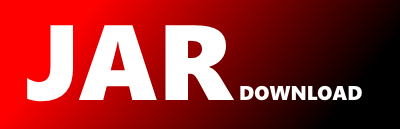
eu.stratosphere.api.java.operators.translation.WrappingFunction Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* Copyright (C) 2010-2013 by the Stratosphere project (http://stratosphere.eu)
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
**********************************************************************************************************************/
package eu.stratosphere.api.java.operators.translation;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import eu.stratosphere.api.common.accumulators.Accumulator;
import eu.stratosphere.api.common.accumulators.DoubleCounter;
import eu.stratosphere.api.common.accumulators.Histogram;
import eu.stratosphere.api.common.accumulators.IntCounter;
import eu.stratosphere.api.common.accumulators.LongCounter;
import eu.stratosphere.api.common.aggregators.Aggregator;
import eu.stratosphere.api.common.cache.DistributedCache;
import eu.stratosphere.api.common.functions.AbstractFunction;
import eu.stratosphere.api.common.functions.IterationRuntimeContext;
import eu.stratosphere.api.common.functions.RuntimeContext;
import eu.stratosphere.configuration.Configuration;
import eu.stratosphere.types.Value;
public abstract class WrappingFunction extends AbstractFunction {
private static final long serialVersionUID = 1L;
protected final T wrappedFunction;
protected WrappingFunction(T wrappedFunction) {
this.wrappedFunction = wrappedFunction;
}
@Override
public void open(Configuration parameters) throws Exception {
this.wrappedFunction.open(parameters);
}
@Override
public void close() throws Exception {
this.wrappedFunction.close();
}
@Override
public void setRuntimeContext(RuntimeContext t) {
super.setRuntimeContext(t);
if (t instanceof IterationRuntimeContext) {
this.wrappedFunction.setRuntimeContext(new WrappingIterationRuntimeContext(t));
}
else{
this.wrappedFunction.setRuntimeContext(new WrappingRuntimeContext(t));
}
}
private static class WrappingRuntimeContext implements RuntimeContext {
protected final RuntimeContext context;
protected WrappingRuntimeContext(RuntimeContext context) {
this.context = context;
}
@Override
public String getTaskName() {
return context.getTaskName();
}
@Override
public int getNumberOfParallelSubtasks() {
return context.getNumberOfParallelSubtasks();
}
@Override
public int getIndexOfThisSubtask() {
return context.getIndexOfThisSubtask();
}
@Override
public void addAccumulator(String name, Accumulator accumulator) {
context.addAccumulator(name, accumulator);
}
@Override
public Accumulator getAccumulator(String name) {
return context.getAccumulator(name);
}
@Override
public HashMap> getAllAccumulators() {
return context.getAllAccumulators();
}
@Override
public IntCounter getIntCounter(String name) {
return context.getIntCounter(name);
}
@Override
public LongCounter getLongCounter(String name) {
return context.getLongCounter(name);
}
@Override
public DoubleCounter getDoubleCounter(String name) {
return context.getDoubleCounter(name);
}
@Override
public Histogram getHistogram(String name) {
return context.getHistogram(name);
}
@Override
public
© 2015 - 2025 Weber Informatics LLC | Privacy Policy