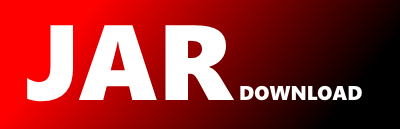
eu.fbk.knowledgestore.server.http.SecurityConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ks-server-http Show documentation
Show all versions of ks-server-http Show documentation
The HTTP server module (ks-server-http) implements the Web API of
the KnowledgeStore, which includes the two CRUD and SPARQL endpoints.
The CRUD Endpoint supports the retrieval and manipulation of
semi-structured data about resource, mention, entity and axiom records
(encoded in RDF, possibly using JSONLD), and the upload / download of
resource representation. The SPARQL Endpoint supports SPARQL SELECT,
CONSTRUCT, DESCRIBE and ASK queries according to the W3C SPARQL
protocol. The two endpoints are implemented on top of a component
implementing the KnowledgeStore Java API (the Store interface), which
can be either the the KnowledgeStore frontend (ks-frontend) or the Java
Client. The implementation of the module is based on the Jetty Web sever
(run in embedded mode) and the Jersey JAX-RS implementation. Reference
documentation of the Web API is automatically generated using the
Enunciate tool.
package eu.fbk.knowledgestore.server.http;
import java.net.URL;
import java.util.Arrays;
import java.util.Set;
import javax.annotation.Nullable;
import com.google.common.base.Joiner;
import com.google.common.base.Objects;
import com.google.common.base.Preconditions;
import com.google.common.collect.ImmutableSet;
import eu.fbk.knowledgestore.internal.Util;
public final class SecurityConfig {
public static final String ROLE_DOWNLOADER = "downloader";
public static final String ROLE_CRUD_READER = "crud_reader";
public static final String ROLE_SPARQL_READER = "sparql_reader";
public static final String ROLE_WRITER = "writer";
public static final String ROLE_UI_USER = "ui_user";
static final Set ALL_ROLES = ImmutableSet.of(ROLE_DOWNLOADER, ROLE_CRUD_READER,
ROLE_SPARQL_READER, ROLE_WRITER, ROLE_UI_USER);
@Nullable
private final String realm;
private final String userdbLocation;
private final URL userdbURL;
private final Set anonymousRoles;
public SecurityConfig(@Nullable final String realm, final String userdbLocation,
final String... anonymousRoles) {
this(realm, userdbLocation, Arrays.asList(anonymousRoles));
}
public SecurityConfig(@Nullable final String realm, final String userdbLocation,
final Iterable extends String> anonymousRoles) {
this.realm = realm;
this.userdbLocation = Preconditions.checkNotNull(userdbLocation);
this.userdbURL = Util.getURL(userdbLocation);
this.anonymousRoles = ImmutableSet.copyOf(anonymousRoles);
for (final String role : anonymousRoles) {
Preconditions.checkArgument(ALL_ROLES.contains(role), "Invalid role %s", role);
}
}
@Nullable
public String getRealm() {
return this.realm;
}
public String getUserdbLocation() {
return this.userdbLocation;
}
public URL getUserdbURL() {
return this.userdbURL;
}
public Set getAnonymousRoles() {
return this.anonymousRoles;
}
@Override
public boolean equals(final Object object) {
if (object == this) {
return true;
}
if (!(object instanceof SecurityConfig)) {
return false;
}
final SecurityConfig o = (SecurityConfig) object;
return Objects.equal(this.realm, o.realm) && this.userdbLocation.equals(o.userdbLocation)
&& this.anonymousRoles.equals(o.anonymousRoles);
}
@Override
public int hashCode() {
return Objects.hashCode(this.realm, this.userdbLocation, this.anonymousRoles);
}
@Override
public String toString() {
final StringBuilder builder = new StringBuilder();
if (this.realm != null) {
builder.append("realm=").append(this.realm).append(", ");
}
builder.append("userdb=").append(this.userdbLocation);
builder.append(", anonymousRoles=").append(Joiner.on(";").join(this.anonymousRoles));
return super.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy