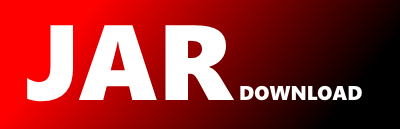
nz.co.senanque.notknown.Customer Maven / Gradle / Ivy
Show all versions of madura-rules Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.4
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2016.03.24 at 09:48:54 PM NZDT
//
package nz.co.senanque.notknown;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import javax.persistence.Basic;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.Inheritance;
import javax.persistence.InheritanceType;
import javax.persistence.JoinColumn;
import javax.persistence.OneToMany;
import javax.persistence.Table;
import javax.persistence.Transient;
import javax.persistence.Version;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlTransient;
import javax.xml.bind.annotation.XmlType;
import nz.co.senanque.validationengine.ListeningArray;
import nz.co.senanque.validationengine.ObjectMetadata;
import nz.co.senanque.validationengine.ValidationObject;
import nz.co.senanque.validationengine.ValidationSession;
import nz.co.senanque.validationengine.ValidationUtils;
import nz.co.senanque.validationengine.annotations.Email;
import nz.co.senanque.validationengine.annotations.Label;
import nz.co.senanque.validationengine.annotations.Length;
import nz.co.senanque.validationengine.annotations.Range;
import nz.co.senanque.validationengine.annotations.ReadPermission;
import nz.co.senanque.validationengine.annotations.Required;
import nz.co.senanque.validationengine.annotations.Secret;
import nz.co.senanque.validationengine.annotations.Unknown;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
/**
* Java class for Customer complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Customer">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="id" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="version" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="name">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <maxLength value="50"/>
* </restriction>
* </simpleType>
* </element>
* <element name="email">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <maxLength value="50"/>
* </restriction>
* </simpleType>
* </element>
* <element name="password">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <maxLength value="50"/>
* </restriction>
* </simpleType>
* </element>
* <element name="address">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <maxLength value="100"/>
* </restriction>
* </simpleType>
* </element>
* <element name="dynamic" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="weight" type="{http://www.w3.org/2001/XMLSchema}double"/>
* <element name="weightKilos" type="{http://www.w3.org/2001/XMLSchema}double"/>
* <element name="weightPounds" type="{http://www.w3.org/2001/XMLSchema}double"/>
* <element name="height" type="{http://www.w3.org/2001/XMLSchema}double"/>
* <element name="heightMetric" type="{http://www.w3.org/2001/XMLSchema}double"/>
* <element name="heightInches" type="{http://www.w3.org/2001/XMLSchema}double"/>
* <element name="heightFeet" type="{http://www.w3.org/2001/XMLSchema}double"/>
* <element name="bmi" type="{http://www.w3.org/2001/XMLSchema}double"/>
* <element name="preferences" type="{http://www.senanque.co.nz/pizzaorder}Preference" maxOccurs="unbounded" minOccurs="0"/>
* <element name="orders" type="{http://www.senanque.co.nz/pizzaorder}Order" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Customer", propOrder = {
"id",
"version",
"name",
"email",
"password",
"address",
"dynamic",
"weight",
"weightKilos",
"weightPounds",
"height",
"heightMetric",
"heightInches",
"heightFeet",
"bmi",
"preferences",
"orders"
})
@Entity(name = "Customer")
@Table(name = "CUSTOMER")
@Inheritance(strategy = InheritanceType.JOINED)
public class Customer
implements Serializable, ValidationObject, Equals, HashCode, ToString
{
protected long id;
protected long version;
@XmlElement(required = true)
protected String name;
@XmlElement(required = true, defaultValue = "[email protected]")
protected String email;
@XmlElement(required = true)
protected String password;
@XmlElement(required = true)
protected String address;
protected boolean dynamic;
protected double weight;
protected double weightKilos;
protected double weightPounds;
protected double height;
protected double heightMetric;
protected double heightInches;
protected double heightFeet;
protected double bmi;
protected List preferences;
protected List orders;
@XmlTransient
protected ValidationSession m_validationSession;
@XmlTransient
protected ObjectMetadata m_metadata;
@XmlTransient
public final static String ID = "id";
@XmlTransient
public final static String VERSION = "version";
@XmlTransient
public final static String NAME = "name";
@XmlTransient
public final static String EMAIL = "email";
@XmlTransient
public final static String PASSWORD = "password";
@XmlTransient
public final static String ADDRESS = "address";
@XmlTransient
public final static String DYNAMIC = "dynamic";
@XmlTransient
public final static String WEIGHT = "weight";
@XmlTransient
public final static String WEIGHTKILOS = "weightKilos";
@XmlTransient
public final static String WEIGHTPOUNDS = "weightPounds";
@XmlTransient
public final static String HEIGHT = "height";
@XmlTransient
public final static String HEIGHTMETRIC = "heightMetric";
@XmlTransient
public final static String HEIGHTINCHES = "heightInches";
@XmlTransient
public final static String HEIGHTFEET = "heightFeet";
@XmlTransient
public final static String BMI = "bmi";
@XmlTransient
public final static String PREFERENCES = "preferences";
@XmlTransient
public final static String ORDERS = "orders";
public Customer() {
ValidationUtils.setDefaults(this);
}
/**
* Gets the value of the id property.
*
*/
@Id
@Column(name = "ID", scale = 0)
@Range(maxInclusive = "9223372036854775807", minInclusive = "-9223372036854775808")
public long getId() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return id;
}
/**
* Sets the value of the id property.
*
*/
public void setId(long value) {
getMetadata().removeUnknown("id");
if (m_validationSession!= null) {
m_validationSession.set(this, "id", value, id);
}
this.id = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "id", value, id);
}
}
/**
* Gets the value of the version property.
*
*/
@Version
@Column(name = "VERSION_", scale = 0)
@Range(maxInclusive = "9223372036854775807", minInclusive = "-9223372036854775808")
public long getVersion() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return version;
}
/**
* Sets the value of the version property.
*
*/
public void setVersion(long value) {
getMetadata().removeUnknown("version");
if (m_validationSession!= null) {
m_validationSession.set(this, "version", value, version);
}
this.version = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "version", value, version);
}
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
@Label(labelName = "Name")
@Basic
@Column(name = "NAME_", length = 50)
@Length(minLength = "0", maxLength = "50")
public String getName() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
getMetadata().removeUnknown("name");
if (m_validationSession!= null) {
m_validationSession.set(this, "name", value, name);
}
this.name = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "name", value, name);
}
}
/**
* Gets the value of the email property.
*
* @return
* possible object is
* {@link String }
*
*/
@Label(labelName = "email")
@Email
@Required
@Basic
@Column(name = "EMAIL", length = 50)
@Length(minLength = "0", maxLength = "50")
public String getEmail() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return email;
}
/**
* Sets the value of the email property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEmail(String value) {
getMetadata().removeUnknown("email");
if (m_validationSession!= null) {
m_validationSession.set(this, "email", value, email);
}
this.email = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "email", value, email);
}
}
/**
* Gets the value of the password property.
*
* @return
* possible object is
* {@link String }
*
*/
@Label(labelName = "password")
@Secret
@Basic
@Column(name = "PASSWORD_", length = 50)
@Length(minLength = "0", maxLength = "50")
public String getPassword() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return password;
}
/**
* Sets the value of the password property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPassword(String value) {
getMetadata().removeUnknown("password");
if (m_validationSession!= null) {
m_validationSession.set(this, "password", value, password);
}
this.password = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "password", value, password);
}
}
/**
* Gets the value of the address property.
*
* @return
* possible object is
* {@link String }
*
*/
@Label(labelName = "delivery.address")
@ReadPermission(name = "ADMIN")
@Unknown
@Basic
@Column(name = "ADDRESS", length = 100)
@Length(minLength = "0", maxLength = "100")
public String getAddress() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return address;
}
/**
* Sets the value of the address property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAddress(String value) {
getMetadata().removeUnknown("address");
if (m_validationSession!= null) {
m_validationSession.set(this, "address", value, address);
}
this.address = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "address", value, address);
}
}
/**
* Gets the value of the dynamic property.
*
*/
@Label(labelName = "dynamic")
@Basic
@Column(name = "DYNAMIC_")
public boolean isDynamic() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return dynamic;
}
/**
* Sets the value of the dynamic property.
*
*/
public void setDynamic(boolean value) {
getMetadata().removeUnknown("dynamic");
if (m_validationSession!= null) {
m_validationSession.set(this, "dynamic", value, dynamic);
}
this.dynamic = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "dynamic", value, dynamic);
}
}
/**
* Gets the value of the weight property.
*
*/
@Label(labelName = "weight")
@Unknown
@Transient
public double getWeight() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return weight;
}
/**
* Sets the value of the weight property.
*
*/
public void setWeight(double value) {
getMetadata().removeUnknown("weight");
if (m_validationSession!= null) {
m_validationSession.set(this, "weight", value, weight);
}
this.weight = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "weight", value, weight);
}
}
/**
* Gets the value of the weightKilos property.
*
*/
@Label(labelName = "weightKilos")
@Unknown
@Basic
@Column(name = "WEIGHTKILOS", precision = 20, scale = 10)
public double getWeightKilos() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return weightKilos;
}
/**
* Sets the value of the weightKilos property.
*
*/
public void setWeightKilos(double value) {
getMetadata().removeUnknown("weightKilos");
if (m_validationSession!= null) {
m_validationSession.set(this, "weightKilos", value, weightKilos);
}
this.weightKilos = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "weightKilos", value, weightKilos);
}
}
/**
* Gets the value of the weightPounds property.
*
*/
@Label(labelName = "weightPounds")
@Unknown
@Basic
@Column(name = "WEIGHTPOUNDS", precision = 20, scale = 10)
public double getWeightPounds() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return weightPounds;
}
/**
* Sets the value of the weightPounds property.
*
*/
public void setWeightPounds(double value) {
getMetadata().removeUnknown("weightPounds");
if (m_validationSession!= null) {
m_validationSession.set(this, "weightPounds", value, weightPounds);
}
this.weightPounds = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "weightPounds", value, weightPounds);
}
}
/**
* Gets the value of the height property.
*
*/
@Label(labelName = "height")
@Unknown
@Transient
public double getHeight() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return height;
}
/**
* Sets the value of the height property.
*
*/
public void setHeight(double value) {
getMetadata().removeUnknown("height");
if (m_validationSession!= null) {
m_validationSession.set(this, "height", value, height);
}
this.height = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "height", value, height);
}
}
/**
* Gets the value of the heightMetric property.
*
*/
@Label(labelName = "heightMetric")
@Unknown
@Basic
@Column(name = "HEIGHTMETRIC", precision = 20, scale = 10)
public double getHeightMetric() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return heightMetric;
}
/**
* Sets the value of the heightMetric property.
*
*/
public void setHeightMetric(double value) {
getMetadata().removeUnknown("heightMetric");
if (m_validationSession!= null) {
m_validationSession.set(this, "heightMetric", value, heightMetric);
}
this.heightMetric = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "heightMetric", value, heightMetric);
}
}
/**
* Gets the value of the heightInches property.
*
*/
@Label(labelName = "heightInches")
@Unknown
@Basic
@Column(name = "HEIGHTINCHES", precision = 20, scale = 10)
public double getHeightInches() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return heightInches;
}
/**
* Sets the value of the heightInches property.
*
*/
public void setHeightInches(double value) {
getMetadata().removeUnknown("heightInches");
if (m_validationSession!= null) {
m_validationSession.set(this, "heightInches", value, heightInches);
}
this.heightInches = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "heightInches", value, heightInches);
}
}
/**
* Gets the value of the heightFeet property.
*
*/
@Label(labelName = "heightFeet")
@Unknown
@Basic
@Column(name = "HEIGHTFEET", precision = 20, scale = 10)
public double getHeightFeet() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return heightFeet;
}
/**
* Sets the value of the heightFeet property.
*
*/
public void setHeightFeet(double value) {
getMetadata().removeUnknown("heightFeet");
if (m_validationSession!= null) {
m_validationSession.set(this, "heightFeet", value, heightFeet);
}
this.heightFeet = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "heightFeet", value, heightFeet);
}
}
/**
* Gets the value of the bmi property.
*
*/
@Label(labelName = "BMI")
@Unknown
@Basic
@Column(name = "BMI", precision = 20, scale = 10)
public double getBmi() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return bmi;
}
/**
* Sets the value of the bmi property.
*
*/
public void setBmi(double value) {
getMetadata().removeUnknown("bmi");
if (m_validationSession!= null) {
m_validationSession.set(this, "bmi", value, bmi);
}
this.bmi = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "bmi", value, bmi);
}
}
/**
* Gets the value of the preferences property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the preferences property.
*
*
* For example, to add a new item, do as follows:
*
* getPreferences().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Preference }
*
*
*/
@OneToMany(targetEntity = Preference.class, cascade = {
CascadeType.ALL
})
@JoinColumn(name = "PREFERENCES_CUSTOMER_ID")
public List getPreferences() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
if (preferences == null) {
preferences = new ListeningArray();
}
if (preferences == null) {
preferences = new ArrayList();
}
return this.preferences;
}
/**
*
*
*/
public void setPreferences(List preferences) {
getMetadata().removeUnknown("preferences");
if (m_validationSession!= null) {
m_validationSession.set(this, "preferences", preferences, preferences);
}
this.preferences = preferences;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "preferences", preferences, preferences);
}
}
/**
* Gets the value of the orders property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the orders property.
*
*
* For example, to add a new item, do as follows:
*
* getOrders().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Order }
*
*
*/
@OneToMany(targetEntity = Order.class, cascade = {
CascadeType.ALL
})
@JoinColumn(name = "ORDERS_CUSTOMER_ID")
public List getOrders() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
if (orders == null) {
orders = new ListeningArray();
}
if (orders == null) {
orders = new ArrayList();
}
return this.orders;
}
/**
*
*
*/
public void setOrders(List orders) {
getMetadata().removeUnknown("orders");
if (m_validationSession!= null) {
m_validationSession.set(this, "orders", orders, orders);
}
this.orders = orders;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "orders", orders, orders);
}
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy strategy) {
if (!(object instanceof Customer)) {
return false;
}
if (this == object) {
return true;
}
final Customer that = ((Customer) object);
{
long lhsId;
lhsId = (true?this.getId(): 0L);
long rhsId;
rhsId = (true?that.getId(): 0L);
if (!strategy.equals(LocatorUtils.property(thisLocator, "id", lhsId), LocatorUtils.property(thatLocator, "id", rhsId), lhsId, rhsId)) {
return false;
}
}
{
long lhsVersion;
lhsVersion = (true?this.getVersion(): 0L);
long rhsVersion;
rhsVersion = (true?that.getVersion(): 0L);
if (!strategy.equals(LocatorUtils.property(thisLocator, "version", lhsVersion), LocatorUtils.property(thatLocator, "version", rhsVersion), lhsVersion, rhsVersion)) {
return false;
}
}
{
String lhsName;
lhsName = this.getName();
String rhsName;
rhsName = that.getName();
if (!strategy.equals(LocatorUtils.property(thisLocator, "name", lhsName), LocatorUtils.property(thatLocator, "name", rhsName), lhsName, rhsName)) {
return false;
}
}
{
String lhsEmail;
lhsEmail = this.getEmail();
String rhsEmail;
rhsEmail = that.getEmail();
if (!strategy.equals(LocatorUtils.property(thisLocator, "email", lhsEmail), LocatorUtils.property(thatLocator, "email", rhsEmail), lhsEmail, rhsEmail)) {
return false;
}
}
{
String lhsPassword;
lhsPassword = this.getPassword();
String rhsPassword;
rhsPassword = that.getPassword();
if (!strategy.equals(LocatorUtils.property(thisLocator, "password", lhsPassword), LocatorUtils.property(thatLocator, "password", rhsPassword), lhsPassword, rhsPassword)) {
return false;
}
}
{
String lhsAddress;
lhsAddress = this.getAddress();
String rhsAddress;
rhsAddress = that.getAddress();
if (!strategy.equals(LocatorUtils.property(thisLocator, "address", lhsAddress), LocatorUtils.property(thatLocator, "address", rhsAddress), lhsAddress, rhsAddress)) {
return false;
}
}
{
boolean lhsDynamic;
lhsDynamic = (true?this.isDynamic():false);
boolean rhsDynamic;
rhsDynamic = (true?that.isDynamic():false);
if (!strategy.equals(LocatorUtils.property(thisLocator, "dynamic", lhsDynamic), LocatorUtils.property(thatLocator, "dynamic", rhsDynamic), lhsDynamic, rhsDynamic)) {
return false;
}
}
{
double lhsWeight;
lhsWeight = (true?this.getWeight(): 0.0D);
double rhsWeight;
rhsWeight = (true?that.getWeight(): 0.0D);
if (!strategy.equals(LocatorUtils.property(thisLocator, "weight", lhsWeight), LocatorUtils.property(thatLocator, "weight", rhsWeight), lhsWeight, rhsWeight)) {
return false;
}
}
{
double lhsWeightKilos;
lhsWeightKilos = (true?this.getWeightKilos(): 0.0D);
double rhsWeightKilos;
rhsWeightKilos = (true?that.getWeightKilos(): 0.0D);
if (!strategy.equals(LocatorUtils.property(thisLocator, "weightKilos", lhsWeightKilos), LocatorUtils.property(thatLocator, "weightKilos", rhsWeightKilos), lhsWeightKilos, rhsWeightKilos)) {
return false;
}
}
{
double lhsWeightPounds;
lhsWeightPounds = (true?this.getWeightPounds(): 0.0D);
double rhsWeightPounds;
rhsWeightPounds = (true?that.getWeightPounds(): 0.0D);
if (!strategy.equals(LocatorUtils.property(thisLocator, "weightPounds", lhsWeightPounds), LocatorUtils.property(thatLocator, "weightPounds", rhsWeightPounds), lhsWeightPounds, rhsWeightPounds)) {
return false;
}
}
{
double lhsHeight;
lhsHeight = (true?this.getHeight(): 0.0D);
double rhsHeight;
rhsHeight = (true?that.getHeight(): 0.0D);
if (!strategy.equals(LocatorUtils.property(thisLocator, "height", lhsHeight), LocatorUtils.property(thatLocator, "height", rhsHeight), lhsHeight, rhsHeight)) {
return false;
}
}
{
double lhsHeightMetric;
lhsHeightMetric = (true?this.getHeightMetric(): 0.0D);
double rhsHeightMetric;
rhsHeightMetric = (true?that.getHeightMetric(): 0.0D);
if (!strategy.equals(LocatorUtils.property(thisLocator, "heightMetric", lhsHeightMetric), LocatorUtils.property(thatLocator, "heightMetric", rhsHeightMetric), lhsHeightMetric, rhsHeightMetric)) {
return false;
}
}
{
double lhsHeightInches;
lhsHeightInches = (true?this.getHeightInches(): 0.0D);
double rhsHeightInches;
rhsHeightInches = (true?that.getHeightInches(): 0.0D);
if (!strategy.equals(LocatorUtils.property(thisLocator, "heightInches", lhsHeightInches), LocatorUtils.property(thatLocator, "heightInches", rhsHeightInches), lhsHeightInches, rhsHeightInches)) {
return false;
}
}
{
double lhsHeightFeet;
lhsHeightFeet = (true?this.getHeightFeet(): 0.0D);
double rhsHeightFeet;
rhsHeightFeet = (true?that.getHeightFeet(): 0.0D);
if (!strategy.equals(LocatorUtils.property(thisLocator, "heightFeet", lhsHeightFeet), LocatorUtils.property(thatLocator, "heightFeet", rhsHeightFeet), lhsHeightFeet, rhsHeightFeet)) {
return false;
}
}
{
double lhsBmi;
lhsBmi = (true?this.getBmi(): 0.0D);
double rhsBmi;
rhsBmi = (true?that.getBmi(): 0.0D);
if (!strategy.equals(LocatorUtils.property(thisLocator, "bmi", lhsBmi), LocatorUtils.property(thatLocator, "bmi", rhsBmi), lhsBmi, rhsBmi)) {
return false;
}
}
{
List lhsPreferences;
lhsPreferences = (((this.preferences!= null)&&(!this.preferences.isEmpty()))?this.getPreferences():null);
List rhsPreferences;
rhsPreferences = (((that.preferences!= null)&&(!that.preferences.isEmpty()))?that.getPreferences():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "preferences", lhsPreferences), LocatorUtils.property(thatLocator, "preferences", rhsPreferences), lhsPreferences, rhsPreferences)) {
return false;
}
}
{
List lhsOrders;
lhsOrders = (((this.orders!= null)&&(!this.orders.isEmpty()))?this.getOrders():null);
List rhsOrders;
rhsOrders = (((that.orders!= null)&&(!that.orders.isEmpty()))?that.getOrders():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "orders", lhsOrders), LocatorUtils.property(thatLocator, "orders", rhsOrders), lhsOrders, rhsOrders)) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
long theId;
theId = (true?this.getId(): 0L);
strategy.appendField(locator, this, "id", buffer, theId);
}
{
long theVersion;
theVersion = (true?this.getVersion(): 0L);
strategy.appendField(locator, this, "version", buffer, theVersion);
}
{
String theName;
theName = this.getName();
strategy.appendField(locator, this, "name", buffer, theName);
}
{
String theEmail;
theEmail = this.getEmail();
strategy.appendField(locator, this, "email", buffer, theEmail);
}
{
String thePassword;
thePassword = this.getPassword();
strategy.appendField(locator, this, "password", buffer, thePassword);
}
{
String theAddress;
theAddress = this.getAddress();
strategy.appendField(locator, this, "address", buffer, theAddress);
}
{
boolean theDynamic;
theDynamic = (true?this.isDynamic():false);
strategy.appendField(locator, this, "dynamic", buffer, theDynamic);
}
{
double theWeight;
theWeight = (true?this.getWeight(): 0.0D);
strategy.appendField(locator, this, "weight", buffer, theWeight);
}
{
double theWeightKilos;
theWeightKilos = (true?this.getWeightKilos(): 0.0D);
strategy.appendField(locator, this, "weightKilos", buffer, theWeightKilos);
}
{
double theWeightPounds;
theWeightPounds = (true?this.getWeightPounds(): 0.0D);
strategy.appendField(locator, this, "weightPounds", buffer, theWeightPounds);
}
{
double theHeight;
theHeight = (true?this.getHeight(): 0.0D);
strategy.appendField(locator, this, "height", buffer, theHeight);
}
{
double theHeightMetric;
theHeightMetric = (true?this.getHeightMetric(): 0.0D);
strategy.appendField(locator, this, "heightMetric", buffer, theHeightMetric);
}
{
double theHeightInches;
theHeightInches = (true?this.getHeightInches(): 0.0D);
strategy.appendField(locator, this, "heightInches", buffer, theHeightInches);
}
{
double theHeightFeet;
theHeightFeet = (true?this.getHeightFeet(): 0.0D);
strategy.appendField(locator, this, "heightFeet", buffer, theHeightFeet);
}
{
double theBmi;
theBmi = (true?this.getBmi(): 0.0D);
strategy.appendField(locator, this, "bmi", buffer, theBmi);
}
{
List thePreferences;
thePreferences = (((this.preferences!= null)&&(!this.preferences.isEmpty()))?this.getPreferences():null);
strategy.appendField(locator, this, "preferences", buffer, thePreferences);
}
{
List theOrders;
theOrders = (((this.orders!= null)&&(!this.orders.isEmpty()))?this.getOrders():null);
strategy.appendField(locator, this, "orders", buffer, theOrders);
}
return buffer;
}
public int hashCode(ObjectLocator locator, HashCodeStrategy strategy) {
int currentHashCode = 1;
{
long theId;
theId = (true?this.getId(): 0L);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "id", theId), currentHashCode, theId);
}
{
long theVersion;
theVersion = (true?this.getVersion(): 0L);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "version", theVersion), currentHashCode, theVersion);
}
{
String theName;
theName = this.getName();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "name", theName), currentHashCode, theName);
}
{
String theEmail;
theEmail = this.getEmail();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "email", theEmail), currentHashCode, theEmail);
}
{
String thePassword;
thePassword = this.getPassword();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "password", thePassword), currentHashCode, thePassword);
}
{
String theAddress;
theAddress = this.getAddress();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "address", theAddress), currentHashCode, theAddress);
}
{
boolean theDynamic;
theDynamic = (true?this.isDynamic():false);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "dynamic", theDynamic), currentHashCode, theDynamic);
}
{
double theWeight;
theWeight = (true?this.getWeight(): 0.0D);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "weight", theWeight), currentHashCode, theWeight);
}
{
double theWeightKilos;
theWeightKilos = (true?this.getWeightKilos(): 0.0D);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "weightKilos", theWeightKilos), currentHashCode, theWeightKilos);
}
{
double theWeightPounds;
theWeightPounds = (true?this.getWeightPounds(): 0.0D);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "weightPounds", theWeightPounds), currentHashCode, theWeightPounds);
}
{
double theHeight;
theHeight = (true?this.getHeight(): 0.0D);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "height", theHeight), currentHashCode, theHeight);
}
{
double theHeightMetric;
theHeightMetric = (true?this.getHeightMetric(): 0.0D);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "heightMetric", theHeightMetric), currentHashCode, theHeightMetric);
}
{
double theHeightInches;
theHeightInches = (true?this.getHeightInches(): 0.0D);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "heightInches", theHeightInches), currentHashCode, theHeightInches);
}
{
double theHeightFeet;
theHeightFeet = (true?this.getHeightFeet(): 0.0D);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "heightFeet", theHeightFeet), currentHashCode, theHeightFeet);
}
{
double theBmi;
theBmi = (true?this.getBmi(): 0.0D);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "bmi", theBmi), currentHashCode, theBmi);
}
{
List thePreferences;
thePreferences = (((this.preferences!= null)&&(!this.preferences.isEmpty()))?this.getPreferences():null);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "preferences", thePreferences), currentHashCode, thePreferences);
}
{
List theOrders;
theOrders = (((this.orders!= null)&&(!this.orders.isEmpty()))?this.getOrders():null);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "orders", theOrders), currentHashCode, theOrders);
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
@Transient
public ObjectMetadata getMetadata() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
if (m_metadata == null) {
m_metadata = new ObjectMetadata();
}
return m_metadata;
}
@XmlTransient
public void setValidationSession(ValidationSession validationSession) {
m_validationSession = validationSession;
}
}