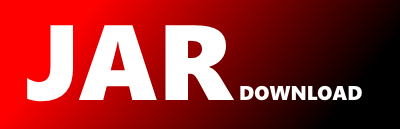
nz.co.senanque.base.Invoice Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.4
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2018.04.01 at 06:43:06 AM UTC
//
package nz.co.senanque.base;
import java.io.Serializable;
import java.util.Date;
import javax.persistence.Basic;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.Inheritance;
import javax.persistence.InheritanceType;
import javax.persistence.Table;
import javax.persistence.Temporal;
import javax.persistence.TemporalType;
import javax.persistence.Transient;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlTransient;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import nz.co.senanque.validationengine.ObjectMetadata;
import nz.co.senanque.validationengine.ValidationObject;
import nz.co.senanque.validationengine.ValidationSession;
import nz.co.senanque.validationengine.ValidationUtils;
import nz.co.senanque.validationengine.annotations.Digits;
import nz.co.senanque.validationengine.annotations.Label;
import nz.co.senanque.validationengine.annotations.Range;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
import org.w3._2001.xmlschema.Adapter2;
/**
* Java class for Invoice complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Invoice">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="id" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="parentid" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="description" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="amount" type="{http://www.w3.org/2001/XMLSchema}double"/>
* <element name="outstanding" type="{http://www.w3.org/2001/XMLSchema}double"/>
* <element name="date" type="{http://www.w3.org/2001/XMLSchema}date"/>
* <element name="revisedDate" type="{http://www.w3.org/2001/XMLSchema}date"/>
* <element name="days" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="testBoolean" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Invoice", propOrder = {
"id",
"parentid",
"description",
"amount",
"outstanding",
"date",
"revisedDate",
"days",
"testBoolean"
})
@Entity(name = "Invoice")
@Table(name = "INVOICE")
@Inheritance(strategy = InheritanceType.JOINED)
public class Invoice
implements Serializable, ValidationObject, Equals, HashCode, ToString
{
@XmlElement(required = true)
protected String id;
@XmlElement(required = true)
protected String parentid;
@XmlElement(required = true)
protected String description;
protected double amount;
protected double outstanding;
@XmlElement(required = true, type = String.class)
@XmlJavaTypeAdapter(Adapter2 .class)
@XmlSchemaType(name = "date")
protected Date date;
@XmlElement(required = true, type = String.class)
@XmlJavaTypeAdapter(Adapter2 .class)
@XmlSchemaType(name = "date")
protected Date revisedDate;
protected long days;
protected boolean testBoolean;
@XmlAttribute(name = "Hjid")
protected Long hjid;
@XmlTransient
protected ValidationSession m_validationSession;
@XmlTransient
protected ObjectMetadata m_metadata;
@XmlTransient
public final static String ID = "id";
@XmlTransient
public final static String PARENTID = "parentid";
@XmlTransient
public final static String DESCRIPTION = "description";
@XmlTransient
public final static String AMOUNT = "amount";
@XmlTransient
public final static String OUTSTANDING = "outstanding";
@XmlTransient
public final static String DATE = "date";
@XmlTransient
public final static String REVISEDDATE = "revisedDate";
@XmlTransient
public final static String DAYS = "days";
@XmlTransient
public final static String TESTBOOLEAN = "testBoolean";
@XmlTransient
public final static String HJID = "hjid";
public Invoice() {
ValidationUtils.setDefaults(this);
}
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
@Basic
@Column(name = "ID", length = 255)
public String getId() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(String value) {
getMetadata().removeUnknown("id");
if (m_validationSession!= null) {
m_validationSession.set(this, "id", value, id);
}
this.id = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "id", value, id);
}
}
/**
* Gets the value of the parentid property.
*
* @return
* possible object is
* {@link String }
*
*/
@Basic
@Column(name = "PARENTID", length = 255)
public String getParentid() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return parentid;
}
/**
* Sets the value of the parentid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setParentid(String value) {
getMetadata().removeUnknown("parentid");
if (m_validationSession!= null) {
m_validationSession.set(this, "parentid", value, parentid);
}
this.parentid = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "parentid", value, parentid);
}
}
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link String }
*
*/
@Label(labelName = "Description")
@Basic
@Column(name = "DESCRIPTION", length = 255)
public String getDescription() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDescription(String value) {
getMetadata().removeUnknown("description");
if (m_validationSession!= null) {
m_validationSession.set(this, "description", value, description);
}
this.description = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "description", value, description);
}
}
/**
* Gets the value of the amount property.
*
*/
@Label(labelName = "Amount")
@Digits(integerDigits = "8", fractionalDigits = "2")
@Range(maxInclusive = "1000", minInclusive = "100")
@Basic
@Column(name = "AMOUNT", precision = 20, scale = 10)
public double getAmount() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return amount;
}
/**
* Sets the value of the amount property.
*
*/
public void setAmount(double value) {
getMetadata().removeUnknown("amount");
if (m_validationSession!= null) {
m_validationSession.set(this, "amount", value, amount);
}
this.amount = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "amount", value, amount);
}
}
/**
* Gets the value of the outstanding property.
*
*/
@Label(labelName = "Outstanding")
@Basic
@Column(name = "OUTSTANDING", precision = 20, scale = 10)
public double getOutstanding() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return outstanding;
}
/**
* Sets the value of the outstanding property.
*
*/
public void setOutstanding(double value) {
getMetadata().removeUnknown("outstanding");
if (m_validationSession!= null) {
m_validationSession.set(this, "outstanding", value, outstanding);
}
this.outstanding = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "outstanding", value, outstanding);
}
}
/**
* Gets the value of the date property.
*
* @return
* possible object is
* {@link String }
*
*/
@Label(labelName = "Date")
@Basic
@Column(name = "DATE_")
@Temporal(TemporalType.DATE)
public Date getDate() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return date;
}
/**
* Sets the value of the date property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDate(Date value) {
getMetadata().removeUnknown("date");
if (m_validationSession!= null) {
m_validationSession.set(this, "date", value, date);
}
this.date = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "date", value, date);
}
}
/**
* Gets the value of the revisedDate property.
*
* @return
* possible object is
* {@link String }
*
*/
@Label(labelName = "revisedDate")
@Basic
@Column(name = "REVISEDDATE")
@Temporal(TemporalType.DATE)
public Date getRevisedDate() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return revisedDate;
}
/**
* Sets the value of the revisedDate property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRevisedDate(Date value) {
getMetadata().removeUnknown("revisedDate");
if (m_validationSession!= null) {
m_validationSession.set(this, "revisedDate", value, revisedDate);
}
this.revisedDate = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "revisedDate", value, revisedDate);
}
}
/**
* Gets the value of the days property.
*
*/
@Label(labelName = "Days")
@Basic
@Column(name = "DAYS", precision = 20, scale = 0)
@Range(maxInclusive = "9223372036854775807", minInclusive = "-9223372036854775808")
public long getDays() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return days;
}
/**
* Sets the value of the days property.
*
*/
public void setDays(long value) {
getMetadata().removeUnknown("days");
if (m_validationSession!= null) {
m_validationSession.set(this, "days", value, days);
}
this.days = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "days", value, days);
}
}
/**
* Gets the value of the testBoolean property.
*
*/
@Label(labelName = "testBoolean")
@Basic
@Column(name = "TESTBOOLEAN")
public boolean isTestBoolean() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return testBoolean;
}
/**
* Sets the value of the testBoolean property.
*
*/
public void setTestBoolean(boolean value) {
getMetadata().removeUnknown("testBoolean");
if (m_validationSession!= null) {
m_validationSession.set(this, "testBoolean", value, testBoolean);
}
this.testBoolean = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "testBoolean", value, testBoolean);
}
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy strategy) {
if (!(object instanceof Invoice)) {
return false;
}
if (this == object) {
return true;
}
final Invoice that = ((Invoice) object);
{
String lhsId;
lhsId = this.getId();
String rhsId;
rhsId = that.getId();
if (!strategy.equals(LocatorUtils.property(thisLocator, "id", lhsId), LocatorUtils.property(thatLocator, "id", rhsId), lhsId, rhsId)) {
return false;
}
}
{
String lhsParentid;
lhsParentid = this.getParentid();
String rhsParentid;
rhsParentid = that.getParentid();
if (!strategy.equals(LocatorUtils.property(thisLocator, "parentid", lhsParentid), LocatorUtils.property(thatLocator, "parentid", rhsParentid), lhsParentid, rhsParentid)) {
return false;
}
}
{
String lhsDescription;
lhsDescription = this.getDescription();
String rhsDescription;
rhsDescription = that.getDescription();
if (!strategy.equals(LocatorUtils.property(thisLocator, "description", lhsDescription), LocatorUtils.property(thatLocator, "description", rhsDescription), lhsDescription, rhsDescription)) {
return false;
}
}
{
double lhsAmount;
lhsAmount = (true?this.getAmount(): 0.0D);
double rhsAmount;
rhsAmount = (true?that.getAmount(): 0.0D);
if (!strategy.equals(LocatorUtils.property(thisLocator, "amount", lhsAmount), LocatorUtils.property(thatLocator, "amount", rhsAmount), lhsAmount, rhsAmount)) {
return false;
}
}
{
double lhsOutstanding;
lhsOutstanding = (true?this.getOutstanding(): 0.0D);
double rhsOutstanding;
rhsOutstanding = (true?that.getOutstanding(): 0.0D);
if (!strategy.equals(LocatorUtils.property(thisLocator, "outstanding", lhsOutstanding), LocatorUtils.property(thatLocator, "outstanding", rhsOutstanding), lhsOutstanding, rhsOutstanding)) {
return false;
}
}
{
Date lhsDate;
lhsDate = this.getDate();
Date rhsDate;
rhsDate = that.getDate();
if (!strategy.equals(LocatorUtils.property(thisLocator, "date", lhsDate), LocatorUtils.property(thatLocator, "date", rhsDate), lhsDate, rhsDate)) {
return false;
}
}
{
Date lhsRevisedDate;
lhsRevisedDate = this.getRevisedDate();
Date rhsRevisedDate;
rhsRevisedDate = that.getRevisedDate();
if (!strategy.equals(LocatorUtils.property(thisLocator, "revisedDate", lhsRevisedDate), LocatorUtils.property(thatLocator, "revisedDate", rhsRevisedDate), lhsRevisedDate, rhsRevisedDate)) {
return false;
}
}
{
long lhsDays;
lhsDays = (true?this.getDays(): 0L);
long rhsDays;
rhsDays = (true?that.getDays(): 0L);
if (!strategy.equals(LocatorUtils.property(thisLocator, "days", lhsDays), LocatorUtils.property(thatLocator, "days", rhsDays), lhsDays, rhsDays)) {
return false;
}
}
{
boolean lhsTestBoolean;
lhsTestBoolean = (true?this.isTestBoolean():false);
boolean rhsTestBoolean;
rhsTestBoolean = (true?that.isTestBoolean():false);
if (!strategy.equals(LocatorUtils.property(thisLocator, "testBoolean", lhsTestBoolean), LocatorUtils.property(thatLocator, "testBoolean", rhsTestBoolean), lhsTestBoolean, rhsTestBoolean)) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
String theId;
theId = this.getId();
strategy.appendField(locator, this, "id", buffer, theId);
}
{
String theParentid;
theParentid = this.getParentid();
strategy.appendField(locator, this, "parentid", buffer, theParentid);
}
{
String theDescription;
theDescription = this.getDescription();
strategy.appendField(locator, this, "description", buffer, theDescription);
}
{
double theAmount;
theAmount = (true?this.getAmount(): 0.0D);
strategy.appendField(locator, this, "amount", buffer, theAmount);
}
{
double theOutstanding;
theOutstanding = (true?this.getOutstanding(): 0.0D);
strategy.appendField(locator, this, "outstanding", buffer, theOutstanding);
}
{
Date theDate;
theDate = this.getDate();
strategy.appendField(locator, this, "date", buffer, theDate);
}
{
Date theRevisedDate;
theRevisedDate = this.getRevisedDate();
strategy.appendField(locator, this, "revisedDate", buffer, theRevisedDate);
}
{
long theDays;
theDays = (true?this.getDays(): 0L);
strategy.appendField(locator, this, "days", buffer, theDays);
}
{
boolean theTestBoolean;
theTestBoolean = (true?this.isTestBoolean():false);
strategy.appendField(locator, this, "testBoolean", buffer, theTestBoolean);
}
return buffer;
}
public int hashCode(ObjectLocator locator, HashCodeStrategy strategy) {
int currentHashCode = 1;
{
String theId;
theId = this.getId();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "id", theId), currentHashCode, theId);
}
{
String theParentid;
theParentid = this.getParentid();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "parentid", theParentid), currentHashCode, theParentid);
}
{
String theDescription;
theDescription = this.getDescription();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "description", theDescription), currentHashCode, theDescription);
}
{
double theAmount;
theAmount = (true?this.getAmount(): 0.0D);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "amount", theAmount), currentHashCode, theAmount);
}
{
double theOutstanding;
theOutstanding = (true?this.getOutstanding(): 0.0D);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "outstanding", theOutstanding), currentHashCode, theOutstanding);
}
{
Date theDate;
theDate = this.getDate();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "date", theDate), currentHashCode, theDate);
}
{
Date theRevisedDate;
theRevisedDate = this.getRevisedDate();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "revisedDate", theRevisedDate), currentHashCode, theRevisedDate);
}
{
long theDays;
theDays = (true?this.getDays(): 0L);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "days", theDays), currentHashCode, theDays);
}
{
boolean theTestBoolean;
theTestBoolean = (true?this.isTestBoolean():false);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "testBoolean", theTestBoolean), currentHashCode, theTestBoolean);
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
/**
* Gets the value of the hjid property.
*
* @return
* possible object is
* {@link Long }
*
*/
@Id
@Column(name = "HJID")
@GeneratedValue(strategy = GenerationType.AUTO)
public Long getHjid() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return hjid;
}
/**
* Sets the value of the hjid property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setHjid(Long value) {
getMetadata().removeUnknown("hjid");
if (m_validationSession!= null) {
m_validationSession.set(this, "hjid", value, hjid);
}
this.hjid = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "hjid", value, hjid);
}
}
@Transient
public ObjectMetadata getMetadata() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
if (m_metadata == null) {
m_metadata = new ObjectMetadata();
}
return m_metadata;
}
@XmlTransient
public void setValidationSession(ValidationSession validationSession) {
m_validationSession = validationSession;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy