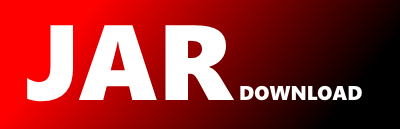
umcg.genetica.collections.ChrPosMap Maven / Gradle / Ivy
package umcg.genetica.collections;
import com.google.common.collect.Iterators;
import gnu.trove.map.TIntObjectMap;
import gnu.trove.map.hash.TIntObjectHashMap;
import java.util.HashMap;
import java.util.Iterator;
/**
*
* @author Patrick Deelen
*/
public class ChrPosMap implements Iterable{
private final HashMap> data;
public ChrPosMap() {
data = new HashMap>();
}
public void put(String chr, int pos, E element){
TIntObjectMap chrElements = data.get(chr);
if(chrElements == null){
chrElements = new TIntObjectHashMap();
data.put(chr, chrElements);
}
chrElements.put(pos, element);
}
/**
*
* @param chr
* @param pos
* @return null if not found
*/
public E get(String chr, int pos){
TIntObjectMap chrElements = data.get(chr);
if(chrElements == null){
return null;
} else {
return chrElements.get(pos);
}
}
/**
*
* @param chr
* @param pos
* @return the removed element or null
*/
public E remove(String chr, int pos){
TIntObjectMap chrElements = data.get(chr);
if(chrElements == null){
return null;
} else {
return chrElements.remove(pos);
}
}
public Iterator chrIterator(String chr) {
TIntObjectMap chrElements = data.get(chr);
if(chrElements == null){
return null;
} else {
return chrElements.valueCollection().iterator();
}
}
@Override
public Iterator iterator() {
Iterator[] chrIterators = new Iterator[data.size()];
int i = 0;
for(TIntObjectMap chrData : data.values()){
chrIterators[i] = chrData.valueCollection().iterator();
++i;
}
return Iterators.concat(chrIterators);
}
public int size(){
int count = 0;
for(TIntObjectMap chrResults : data.values()){
count += chrResults.size();
}
return count;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy