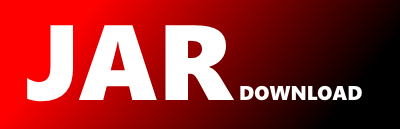
eu.fbk.knowledgestore.tool.TestUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ks-tool Show documentation
Show all versions of ks-tool Show documentation
A collection of command line tools for interacting with a KnowledgeStore server,
including benchmarking tools to create and perform a performance test of KS
retrieval methods as well as a tool for dumping the contents of a KS instance
to RDF files.
package eu.fbk.knowledgestore.tool;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import com.google.common.base.Preconditions;
import com.google.common.base.Strings;
import com.google.common.collect.Maps;
import org.openrdf.model.Value;
import org.openrdf.query.BindingSet;
import org.openrdf.query.impl.MapBindingSet;
import eu.fbk.knowledgestore.data.Data;
import eu.fbk.rdfpro.util.Namespaces;
import eu.fbk.rdfpro.util.Statements;
final class TestUtil {
// Properties manipulation
public static T read(final Properties properties, final String name, final Class type) {
final T value = read(properties, name, type, null);
if (value == null) {
throw new IllegalArgumentException("No value for mandatory property '" + name + "'");
}
return value;
}
public static T read(final Properties properties, final String name, final Class type,
final T defaultValue) {
final String value = properties.getProperty(name);
if (value == null) {
return defaultValue;
}
try {
return Data.convert(value, type);
} catch (final Throwable ex) {
throw new IllegalArgumentException("Invalid value for property '" + name + "': "
+ value + " (" + ex.getMessage() + ")");
}
}
public static void expand(final Properties targetProperties, final Properties defaultProperties) {
for (final Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy