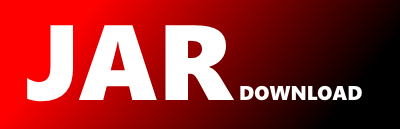
eu.fbk.knowledgestore.server.http.jaxrs.TableQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ks-server-http Show documentation
Show all versions of ks-server-http Show documentation
The HTTP server module (ks-server-http) implements the Web API of
the KnowledgeStore, which includes the two CRUD and SPARQL endpoints.
The CRUD Endpoint supports the retrieval and manipulation of
semi-structured data about resource, mention, entity and axiom records
(encoded in RDF, possibly using JSONLD), and the upload / download of
resource representation. The SPARQL Endpoint supports SPARQL SELECT,
CONSTRUCT, DESCRIBE and ASK queries according to the W3C SPARQL
protocol. The two endpoints are implemented on top of a component
implementing the KnowledgeStore Java API (the Store interface), which
can be either the the KnowledgeStore frontend (ks-frontend) or the Java
Client. The implementation of the module is based on the Jetty Web sever
(run in embedded mode) and the Jersey JAX-RS implementation. Reference
documentation of the Web API is automatically generated using the
Enunciate tool.
package eu.fbk.knowledgestore.server.http.jaxrs;
import java.io.IOException;
import java.util.List;
import java.util.Set;
import javax.annotation.Nullable;
import com.google.common.collect.HashMultiset;
import com.google.common.collect.Lists;
import com.google.common.collect.Multiset;
import com.google.common.collect.Ordering;
import com.google.common.collect.Sets;
import org.openrdf.model.URI;
import org.openrdf.model.Value;
import eu.fbk.knowledgestore.Session;
import eu.fbk.knowledgestore.data.Data;
import eu.fbk.knowledgestore.data.Record;
import eu.fbk.knowledgestore.data.Stream;
import eu.fbk.knowledgestore.vocabulary.KS;
public class TableQuery {
private static final int MAX_MENTIONS = 10000;
private final Session session;
public TableQuery(final Session session) {
this.session = session;
}
public void renderPropertyOccurrencesTable(final Appendable out, final URI entityURI)
throws Throwable {
// Compute the # of occurrences of each property URI in entity mentions
final Multiset propertyURIs = HashMultiset.create();
for (final Record mention : getEntityMentions(entityURI, MAX_MENTIONS)) {
propertyURIs.addAll(mention.getProperties());
}
// Render the table
renderMultisetTable(out, propertyURIs);
}
public void renderValueOccurrencesTable(final Appendable out, final URI entityURI,
final URI propertyURI) throws Throwable {
// Compute the # of occurrences of all the values of the given property in entity mentions
final Multiset propertyValues = HashMultiset.create();
for (final Record mention : getEntityMentions(entityURI, MAX_MENTIONS)) {
propertyValues.addAll(mention.get(propertyURI, Value.class));
}
// Render the table
renderMultisetTable(out, propertyValues);
}
public void renderMentionTable(final URI entity, final URI property, final String value,
final Appendable out) throws Throwable {
// Retrieve all the mentions satisfying the property[=value] optional filter
final List mentions = Lists.newArrayList();
for (final Record mention : getEntityMentions(entity, MAX_MENTIONS)) {
if (property == null || !mention.isNull(property)
&& (value == null || mention.get(property).contains(value))) {
mentions.add(mention);
}
}
// Render the mention table, including column toggling functionality
renderRecordsTable(out, mentions, null, true);
}
public Stream getEntityMentions(final URI entityURI, final int maxResults)
throws Throwable {
// First retrieve all the URIs of the mentions denoting the entity, via SPARQL query
final List mentionURIs = this.session
.sparql("SELECT ?mention WHERE { $$ gaf:denotedBy ?mention}", entityURI)
.execTuples().transform(URI.class, true, "mention").toList();
// Then return a stream that returns the mention records as they are fetched from the KS
return this.session.retrieve(KS.MENTION).limit((long) maxResults).ids(mentionURIs).exec();
}
public static T renderMultisetTable(final T out,
final Multiset> multiset) throws IOException {
out.append("\n");
out.append("\nValues Occurrences \n\n");
out.append("\n");
for (final Object element : multiset.elementSet()) {
final int occurrences = multiset.count(element);
out.append("").append(RenderUtils.render(element)).append(" ")
.append(Integer.toString(occurrences)).append(" \n");
}
out.append("\n
\n");
return out;
}
public static T renderRecordsTable(final T out,
final Iterable records, @Nullable List propertyURIs,
final boolean toggleColumns) throws IOException {
// Extract the properties to show if not explicitly supplied
if (propertyURIs == null) {
final Set uriSet = Sets.newHashSet();
for (final Record record : records) {
uriSet.addAll(record.getProperties());
}
propertyURIs = Ordering.from(Data.getTotalComparator()).sortedCopy(propertyURIs);
}
// Emit the panel for toggling displayed columns
out.append("Toggle column: | mentionURI |");
for (int i = 0; i < propertyURIs.size(); ++i) {
final String qname = RenderUtils.shortenURI(propertyURIs.get(i));
out.append(" ").append(qname).append(" | ");
}
out.append("");
// Emit the table
out.append("\n\nURI ");
for (final URI propertyURI : propertyURIs) {
out.append("").append(RenderUtils.shortenURI(propertyURI)).append(" ");
}
out.append(" \n\n\n");
for (final Record record : records) {
out.append("").append(RenderUtils.render(record.getID())).append(" ");
for (final URI propertyURI : propertyURIs) {
out.append("").append(RenderUtils.render(record.get(propertyURI)))
.append(" ");
}
out.append(" \n");
}
out.append("\n
\n");
return out;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy