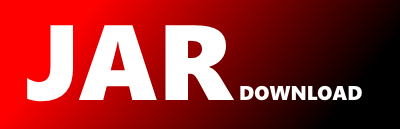
eu.stratosphere.api.java.operators.translation.PlanUnwrappingCoGroupOperator Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* Copyright (C) 2010-2013 by the Stratosphere project (http://stratosphere.eu)
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
**********************************************************************************************************************/
package eu.stratosphere.api.java.operators.translation;
import java.util.Iterator;
import eu.stratosphere.api.common.functions.GenericCoGrouper;
import eu.stratosphere.api.common.operators.BinaryOperatorInformation;
import eu.stratosphere.api.common.operators.base.CoGroupOperatorBase;
import eu.stratosphere.api.java.functions.CoGroupFunction;
import eu.stratosphere.api.java.operators.Keys;
import eu.stratosphere.api.java.tuple.Tuple2;
import eu.stratosphere.types.TypeInformation;
import eu.stratosphere.util.Collector;
public class PlanUnwrappingCoGroupOperator
extends CoGroupOperatorBase, Tuple2, OUT, GenericCoGrouper, Tuple2, OUT>>
{
public PlanUnwrappingCoGroupOperator(CoGroupFunction udf,
Keys.SelectorFunctionKeys key1, Keys.SelectorFunctionKeys key2, String name,
TypeInformation type, TypeInformation> typeInfoWithKey1, TypeInformation> typeInfoWithKey2)
{
super(new TupleUnwrappingCoGrouper(udf),
new BinaryOperatorInformation, Tuple2, OUT>(typeInfoWithKey1, typeInfoWithKey2, type),
key1.computeLogicalKeyPositions(), key2.computeLogicalKeyPositions(), name);
}
public PlanUnwrappingCoGroupOperator(CoGroupFunction udf,
int[] key1, Keys.SelectorFunctionKeys key2, String name,
TypeInformation type, TypeInformation> typeInfoWithKey1, TypeInformation> typeInfoWithKey2)
{
super(new TupleUnwrappingCoGrouper(udf),
new BinaryOperatorInformation, Tuple2, OUT>(typeInfoWithKey1,typeInfoWithKey2, type),
new int[]{0}, key2.computeLogicalKeyPositions(), name);
}
public PlanUnwrappingCoGroupOperator(CoGroupFunction udf,
Keys.SelectorFunctionKeys key1, int[] key2, String name,
TypeInformation type, TypeInformation> typeInfoWithKey1, TypeInformation> typeInfoWithKey2)
{
super(new TupleUnwrappingCoGrouper(udf),
new BinaryOperatorInformation, Tuple2, OUT>(typeInfoWithKey1,typeInfoWithKey2, type),
key1.computeLogicalKeyPositions(), new int[]{0}, name);
}
// --------------------------------------------------------------------------------------------
public static final class TupleUnwrappingCoGrouper extends WrappingFunction>
implements GenericCoGrouper, Tuple2, OUT>
{
private static final long serialVersionUID = 1L;
private final TupleUnwrappingIterator iter1;
private final TupleUnwrappingIterator iter2;
private TupleUnwrappingCoGrouper(CoGroupFunction wrapped) {
super(wrapped);
this.iter1 = new TupleUnwrappingIterator();
this.iter2 = new TupleUnwrappingIterator();
}
@Override
public void coGroup(Iterator> records1, Iterator> records2, Collector out) throws Exception {
iter1.set(records1);
iter2.set(records2);
this.wrappedFunction.coGroup(iter1, iter2, out);
}
}
public static class UnwrappingKeyIterator implements Iterator {
private Iterator> outerIterator;
I1 firstValue;
public UnwrappingKeyIterator(Iterator> records1) {
this.outerIterator = records1;
this.firstValue = null;
}
public UnwrappingKeyIterator(Iterator> records1, I1 firstValue ) {
this.outerIterator = records1;
this.firstValue = firstValue;
}
@Override
public boolean hasNext() {
return firstValue != null || outerIterator.hasNext();
}
@Override
public I1 next() {
if(firstValue != null) {
firstValue = null;
return firstValue;
}
return outerIterator.next().getField(1);
}
@Override
public void remove() {
throw new UnsupportedOperationException();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy