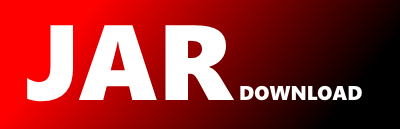
eu.stratosphere.util.ReflectionUtil Maven / Gradle / Ivy
/***********************************************************************************************************************
* Copyright (C) 2010-2013 by the Stratosphere project (http://stratosphere.eu)
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
**********************************************************************************************************************/
package eu.stratosphere.util;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
public class ReflectionUtil {
public static T newInstance(Class clazz) {
try {
return clazz.newInstance();
} catch (Exception e) {
throw new RuntimeException(e);
}
}
@SuppressWarnings("unchecked")
public static Class getTemplateType(Class> clazz, int num) {
return (Class) getSuperTemplateTypes(clazz)[num];
}
@SuppressWarnings("unchecked")
public static Class getTemplateType(Class> clazz, Class> classWithParameter, int num) {
return (Class) getSuperTemplateTypes(clazz)[num];
}
public static Class getTemplateType1(Class> clazz) {
return getTemplateType(clazz, 0);
}
public static Class getTemplateType2(Class> clazz) {
return getTemplateType(clazz, 1);
}
public static Class getTemplateType3(Class> clazz) {
return getTemplateType(clazz, 2);
}
public static Class getTemplateType4(Class> clazz) {
return getTemplateType(clazz, 3);
}
public static Class getTemplateType5(Class> clazz) {
return getTemplateType(clazz, 4);
}
public static Class getTemplateType6(Class> clazz) {
return getTemplateType(clazz, 5);
}
public static Class getTemplateType7(Class> clazz) {
return getTemplateType(clazz, 6);
}
public static Class getTemplateType8(Class> clazz) {
return getTemplateType(clazz, 7);
}
public static Class>[] getSuperTemplateTypes(Class> clazz) {
Type type = clazz.getGenericSuperclass();
while (true) {
if (type instanceof ParameterizedType) {
return getTemplateTypes((ParameterizedType) type);
}
if (clazz.getGenericSuperclass() == null) {
throw new IllegalArgumentException();
}
type = clazz.getGenericSuperclass();
clazz = clazz.getSuperclass();
}
}
public static Class>[] getSuperTemplateTypes(Class> clazz, Class> searchedSuperClass) {
if (clazz == null || searchedSuperClass == null) {
throw new NullPointerException();
}
Class> superClass = null;
do {
superClass = clazz.getSuperclass();
if (superClass == searchedSuperClass) {
break;
}
}
while ((clazz = superClass) != null);
if (clazz == null) {
throw new IllegalArgumentException("The searched for superclass is not a superclass of the given class.");
}
final Type type = clazz.getGenericSuperclass();
if (type instanceof ParameterizedType) {
return getTemplateTypes((ParameterizedType) type);
}
else {
throw new IllegalArgumentException("The searched for superclass is not a generic class.");
}
}
public static Class>[] getTemplateTypes(ParameterizedType paramterizedType) {
Class>[] types = new Class>[paramterizedType.getActualTypeArguments().length];
int i = 0;
for (Type templateArgument : paramterizedType.getActualTypeArguments()) {
assert (templateArgument instanceof Class>);
types[i++] = (Class>) templateArgument;
}
return types;
}
public static Class>[] getTemplateTypes(Class> clazz) {
Type type = clazz.getGenericSuperclass();
assert (type instanceof ParameterizedType);
ParameterizedType paramterizedType = (ParameterizedType) type;
Class>[] types = new Class>[paramterizedType.getActualTypeArguments().length];
int i = 0;
for (Type templateArgument : paramterizedType.getActualTypeArguments()) {
assert (templateArgument instanceof Class>);
types[i++] = (Class>) templateArgument;
}
return types;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy