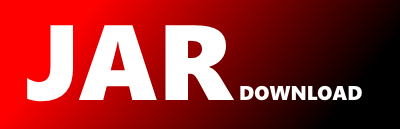
nz.co.senanque.pizzaorder.Preference Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of madura-rules Show documentation
Show all versions of madura-rules Show documentation
This is a plugin to Madura Objects. It provides a rules/constraint engine to assist with validation,
deriving new values from user inputs (eg total of invoices entered on this customer) an manipulating metadata
(eg because the amount is above X we make some field readonly). Note that the Java that is using the
monitored objects is quite unaware of the rules layer implemented here. That means you can change rules
without having to go back to your Java code, and it also means you don't have to wonder if everything
implemented the same rules. Anything using that Java object has the rules (unless you turn them all off).
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.4
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2016.03.24 at 09:48:53 PM NZDT
//
package nz.co.senanque.pizzaorder;
import java.io.Serializable;
import javax.persistence.Basic;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.EnumType;
import javax.persistence.Enumerated;
import javax.persistence.Id;
import javax.persistence.Inheritance;
import javax.persistence.InheritanceType;
import javax.persistence.Table;
import javax.persistence.Transient;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlTransient;
import javax.xml.bind.annotation.XmlType;
import nz.co.senanque.validationengine.ObjectMetadata;
import nz.co.senanque.validationengine.ValidationObject;
import nz.co.senanque.validationengine.ValidationSession;
import nz.co.senanque.validationengine.ValidationUtils;
import nz.co.senanque.validationengine.annotations.Range;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
/**
* Java class for Preference complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Preference">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="id" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="parentid" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="type" type="{http://www.senanque.co.nz/pizzaorder}PreferenceType"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Preference", propOrder = {
"id",
"parentid",
"type"
})
@Entity(name = "Preference")
@Table(name = "PREFERENCE")
@Inheritance(strategy = InheritanceType.JOINED)
public class Preference
implements Serializable, ValidationObject, Equals, HashCode, ToString
{
protected long id;
protected long parentid;
@XmlElement(required = true)
protected PreferenceType type;
@XmlTransient
protected ValidationSession m_validationSession;
@XmlTransient
protected ObjectMetadata m_metadata;
@XmlTransient
public final static String ID = "id";
@XmlTransient
public final static String PARENTID = "parentid";
@XmlTransient
public final static String TYPE = "type";
public Preference() {
ValidationUtils.setDefaults(this);
}
/**
* Gets the value of the id property.
*
*/
@Id
@Column(name = "ID", scale = 0)
@Range(maxInclusive = "9223372036854775807", minInclusive = "-9223372036854775808")
public long getId() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return id;
}
/**
* Sets the value of the id property.
*
*/
public void setId(long value) {
getMetadata().removeUnknown("id");
if (m_validationSession!= null) {
m_validationSession.set(this, "id", value, id);
}
this.id = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "id", value, id);
}
}
/**
* Gets the value of the parentid property.
*
*/
@Basic
@Column(name = "PARENTID", precision = 20, scale = 0)
@Range(maxInclusive = "9223372036854775807", minInclusive = "-9223372036854775808")
public long getParentid() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return parentid;
}
/**
* Sets the value of the parentid property.
*
*/
public void setParentid(long value) {
getMetadata().removeUnknown("parentid");
if (m_validationSession!= null) {
m_validationSession.set(this, "parentid", value, parentid);
}
this.parentid = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "parentid", value, parentid);
}
}
/**
* Gets the value of the type property.
*
* @return
* possible object is
* {@link PreferenceType }
*
*/
@Basic
@Column(name = "TYPE_", length = 255)
@Enumerated(EnumType.STRING)
public PreferenceType getType() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
return type;
}
/**
* Sets the value of the type property.
*
* @param value
* allowed object is
* {@link PreferenceType }
*
*/
public void setType(PreferenceType value) {
getMetadata().removeUnknown("type");
if (m_validationSession!= null) {
m_validationSession.set(this, "type", value, type);
}
this.type = value;
if (m_validationSession!= null) {
m_validationSession.invokeListeners(this, "type", value, type);
}
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy strategy) {
if (!(object instanceof Preference)) {
return false;
}
if (this == object) {
return true;
}
final Preference that = ((Preference) object);
{
long lhsId;
lhsId = (true?this.getId(): 0L);
long rhsId;
rhsId = (true?that.getId(): 0L);
if (!strategy.equals(LocatorUtils.property(thisLocator, "id", lhsId), LocatorUtils.property(thatLocator, "id", rhsId), lhsId, rhsId)) {
return false;
}
}
{
long lhsParentid;
lhsParentid = (true?this.getParentid(): 0L);
long rhsParentid;
rhsParentid = (true?that.getParentid(): 0L);
if (!strategy.equals(LocatorUtils.property(thisLocator, "parentid", lhsParentid), LocatorUtils.property(thatLocator, "parentid", rhsParentid), lhsParentid, rhsParentid)) {
return false;
}
}
{
PreferenceType lhsType;
lhsType = this.getType();
PreferenceType rhsType;
rhsType = that.getType();
if (!strategy.equals(LocatorUtils.property(thisLocator, "type", lhsType), LocatorUtils.property(thatLocator, "type", rhsType), lhsType, rhsType)) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
long theId;
theId = (true?this.getId(): 0L);
strategy.appendField(locator, this, "id", buffer, theId);
}
{
long theParentid;
theParentid = (true?this.getParentid(): 0L);
strategy.appendField(locator, this, "parentid", buffer, theParentid);
}
{
PreferenceType theType;
theType = this.getType();
strategy.appendField(locator, this, "type", buffer, theType);
}
return buffer;
}
public int hashCode(ObjectLocator locator, HashCodeStrategy strategy) {
int currentHashCode = 1;
{
long theId;
theId = (true?this.getId(): 0L);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "id", theId), currentHashCode, theId);
}
{
long theParentid;
theParentid = (true?this.getParentid(): 0L);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "parentid", theParentid), currentHashCode, theParentid);
}
{
PreferenceType theType;
theType = this.getType();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "type", theType), currentHashCode, theType);
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
@Transient
public ObjectMetadata getMetadata() {
if (m_validationSession!= null) {
m_validationSession.clean(this);
}
if (m_metadata == null) {
m_metadata = new ObjectMetadata();
}
return m_metadata;
}
@XmlTransient
public void setValidationSession(ValidationSession validationSession) {
m_validationSession = validationSession;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy