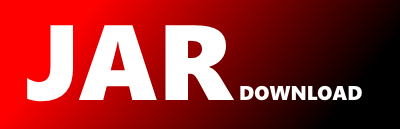
umcg.genetica.math.matrix.SymmetricShortDistanceMatrix Maven / Gradle / Ivy
/*
* SymmetricShortDistancaMatrix.java
*
* Created on 04 June 2004, 16:03
*/
package umcg.genetica.math.matrix;
/**
*
* Symmetric Short Distance Matrix: A memory efficient matrix capable of performing calculations on all genes x all genes:
*
* @author Lude Franke
*/
public class SymmetricShortDistanceMatrix {
private int size;
private short[] matrix;
private final static double eLog10 = java.lang.Math.log(10);
private final static int MAX_ALL_PAIRS_STEPS = 50;
public final static int MAX_VALUE = Short.MAX_VALUE + 32768;
private long[] elementIndex;
/** Creates a new instance of SymmetricShortDistancaMatrix
*
* Defines a new Symmetric matrix, with a predefined size
*
* Initially all values will be Short.MAX_Value (65535)
*
* All data is stored in memory efficient one dimensional array, which costs size * (size + 1) bytes
*
*/
public SymmetricShortDistanceMatrix(int size) {
this.size = size;
long arraySize = ((long) size * (long) (size + 1)) / 2l;
matrix = new short[(int) arraySize];
elementIndex = new long[size]; for (int x=0; xy) {
return elementIndex[y] + (long) x;
} else {
return elementIndex[x] + (long) y;
}
}
/* Set the value for point (x,y):
*/
public void set(int x, int y, int value) {
matrix[(int) getElement(x,y)] = (short) (value - 32768);
}
/* Get the value for point (x,y):
*/
public int get(int x, int y) {
return matrix[(int) getElement(x,y)] + 32768;
}
/* Get size of matrix:
*/
public int size() {
return size;
}
public int maxValue() {
return Short.MAX_VALUE + 32768;
}
/* Get the shortest path for all the pairs using the Floyd-Warshall algorithm:
*
* Please be aware that this method replaces the values inside the current matrix with the shortest path values!
*/
public void getAllPairsShortestPath() {
//This method uses the Floyd-Warshall all pairs shortest path algorithm:
//As we deal here with a symmetric matrix, the number of required calculations is 50% less.
//Copy matrix:
//System.out.println("Copying matrix to temporary array:");
short[][] matrixCopy = new short[size()][size()];
for (int x=0; x32767) value = 32767;
matrixCopy[x][y] = (short) (value);
matrixCopy[y][x] = (short) (value);
}
}
for (int v=0; v1000 && k%10==9) System.out.println(k);
/*
if (k%10==9&&sz>20000) {
System.out.print(k + " ");
}
if (k%100==99&&sz>20000) {
System.out.println("");
for (int x=0; x<4; x++) {
System.out.print("\t" + genes[x]);
}
System.out.println("");
for (int x=0; x<4; x++) {
System.out.print(genes[x]);
for (int y=0; y<4; y++) {
System.out.print("\t" + matrixCopy[genes[x]][genes[y]]);
}
System.out.println("");
}
}
*/
}
System.out.println("");
}
/* Get the shortest path from node X to node Y.
*
* Returns a Vector containing the nodes as Integers that in the correct order make up the path. Empty if no path exists.
*
* This algorithm uses the Dijkstra's shortest path algorithm.
*
* Please be aware that it does not use a Fibonacci heap. Implementing this improves the performance considerably.
*/
public java.util.Vector getShortestPath(int x, int y) {
//This method uses the Dijkstra's shortest path algorithm:
//Create path which eventually will contain all the nodes in the shortest path from x to y:
java.util.Vector path = new java.util.Vector();
//Initialise S: Array indicating whether node is already included and traversed
boolean S[] = new boolean[size()];
//Initialise T: Two dimensional array, column 0: distance, column 1: nodeID predecessor:
int T[][] = new int[2][size()];
for (int i=0; i0) {
pathPossible = true;
}
}
//Hypothesis: a path does exist between nodeStart and nodeEnd:
boolean pathExists = true;
//Traverse path, do not stop:
boolean traversePath = true;
//Perform Dijkstra algorithm loop, as long as a path is possible, exists and no stop request has been raised:
while (pathPossible && pathExists && traversePath) {
//Set minimal distance T:
int tMin = maxValue();
//Find node v that is nearest to nodeStart:
int v = -1;
//Traverse all nodes:
for (int i=0; i0) {
//Check whether the distance of the edge between the processed node and node v is the lowest:
if (T[0][v] + get(v, i) < T[0][i]) {
//Change the distance and nodeIndex of the i:
T[0][i] = T[0][v] + (int) get(v,i);
T[1][i] = v;
}
}
}
//Check whether destination has been reached:
if (S[y]) {
traversePath = false;
} else {
//Check whether the path still between nodeStart and nodeEnd is still likely to exist:
pathExists = false;
for (int i=0; i 0){
distance = (int)(-(double)Short.MIN_VALUE + ((correlation) * ((double)Short.MAX_VALUE)));
} else {
distance = (int)(-(double)Short.MIN_VALUE + ((correlation) * (double)Short.MIN_VALUE));
}
return distance;
}
public double getCorrelationFromDistance(int distance){
double correlation;
if (distance > -Short.MIN_VALUE){
correlation = (((double)distance + (double) Short.MIN_VALUE)/(double)Short.MAX_VALUE);
}else{
correlation = (((double)distance + (double) Short.MIN_VALUE)/(-(double)Short.MIN_VALUE));
}
return correlation;
}
public void save(java.io.File fileName) {
try {
//Initialize output stream:
java.io.OutputStream out = new java.io.BufferedOutputStream(new java.io.FileOutputStream(fileName));
//Define the buffer size:
int bufferLength = 2500 * 1024;
byte[] buffer = new byte[bufferLength * 2];
//Define length of read bytes:
int len = 0;
//Initialize location where data is and should go:
int loc = 0;
while(1==1){
// Copy data
if (loc + bufferLength <= matrix.length) {
len = bufferLength;
int bufferLoc = 0;
for (int x=loc; x> 8);
buffer[bufferLoc+1] = (byte)(matrix[x] & 0xff);
bufferLoc+=2;
}
loc+= len;
System.out.print(".");
out.write(buffer);
} else {
len = matrix.length - loc;
buffer = new byte[len * 2];
int bufferLoc = 0;
for (int x=loc; x> 8);
buffer[bufferLoc+1] = (byte)(matrix[x] & 0xff);
bufferLoc+=2;
}
out.write(buffer);
System.out.print(".");
break;
}
}
System.out.println("");
//Dereference buffer:
buffer = null;
//Close file:
out.close();
} catch (Exception e) {
System.out.println("Cannot write to file! (" + e.getMessage() + ")");
}
}
public void load(java.io.File fileName) {
try {
//Get random access to file:
//java.io.RandomAccessFile file = new java.io.RandomAccessFile(fileName,"rw");
java.io.InputStream in = new java.io.BufferedInputStream(new java.io.FileInputStream(fileName));
//Get the size of the file
long length = fileName.length();
//Read contents, check that size is same as defined matrix size:
long matrixLength = 2l * (long) matrix.length;
if (length==matrixLength) {
//Define the buffer size:
int bufferLength = 5000 * 1024;
byte[] buffer = new byte[bufferLength];
//Define length of read bytes:
int len = 0;
//Initialize location where data is and should go:
int loc = 0;
while((len = in.read(buffer, 0, bufferLength)) != -1){
// copy bytes to short:
for (int x=0; x
© 2015 - 2025 Weber Informatics LLC | Privacy Policy