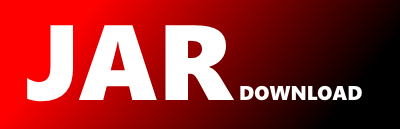
org.onebusaway.gtfs_transformer.updates.CalendarSimplicationStrategy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of onebusaway-gtfs-transformer Show documentation
Show all versions of onebusaway-gtfs-transformer Show documentation
A Java library for transforming Google Transit Feed Spec feeds
The newest version!
/**
* Copyright (C) 2011 Google, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.onebusaway.gtfs_transformer.updates;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.onebusaway.collections.FactoryMap;
import org.onebusaway.csv_entities.schema.annotations.CsvField;
import org.onebusaway.gtfs.impl.calendar.CalendarServiceDataFactoryImpl;
import org.onebusaway.gtfs.model.AgencyAndId;
import org.onebusaway.gtfs.model.Route;
import org.onebusaway.gtfs.model.ServiceCalendar;
import org.onebusaway.gtfs.model.ServiceCalendarDate;
import org.onebusaway.gtfs.model.Trip;
import org.onebusaway.gtfs.model.calendar.ServiceDate;
import org.onebusaway.gtfs.services.GtfsDao;
import org.onebusaway.gtfs.services.GtfsMutableRelationalDao;
import org.onebusaway.gtfs.services.calendar.CalendarService;
import org.onebusaway.gtfs_transformer.impl.RemoveEntityLibrary;
import org.onebusaway.gtfs_transformer.services.GtfsTransformStrategy;
import org.onebusaway.gtfs_transformer.services.TransformContext;
import org.onebusaway.gtfs_transformer.updates.CalendarSimplicationLibrary.ServiceCalendarSummary;
public class CalendarSimplicationStrategy implements GtfsTransformStrategy {
private static Pattern _mergedIdPattern = Pattern.compile("^(.*)_merged_(.*)$");
@CsvField(ignore = true)
private CalendarSimplicationLibrary _library = new CalendarSimplicationLibrary();
// This is only really here so that TransformFactory will detect it as a
// user-specified argument.
@CsvField(optional = true)
private int minNumberOfWeeksForCalendarEntry;
// This is only really here so that TransformFactory will detect it as a
// user-specified argument.
@CsvField(optional = true)
private double dayOfTheWeekInclusionRatio;
@CsvField(optional = true)
private boolean undoGoogleTransitDataFeedMergeTool = false;
public void setMinNumberOfWeeksForCalendarEntry(
int minNumberOfWeeksForCalendarEntry) {
_library.setMinNumberOfWeeksForCalendarEntry(minNumberOfWeeksForCalendarEntry);
}
public void setDayOfTheWeekInclusionRatio(double dayOfTheWeekInclusionRatio) {
_library.setDayOfTheWeekInclusionRatio(dayOfTheWeekInclusionRatio);
}
public void setUndoGoogleTransitDataFeedMergeTool(
boolean undoGoogleTransitDataFeedMergeTool) {
this.undoGoogleTransitDataFeedMergeTool = undoGoogleTransitDataFeedMergeTool;
}
public boolean isUndoGoogleTransitDataFeedMergeTool() {
return undoGoogleTransitDataFeedMergeTool;
}
public CalendarSimplicationLibrary getLibrary() {
return _library;
}
@Override
public String getName() {
return this.getClass().getSimpleName();
}
@Override
public void run(TransformContext context, GtfsMutableRelationalDao dao) {
RemoveEntityLibrary removeEntityLibrary = new RemoveEntityLibrary();
Map, AgencyAndId> serviceIdsToUpdatedServiceId = new HashMap, AgencyAndId>();
Map> mergeToolIdMapping = computeMergeToolIdMapping(dao);
for (Route route : dao.getAllRoutes()) {
Map> tripsByKey = TripKey.groupTripsForRouteByKey(
dao, route);
Map, List> tripKeysByServiceIds = _library.groupTripKeysByServiceIds(tripsByKey);
for (Set serviceIds : tripKeysByServiceIds.keySet()) {
AgencyAndId updatedServiceId = createUpdatedServiceId(
serviceIdsToUpdatedServiceId, serviceIds);
for (TripKey tripKey : tripKeysByServiceIds.get(serviceIds)) {
List tripsForKey = tripsByKey.get(tripKey);
Trip tripToKeep = tripsForKey.get(0);
tripToKeep.setServiceId(updatedServiceId);
for (int i = 1; i < tripsForKey.size(); i++) {
Trip trip = tripsForKey.get(i);
removeEntityLibrary.removeTrip(dao, trip);
}
if (undoGoogleTransitDataFeedMergeTool) {
AgencyAndId updatedTripId = computeUpdatedTripIdForMergedTripsIfApplicable(
mergeToolIdMapping, tripsForKey);
if (updatedTripId != null) {
tripToKeep.setId(updatedTripId);
}
}
}
}
}
CalendarService calendarService = CalendarServiceDataFactoryImpl.createService(dao);
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy