder builder = new ImmutableList.Builder();
for (Person p : members)
builder.add(p.getName());
return builder.build();
}
@Override
public String toString() {
return "Pedigree [name=" + name + ", members=" + members + ", nameToMember=" + nameToMember + "]";
}
/**
* Helper class, used in the name to member map.
*/
public static class IndexedPerson {
private final int idx;
private final Person person;
public IndexedPerson(int idx, Person person) {
this.idx = idx;
this.person = person;
}
/** @return numeric index of person in pedigree */
public int getIdx() {
return idx;
}
/** @return the wrapped {@link Person} */
public Person getPerson() {
return person;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy