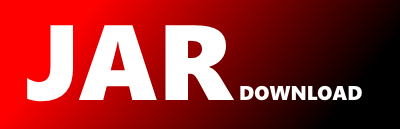
nz.co.senanque.vaadin.converter.StringToChoiceBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of madura-vaadin Show documentation
Show all versions of madura-vaadin Show documentation
Integrates Madura Objects with Vaadin applications including support for dynamic metadata changes (available choices, read/write status of fields etc).
package nz.co.senanque.vaadin.converter;
import java.util.Locale;
import nz.co.senanque.vaadin.MaduraPropertyWrapper;
import nz.co.senanque.validationengine.choicelists.ChoiceBase;
import com.vaadin.data.util.converter.Converter;
/**
* Allows Vaadin to convert between a ChoiceBase and a String.
* @author Roger Parkinson
*
*/
public class StringToChoiceBase implements Converter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy