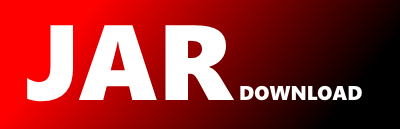
umcg.genetica.io.gwascatalog.GWASTrait Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package umcg.genetica.io.gwascatalog;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
/**
*
* @author harmjan
*/
public class GWASTrait {
int id;
String name;
String cleanName;
HashSet loci = new HashSet();
HashSet publishedIn = new HashSet();
HashSet snps = new HashSet();
HashSet strongestSNPAssociation = new HashSet();
GWASSNP[] snpArray = null;
HashSet reportedGenes = new HashSet();
HashSet mappedGenes = new HashSet();
public String getName() {
return name;
}
public String getCleanName() {
return cleanName;
}
public GWASSNP[] getSNPs() {
if (snpArray == null) {
snpArray = snps.toArray(new GWASSNP[0]);
}
return snpArray;
}
public GWASSNP[] getSNPs(double pThreshold) {
if (snpArray == null) {
snpArray = snps.toArray(new GWASSNP[0]);
}
List limited = new ArrayList();
for (GWASSNP snp : snpArray) {
Double p = snp.getPValueAssociatedWithTrait(this);
if (p != null && p <= pThreshold) {
limited.add(snp);
}
}
return limited.toArray(new GWASSNP[0]);
}
public HashSet getReportedGenes() {
return reportedGenes;
}
public void setReportedGenes(HashSet reportedGenes) {
this.reportedGenes = reportedGenes;
}
public void appendReportedGenes(HashSet reportedGenes) {
this.reportedGenes.addAll(reportedGenes);
}
public HashSet getMappedGenes() {
return mappedGenes;
}
public void setMappedGenes(HashSet mappedGenes) {
this.mappedGenes = mappedGenes;
}
public void appendMappedGenes(HashSet mappedGenes) {
this.mappedGenes.addAll(reportedGenes);
}
@Override
public String toString() {
return name;
}
public void addTopSNP(GWASSNP gwasTopSNPObj) {
this.strongestSNPAssociation.add(gwasTopSNPObj);
this.snps.add(gwasTopSNPObj);
}
public GWASSNP[] getTopAssociations(){
return strongestSNPAssociation.toArray(new GWASSNP[0]);
}
public boolean isTopAssociation(GWASSNP snp){
return strongestSNPAssociation.contains(snp);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy