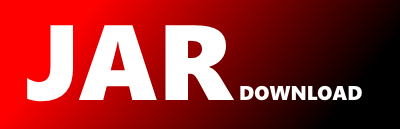
net.sf.blunder.service.persistence.opencsv.BeanToCsv Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of blunder Show documentation
Show all versions of blunder Show documentation
Blunder is an automated tool for analyzing chained exceptions in Java. It's usefull for classify,
generate a customized error message and a list for possible solutions.
The aim of this project is to provide a tool to identify different error contexts, analyze them
and assemble a customized response to an application end-user or another application.
The newest version!
/*
* Blunder is an automated tool for analyzing chained exceptions.
* Copyright (C) 2009 - Ambrosi Lucas
*
* This file is part of Blunder.
*
* Blunder is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License
* version 3 as published by the Free Software Foundation.
*
* Blunder is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Blunder. If not, see .
*/
package net.sf.blunder.service.persistence.opencsv;
import au.com.bytecode.opencsv.CSVWriter;
import java.beans.Introspector;
import java.beans.PropertyDescriptor;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import net.sf.blunder.BlunderException;
import org.apache.log4j.Logger;
public class BeanToCsv {
private static final Logger log = Logger.getLogger( BeanToCsv.class );
private boolean wroteHeader;
private boolean writeHeaders;
private boolean writeDeprecated;
public BeanToCsv( boolean writeHeaders, boolean writeDeprecated ) {
setWriteDeprecated( writeDeprecated );
setWriteHeaders( writeHeaders );
setWroteHeader( false );
}
public void writeBean( CSVWriter writer, Object bean ) {
writeBean( writer, bean, java.lang.Object.class );
}
public void writeBean( CSVWriter writer, Object bean, Class> stopClass ) {
if ( bean == null ) {
throw new NullPointerException( "Bean can not be null!" );
}
writeBean( writer, bean, resolvePropertyDescriptors( bean.getClass(), stopClass ) );
}
public void writeBean( CSVWriter writer, Object bean, List descriptors ) {
try {
List values = new ArrayList();
for ( PropertyDescriptor pd : descriptors ) {
Object value = pd.getReadMethod().invoke( bean, new Object[]{} );
values.add( value == null ? "" : value.toString() );
}
if ( isWriteHeaders() && !isWroteHeader() ) {
writeHeaders( writer, descriptors );
}
writer.writeNext( values.toArray( new String[]{} ) );
} catch ( Exception e ) {
throw new BlunderException( "Error writing bean", e );
}
}
public void writeAllBeans( CSVWriter writer, List> beans ) {
writeAllBeans( writer, beans, java.lang.Object.class );
}
public void writeAllBeans( CSVWriter writer, List> beans, Class> stopClass ) {
List descriptors = resolvePropertyDescriptors( beans.get( 0 ).getClass(), stopClass );
if ( isWriteHeaders() && !isWroteHeader() ) {
writeHeaders( writer, descriptors );
}
for ( Object bean : beans ) {
writeBean( writer, bean, descriptors );
}
}
private void writeHeaders( CSVWriter writer, List descriptors ) {
List headers = new ArrayList();
for ( PropertyDescriptor pd : descriptors ) {
headers.add( pd.getName() );
}
writer.writeNext( headers.toArray( new String[]{} ) );
setWroteHeader( true );
}
private List resolvePropertyDescriptors( Class> beanClass, Class> stopClass ) {
PropertyDescriptor[] descs = null;
try {
descs = Introspector.getBeanInfo( beanClass, stopClass ).getPropertyDescriptors();
} catch ( Exception e ) {
throw new BlunderException( "Error writing bean", e );
}
if ( isWriteDeprecated() ) {
return Arrays.asList( descs );
}
List descriptors = new ArrayList();
for ( PropertyDescriptor pd : descs ) {
if ( pd.getWriteMethod().getAnnotation( Deprecated.class ) != null ) {
log.debug( "Filtering property named [" + pd.getName() + "] for being annotated with @Deprecated!" );
} else {
descriptors.add( pd );
}
}
return descriptors;
}
public boolean isWriteHeaders() {
return writeHeaders;
}
public void setWriteHeaders( boolean writeHeaders ) {
this.writeHeaders = writeHeaders;
}
public boolean isWriteDeprecated() {
return writeDeprecated;
}
public void setWriteDeprecated( boolean writeDeprecated ) {
this.writeDeprecated = writeDeprecated;
}
public boolean isWroteHeader() {
return wroteHeader;
}
public void setWroteHeader( boolean wroteHeader ) {
this.wroteHeader = wroteHeader;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy