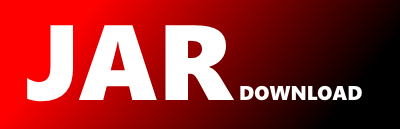
nz.co.senanque.pizzaorder.instances.Pizza Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of madura-perspectives-pizzaorder Show documentation
Show all versions of madura-perspectives-pizzaorder Show documentation
This is a rules-driven pizza configuration which lets you pick different pizza bases, toppings and sizes, except that only some combinations are allowed and the rules enforce this. The rules, UI and underlying domain objects are all delivered by this sub-application.
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.4
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2016.03.30 at 06:05:58 PM NZDT
//
package nz.co.senanque.pizzaorder.instances;
import java.io.Serializable;
import javax.persistence.Basic;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.Inheritance;
import javax.persistence.InheritanceType;
import javax.persistence.Table;
import javax.persistence.Transient;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlTransient;
import javax.xml.bind.annotation.XmlType;
import nz.co.senanque.validationengine.ObjectMetadata;
import nz.co.senanque.validationengine.ValidationObject;
import nz.co.senanque.validationengine.ValidationSession;
import nz.co.senanque.validationengine.ValidationUtils;
import nz.co.senanque.validationengine.annotations.ChoiceList;
import nz.co.senanque.validationengine.annotations.Description;
import nz.co.senanque.validationengine.annotations.Digits;
import nz.co.senanque.validationengine.annotations.Inactive;
import nz.co.senanque.validationengine.annotations.Label;
import nz.co.senanque.validationengine.annotations.Range;
import nz.co.senanque.validationengine.annotations.ReadOnly;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
/**
* Java class for Pizza complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Pizza">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="id" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="amount" type="{http://www.w3.org/2001/XMLSchema}double"/>
* <element name="name" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="description" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="size">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <maxLength value="20"/>
* </restriction>
* </simpleType>
* </element>
* <element name="base">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <maxLength value="20"/>
* </restriction>
* </simpleType>
* </element>
* <element name="topping">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <maxLength value="20"/>
* </restriction>
* </simpleType>
* </element>
* <element name="testing">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <maxLength value="20"/>
* </restriction>
* </simpleType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Pizza", propOrder = {
"id",
"amount",
"name",
"description",
"size",
"base",
"topping",
"testing"
})
@Entity(name = "Pizza")
@Table(name = "PIZZA")
@Inheritance(strategy = InheritanceType.JOINED)
public class Pizza
implements Serializable, ValidationObject, Equals, HashCode, ToString
{
protected long id;
@XmlElement(defaultValue = "0")
protected double amount;
@XmlElement(required = true)
protected String name;
@XmlElement(required = true)
protected String description;
@XmlElement(required = true)
protected String size;
@XmlElement(required = true, defaultValue = "GlutenFree")
protected String base;
@XmlElement(required = true)
protected String topping;
@XmlElement(required = true, defaultValue = "")
protected String testing;
@XmlTransient
protected ValidationSession m_validationSession;
@XmlTransient
protected ObjectMetadata m_metadata;
@XmlTransient
public final static String ID = "id";
@XmlTransient
public final static String AMOUNT = "amount";
@XmlTransient
public final static String NAME = "name";
@XmlTransient
public final static String DESCRIPTION = "description";
@XmlTransient
public final static String SIZE = "size";
@XmlTransient
public final static String BASE = "base";
@XmlTransient
public final static String TOPPING = "topping";
@XmlTransient
public final static String TESTING = "testing";
public Pizza() {
ValidationUtils.setDefaults(this);
}
/**
* Gets the value of the id property.
*
*/
@Id
@Column(name = "ID", scale = 0)
public long getId() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return id;
}
/**
* Sets the value of the id property.
*
*/
public void setId(long value) {
getMetadata().removeUnknown("id");
if (m_validationSession!= null) {
m_validationSession.set(this, "id", value, id);
}
this.id = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "id", value, id);
}
}
/**
* Gets the value of the amount property.
*
*/
@Label(labelName = "Amount")
@Digits(fractionalDigits = "2", integerDigits = "8")
@Range(minInclusive = "5", maxInclusive = "1000")
@ReadOnly
@Basic
@Column(name = "AMOUNT", precision = 20, scale = 10)
public double getAmount() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return amount;
}
/**
* Sets the value of the amount property.
*
*/
public void setAmount(double value) {
getMetadata().removeUnknown("amount");
if (m_validationSession!= null) {
m_validationSession.set(this, "amount", value, amount);
}
this.amount = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "amount", value, amount);
}
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
@Label(labelName = "name")
@ReadOnly
@Basic
@Column(name = "NAME_", length = 255)
public String getName() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
getMetadata().removeUnknown("name");
if (m_validationSession!= null) {
m_validationSession.set(this, "name", value, name);
}
this.name = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "name", value, name);
}
}
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link String }
*
*/
@Label(labelName = "descr")
@ReadOnly
@Basic
@Column(name = "DESCRIPTION", length = 255)
public String getDescription() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDescription(String value) {
getMetadata().removeUnknown("description");
if (m_validationSession!= null) {
m_validationSession.set(this, "description", value, description);
}
this.description = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "description", value, description);
}
}
/**
* Gets the value of the size property.
*
* @return
* possible object is
* {@link String }
*
*/
@Label(labelName = "Size")
@ChoiceList(name = "Sizes")
@Basic
@Column(name = "SIZE_", length = 20)
public String getSize() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return size;
}
/**
* Sets the value of the size property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSize(String value) {
getMetadata().removeUnknown("size");
if (m_validationSession!= null) {
m_validationSession.set(this, "size", value, size);
}
this.size = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "size", value, size);
}
}
/**
* Gets the value of the base property.
*
* @return
* possible object is
* {@link String }
*
*/
@Label(labelName = "Base")
@ChoiceList(name = "Bases")
@Description(name = "bases.long")
@Basic
@Column(name = "BASE", length = 20)
public String getBase() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return base;
}
/**
* Sets the value of the base property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setBase(String value) {
getMetadata().removeUnknown("base");
if (m_validationSession!= null) {
m_validationSession.set(this, "base", value, base);
}
this.base = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "base", value, base);
}
}
/**
* Gets the value of the topping property.
*
* @return
* possible object is
* {@link String }
*
*/
@Label(labelName = "Topping")
@ChoiceList(name = "Toppings")
@Basic
@Column(name = "TOPPING", length = 20)
public String getTopping() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return topping;
}
/**
* Sets the value of the topping property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTopping(String value) {
getMetadata().removeUnknown("topping");
if (m_validationSession!= null) {
m_validationSession.set(this, "topping", value, topping);
}
this.topping = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "topping", value, topping);
}
}
/**
* Gets the value of the testing property.
*
* @return
* possible object is
* {@link String }
*
*/
@Label(labelName = "testing")
@Inactive
@Basic
@Column(name = "TESTING", length = 20)
public String getTesting() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return testing;
}
/**
* Sets the value of the testing property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTesting(String value) {
getMetadata().removeUnknown("testing");
if (m_validationSession!= null) {
m_validationSession.set(this, "testing", value, testing);
}
this.testing = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "testing", value, testing);
}
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy strategy) {
if (!(object instanceof Pizza)) {
return false;
}
if (this == object) {
return true;
}
final Pizza that = ((Pizza) object);
{
long lhsId;
lhsId = (true?this.getId(): 0L);
long rhsId;
rhsId = (true?that.getId(): 0L);
if (!strategy.equals(LocatorUtils.property(thisLocator, "id", lhsId), LocatorUtils.property(thatLocator, "id", rhsId), lhsId, rhsId)) {
return false;
}
}
{
double lhsAmount;
lhsAmount = (true?this.getAmount(): 0.0D);
double rhsAmount;
rhsAmount = (true?that.getAmount(): 0.0D);
if (!strategy.equals(LocatorUtils.property(thisLocator, "amount", lhsAmount), LocatorUtils.property(thatLocator, "amount", rhsAmount), lhsAmount, rhsAmount)) {
return false;
}
}
{
String lhsName;
lhsName = this.getName();
String rhsName;
rhsName = that.getName();
if (!strategy.equals(LocatorUtils.property(thisLocator, "name", lhsName), LocatorUtils.property(thatLocator, "name", rhsName), lhsName, rhsName)) {
return false;
}
}
{
String lhsDescription;
lhsDescription = this.getDescription();
String rhsDescription;
rhsDescription = that.getDescription();
if (!strategy.equals(LocatorUtils.property(thisLocator, "description", lhsDescription), LocatorUtils.property(thatLocator, "description", rhsDescription), lhsDescription, rhsDescription)) {
return false;
}
}
{
String lhsSize;
lhsSize = this.getSize();
String rhsSize;
rhsSize = that.getSize();
if (!strategy.equals(LocatorUtils.property(thisLocator, "size", lhsSize), LocatorUtils.property(thatLocator, "size", rhsSize), lhsSize, rhsSize)) {
return false;
}
}
{
String lhsBase;
lhsBase = this.getBase();
String rhsBase;
rhsBase = that.getBase();
if (!strategy.equals(LocatorUtils.property(thisLocator, "base", lhsBase), LocatorUtils.property(thatLocator, "base", rhsBase), lhsBase, rhsBase)) {
return false;
}
}
{
String lhsTopping;
lhsTopping = this.getTopping();
String rhsTopping;
rhsTopping = that.getTopping();
if (!strategy.equals(LocatorUtils.property(thisLocator, "topping", lhsTopping), LocatorUtils.property(thatLocator, "topping", rhsTopping), lhsTopping, rhsTopping)) {
return false;
}
}
{
String lhsTesting;
lhsTesting = this.getTesting();
String rhsTesting;
rhsTesting = that.getTesting();
if (!strategy.equals(LocatorUtils.property(thisLocator, "testing", lhsTesting), LocatorUtils.property(thatLocator, "testing", rhsTesting), lhsTesting, rhsTesting)) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
long theId;
theId = (true?this.getId(): 0L);
strategy.appendField(locator, this, "id", buffer, theId);
}
{
double theAmount;
theAmount = (true?this.getAmount(): 0.0D);
strategy.appendField(locator, this, "amount", buffer, theAmount);
}
{
String theName;
theName = this.getName();
strategy.appendField(locator, this, "name", buffer, theName);
}
{
String theDescription;
theDescription = this.getDescription();
strategy.appendField(locator, this, "description", buffer, theDescription);
}
{
String theSize;
theSize = this.getSize();
strategy.appendField(locator, this, "size", buffer, theSize);
}
{
String theBase;
theBase = this.getBase();
strategy.appendField(locator, this, "base", buffer, theBase);
}
{
String theTopping;
theTopping = this.getTopping();
strategy.appendField(locator, this, "topping", buffer, theTopping);
}
{
String theTesting;
theTesting = this.getTesting();
strategy.appendField(locator, this, "testing", buffer, theTesting);
}
return buffer;
}
public int hashCode(ObjectLocator locator, HashCodeStrategy strategy) {
int currentHashCode = 1;
{
long theId;
theId = (true?this.getId(): 0L);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "id", theId), currentHashCode, theId);
}
{
double theAmount;
theAmount = (true?this.getAmount(): 0.0D);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "amount", theAmount), currentHashCode, theAmount);
}
{
String theName;
theName = this.getName();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "name", theName), currentHashCode, theName);
}
{
String theDescription;
theDescription = this.getDescription();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "description", theDescription), currentHashCode, theDescription);
}
{
String theSize;
theSize = this.getSize();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "size", theSize), currentHashCode, theSize);
}
{
String theBase;
theBase = this.getBase();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "base", theBase), currentHashCode, theBase);
}
{
String theTopping;
theTopping = this.getTopping();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "topping", theTopping), currentHashCode, theTopping);
}
{
String theTesting;
theTesting = this.getTesting();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "testing", theTesting), currentHashCode, theTesting);
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
@Transient
public ObjectMetadata getMetadata() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
if (m_metadata == null) {
m_metadata = new ObjectMetadata();
}
return m_metadata;
}
@XmlTransient
public void setValidationSession(ValidationSession validationSession) {
m_validationSession = validationSession;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy