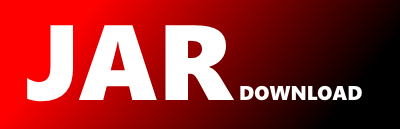
nz.co.senanque.decisiontable.Customer Maven / Gradle / Ivy
Show all versions of madura-rules Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.4
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2016.03.24 at 09:48:53 PM NZDT
//
package nz.co.senanque.decisiontable;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import javax.persistence.Basic;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.Inheritance;
import javax.persistence.InheritanceType;
import javax.persistence.JoinColumn;
import javax.persistence.OneToMany;
import javax.persistence.Table;
import javax.persistence.Transient;
import javax.persistence.Version;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlTransient;
import javax.xml.bind.annotation.XmlType;
import nz.co.senanque.validationengine.ListeningArray;
import nz.co.senanque.validationengine.ObjectMetadata;
import nz.co.senanque.validationengine.ValidationObject;
import nz.co.senanque.validationengine.ValidationSession;
import nz.co.senanque.validationengine.ValidationUtils;
import nz.co.senanque.validationengine.annotations.Email;
import nz.co.senanque.validationengine.annotations.Label;
import nz.co.senanque.validationengine.annotations.Length;
import nz.co.senanque.validationengine.annotations.Range;
import nz.co.senanque.validationengine.annotations.ReadPermission;
import nz.co.senanque.validationengine.annotations.Secret;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
/**
* Java class for Customer complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Customer">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="id" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="version" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="name">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <maxLength value="50"/>
* </restriction>
* </simpleType>
* </element>
* <element name="email">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <maxLength value="50"/>
* </restriction>
* </simpleType>
* </element>
* <element name="password">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <maxLength value="50"/>
* </restriction>
* </simpleType>
* </element>
* <element name="address">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <maxLength value="100"/>
* </restriction>
* </simpleType>
* </element>
* <element name="dynamic" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="preferences" type="{http://www.senanque.co.nz/pizzaorder}Preference" maxOccurs="unbounded" minOccurs="0"/>
* <element name="orders" type="{http://www.senanque.co.nz/pizzaorder}Order" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Customer", propOrder = {
"id",
"version",
"name",
"email",
"password",
"address",
"dynamic",
"preferences",
"orders"
})
@Entity(name = "Customer")
@Table(name = "CUSTOMER")
@Inheritance(strategy = InheritanceType.JOINED)
public class Customer
implements Serializable, ValidationObject, Equals, HashCode, ToString
{
protected long id;
protected long version;
@XmlElement(required = true)
protected String name;
@XmlElement(required = true)
protected String email;
@XmlElement(required = true)
protected String password;
@XmlElement(required = true)
protected String address;
protected boolean dynamic;
protected List preferences;
protected List orders;
@XmlTransient
protected ValidationSession m_validationSession;
@XmlTransient
protected ObjectMetadata m_metadata;
@XmlTransient
public final static String ID = "id";
@XmlTransient
public final static String VERSION = "version";
@XmlTransient
public final static String NAME = "name";
@XmlTransient
public final static String EMAIL = "email";
@XmlTransient
public final static String PASSWORD = "password";
@XmlTransient
public final static String ADDRESS = "address";
@XmlTransient
public final static String DYNAMIC = "dynamic";
@XmlTransient
public final static String PREFERENCES = "preferences";
@XmlTransient
public final static String ORDERS = "orders";
public Customer() {
ValidationUtils.setDefaults(this);
}
/**
* Gets the value of the id property.
*
*/
@Id
@Column(name = "ID", scale = 0)
@Range(maxInclusive = "9223372036854775807", minInclusive = "-9223372036854775808")
public long getId() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return id;
}
/**
* Sets the value of the id property.
*
*/
public void setId(long value) {
getMetadata().removeUnknown("id");
if (m_validationSession!= null) {
m_validationSession.set(this, "id", value, id);
}
this.id = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "id", value, id);
}
}
/**
* Gets the value of the version property.
*
*/
@Version
@Column(name = "VERSION_", scale = 0)
@Range(maxInclusive = "9223372036854775807", minInclusive = "-9223372036854775808")
public long getVersion() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return version;
}
/**
* Sets the value of the version property.
*
*/
public void setVersion(long value) {
getMetadata().removeUnknown("version");
if (m_validationSession!= null) {
m_validationSession.set(this, "version", value, version);
}
this.version = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "version", value, version);
}
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
@Label(labelName = "Name")
@Basic
@Column(name = "NAME_", length = 50)
@Length(minLength = "0", maxLength = "50")
public String getName() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
getMetadata().removeUnknown("name");
if (m_validationSession!= null) {
m_validationSession.set(this, "name", value, name);
}
this.name = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "name", value, name);
}
}
/**
* Gets the value of the email property.
*
* @return
* possible object is
* {@link String }
*
*/
@Label(labelName = "email")
@Email
@Basic
@Column(name = "EMAIL", length = 50)
@Length(minLength = "0", maxLength = "50")
public String getEmail() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return email;
}
/**
* Sets the value of the email property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEmail(String value) {
getMetadata().removeUnknown("email");
if (m_validationSession!= null) {
m_validationSession.set(this, "email", value, email);
}
this.email = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "email", value, email);
}
}
/**
* Gets the value of the password property.
*
* @return
* possible object is
* {@link String }
*
*/
@Label(labelName = "password")
@Secret
@Basic
@Column(name = "PASSWORD_", length = 50)
@Length(minLength = "0", maxLength = "50")
public String getPassword() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return password;
}
/**
* Sets the value of the password property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPassword(String value) {
getMetadata().removeUnknown("password");
if (m_validationSession!= null) {
m_validationSession.set(this, "password", value, password);
}
this.password = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "password", value, password);
}
}
/**
* Gets the value of the address property.
*
* @return
* possible object is
* {@link String }
*
*/
@Label(labelName = "delivery.address")
@ReadPermission(name = "ADMIN")
@Basic
@Column(name = "ADDRESS", length = 100)
@Length(minLength = "0", maxLength = "100")
public String getAddress() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return address;
}
/**
* Sets the value of the address property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAddress(String value) {
getMetadata().removeUnknown("address");
if (m_validationSession!= null) {
m_validationSession.set(this, "address", value, address);
}
this.address = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "address", value, address);
}
}
/**
* Gets the value of the dynamic property.
*
*/
@Label(labelName = "dynamic")
@Basic
@Column(name = "DYNAMIC_")
public boolean isDynamic() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return dynamic;
}
/**
* Sets the value of the dynamic property.
*
*/
public void setDynamic(boolean value) {
getMetadata().removeUnknown("dynamic");
if (m_validationSession!= null) {
m_validationSession.set(this, "dynamic", value, dynamic);
}
this.dynamic = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "dynamic", value, dynamic);
}
}
/**
* Gets the value of the preferences property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the preferences property.
*
*
* For example, to add a new item, do as follows:
*
* getPreferences().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Preference }
*
*
*/
@OneToMany(targetEntity = Preference.class, cascade = {
CascadeType.ALL
})
@JoinColumn(name = "PREFERENCES_CUSTOMER_ID")
public List getPreferences() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
if (preferences == null) {
preferences = new ListeningArray();
}
if (preferences == null) {
preferences = new ArrayList();
}
return this.preferences;
}
/**
*
*
*/
public void setPreferences(List preferences) {
getMetadata().removeUnknown("preferences");
if (m_validationSession!= null) {
m_validationSession.set(this, "preferences", preferences, preferences);
}
this.preferences = preferences;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "preferences", preferences, preferences);
}
}
/**
* Gets the value of the orders property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the orders property.
*
*
* For example, to add a new item, do as follows:
*
* getOrders().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Order }
*
*
*/
@OneToMany(targetEntity = Order.class, cascade = {
CascadeType.ALL
})
@JoinColumn(name = "ORDERS_CUSTOMER_ID")
public List getOrders() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
if (orders == null) {
orders = new ListeningArray();
}
if (orders == null) {
orders = new ArrayList();
}
return this.orders;
}
/**
*
*
*/
public void setOrders(List orders) {
getMetadata().removeUnknown("orders");
if (m_validationSession!= null) {
m_validationSession.set(this, "orders", orders, orders);
}
this.orders = orders;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "orders", orders, orders);
}
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy strategy) {
if (!(object instanceof Customer)) {
return false;
}
if (this == object) {
return true;
}
final Customer that = ((Customer) object);
{
long lhsId;
lhsId = (true?this.getId(): 0L);
long rhsId;
rhsId = (true?that.getId(): 0L);
if (!strategy.equals(LocatorUtils.property(thisLocator, "id", lhsId), LocatorUtils.property(thatLocator, "id", rhsId), lhsId, rhsId)) {
return false;
}
}
{
long lhsVersion;
lhsVersion = (true?this.getVersion(): 0L);
long rhsVersion;
rhsVersion = (true?that.getVersion(): 0L);
if (!strategy.equals(LocatorUtils.property(thisLocator, "version", lhsVersion), LocatorUtils.property(thatLocator, "version", rhsVersion), lhsVersion, rhsVersion)) {
return false;
}
}
{
String lhsName;
lhsName = this.getName();
String rhsName;
rhsName = that.getName();
if (!strategy.equals(LocatorUtils.property(thisLocator, "name", lhsName), LocatorUtils.property(thatLocator, "name", rhsName), lhsName, rhsName)) {
return false;
}
}
{
String lhsEmail;
lhsEmail = this.getEmail();
String rhsEmail;
rhsEmail = that.getEmail();
if (!strategy.equals(LocatorUtils.property(thisLocator, "email", lhsEmail), LocatorUtils.property(thatLocator, "email", rhsEmail), lhsEmail, rhsEmail)) {
return false;
}
}
{
String lhsPassword;
lhsPassword = this.getPassword();
String rhsPassword;
rhsPassword = that.getPassword();
if (!strategy.equals(LocatorUtils.property(thisLocator, "password", lhsPassword), LocatorUtils.property(thatLocator, "password", rhsPassword), lhsPassword, rhsPassword)) {
return false;
}
}
{
String lhsAddress;
lhsAddress = this.getAddress();
String rhsAddress;
rhsAddress = that.getAddress();
if (!strategy.equals(LocatorUtils.property(thisLocator, "address", lhsAddress), LocatorUtils.property(thatLocator, "address", rhsAddress), lhsAddress, rhsAddress)) {
return false;
}
}
{
boolean lhsDynamic;
lhsDynamic = (true?this.isDynamic():false);
boolean rhsDynamic;
rhsDynamic = (true?that.isDynamic():false);
if (!strategy.equals(LocatorUtils.property(thisLocator, "dynamic", lhsDynamic), LocatorUtils.property(thatLocator, "dynamic", rhsDynamic), lhsDynamic, rhsDynamic)) {
return false;
}
}
{
List lhsPreferences;
lhsPreferences = (((this.preferences!= null)&&(!this.preferences.isEmpty()))?this.getPreferences():null);
List rhsPreferences;
rhsPreferences = (((that.preferences!= null)&&(!that.preferences.isEmpty()))?that.getPreferences():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "preferences", lhsPreferences), LocatorUtils.property(thatLocator, "preferences", rhsPreferences), lhsPreferences, rhsPreferences)) {
return false;
}
}
{
List lhsOrders;
lhsOrders = (((this.orders!= null)&&(!this.orders.isEmpty()))?this.getOrders():null);
List rhsOrders;
rhsOrders = (((that.orders!= null)&&(!that.orders.isEmpty()))?that.getOrders():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "orders", lhsOrders), LocatorUtils.property(thatLocator, "orders", rhsOrders), lhsOrders, rhsOrders)) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
long theId;
theId = (true?this.getId(): 0L);
strategy.appendField(locator, this, "id", buffer, theId);
}
{
long theVersion;
theVersion = (true?this.getVersion(): 0L);
strategy.appendField(locator, this, "version", buffer, theVersion);
}
{
String theName;
theName = this.getName();
strategy.appendField(locator, this, "name", buffer, theName);
}
{
String theEmail;
theEmail = this.getEmail();
strategy.appendField(locator, this, "email", buffer, theEmail);
}
{
String thePassword;
thePassword = this.getPassword();
strategy.appendField(locator, this, "password", buffer, thePassword);
}
{
String theAddress;
theAddress = this.getAddress();
strategy.appendField(locator, this, "address", buffer, theAddress);
}
{
boolean theDynamic;
theDynamic = (true?this.isDynamic():false);
strategy.appendField(locator, this, "dynamic", buffer, theDynamic);
}
{
List thePreferences;
thePreferences = (((this.preferences!= null)&&(!this.preferences.isEmpty()))?this.getPreferences():null);
strategy.appendField(locator, this, "preferences", buffer, thePreferences);
}
{
List theOrders;
theOrders = (((this.orders!= null)&&(!this.orders.isEmpty()))?this.getOrders():null);
strategy.appendField(locator, this, "orders", buffer, theOrders);
}
return buffer;
}
public int hashCode(ObjectLocator locator, HashCodeStrategy strategy) {
int currentHashCode = 1;
{
long theId;
theId = (true?this.getId(): 0L);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "id", theId), currentHashCode, theId);
}
{
long theVersion;
theVersion = (true?this.getVersion(): 0L);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "version", theVersion), currentHashCode, theVersion);
}
{
String theName;
theName = this.getName();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "name", theName), currentHashCode, theName);
}
{
String theEmail;
theEmail = this.getEmail();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "email", theEmail), currentHashCode, theEmail);
}
{
String thePassword;
thePassword = this.getPassword();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "password", thePassword), currentHashCode, thePassword);
}
{
String theAddress;
theAddress = this.getAddress();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "address", theAddress), currentHashCode, theAddress);
}
{
boolean theDynamic;
theDynamic = (true?this.isDynamic():false);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "dynamic", theDynamic), currentHashCode, theDynamic);
}
{
List thePreferences;
thePreferences = (((this.preferences!= null)&&(!this.preferences.isEmpty()))?this.getPreferences():null);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "preferences", thePreferences), currentHashCode, thePreferences);
}
{
List theOrders;
theOrders = (((this.orders!= null)&&(!this.orders.isEmpty()))?this.getOrders():null);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "orders", theOrders), currentHashCode, theOrders);
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
@Transient
public ObjectMetadata getMetadata() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
if (m_metadata == null) {
m_metadata = new ObjectMetadata();
}
return m_metadata;
}
@XmlTransient
public void setValidationSession(ValidationSession validationSession) {
m_validationSession = validationSession;
}
}