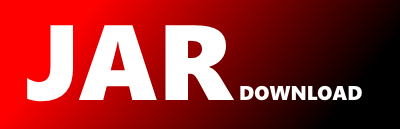
com.sun.syndication.feed.atom.Feed Maven / Gradle / Ivy
/*
* Copyright 2004 Sun Microsystems, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package com.sun.syndication.feed.atom;
import com.sun.syndication.feed.WireFeed;
import com.sun.syndication.feed.module.Module;
import com.sun.syndication.feed.module.impl.ModuleUtils;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
/**
* Bean for Atom feeds.
*
* It handles Atom feeds version 0.3 without loosing any feed information.
*
* @author Alejandro Abdelnur
* @author Dave Johnson (updated for Atom 1.0)
*/
public class Feed extends WireFeed {
private String _xmlBase;
private List _categories;
private List _authors;
private List _contributors;
private Generator _generator;
private String _icon;
private String _id;
private String _logo;
private String _rights; // AKA copyright
private Content _subtitle; // AKA tagline
private Content _title;
private Date _updated; // AKA modified
private List _alternateLinks;
private List _otherLinks;
private List _entries;
private List _modules;
private Content _info; // Atom 0.3 only
private String _language; // Atom 0.3 only
/**
* Default constructor, for bean cloning purposes only.
*
*/
public Feed() {
}
/**
* Feed Constructor. All properties, except the type, are set to null.
*
* @param type the type of the Atom feed.
*
*/
public Feed(String type) {
super(type);
}
/**
* Returns the feed language (Atom 0.3 only)
*
* @return the feed language, null if none.
*
*/
public String getLanguage() {
return _language;
}
/**
* Sets the feed language (Atom 0.3 only)
*
* @param language the feed language to set, null if none.
*
*/
public void setLanguage(String language) {
_language = language;
}
/**
* Returns the feed title.
*
* @return the feed title, null if none.
*
*/
public String getTitle() {
if (_title != null) return _title.getValue();
return null;
}
/**
* Sets the feed title.
*
* @param title the feed title to set, null if none.
*
*/
public void setTitle(String title) {
if (_title == null) _title = new Content();
_title.setValue(title);
}
/**
* Returns the feed title.
*
* @return the feed title, null if none.
*
*/
public Content getTitleEx() {
return _title;
}
/**
* Sets the feed title.
*
* @param title the feed title to set, null if none.
*
*/
public void setTitleEx(Content title) {
_title = title;
}
/**
* Returns the feed alternate links.
*
* @return a list of Link elements with the feed alternate links,
* an empty list if none.
*/
public List getAlternateLinks() {
return (_alternateLinks==null) ? (_alternateLinks=new ArrayList()) : _alternateLinks;
}
/**
* Sets the feed alternate links.
*
* @param alternateLinks the list of Link elements with the feed alternate links to set,
* an empty list or null if none.
*/
public void setAlternateLinks(List alternateLinks) {
_alternateLinks = alternateLinks;
}
/**
* Returns the feed other links (non-alternate ones).
*
* @return a list of Link elements with the feed other links (non-alternate ones),
* an empty list if none.
*/
public List getOtherLinks() {
return (_otherLinks==null) ? (_otherLinks=new ArrayList()) : _otherLinks;
}
/**
* Sets the feed other links (non-alternate ones).
*
* @param otherLinks the list of Link elements with the feed other links (non-alternate ones) to set,
* an empty list or null if none.
*/
public void setOtherLinks(List otherLinks) {
_otherLinks = otherLinks;
}
/**
* Returns the feed author.
*
* @return the feed author, null if none.
*
*/
public List getAuthors() {
return (_authors==null) ? (_authors=new ArrayList()) : _authors;
}
/**
* Sets the feed author.
*
* @param authors the feed author to set, null if none.
*
*/
public void setAuthors(List authors) {
_authors = authors;
}
/**
* Returns the feed contributors.
*
* @return a list of Person elements with the feed contributors,
* an empty list if none.
*
*/
public List getContributors() {
return (_contributors==null) ? (_contributors=new ArrayList()) : _contributors;
}
/**
* Sets the feed contributors.
*
* @param contributors the list of Person elements with the feed contributors to set,
* an empty list or null if none.
*
*/
public void setContributors(List contributors) {
_contributors = contributors;
}
/**
* Returns the feed tag line (Atom 0.3, maps to {@link #getSubtitle()}).
*
* @return the feed tag line, null if none.
*/
public Content getTagline() {
return _subtitle;
}
/**
* Sets the feed tagline (Atom 0.3, maps to {@link #setSubtitle(com.sun.syndication.feed.atom.Content)}).
*
* @param tagline the feed tagline to set, null if none.
*/
public void setTagline(Content tagline) {
_subtitle = tagline;
}
/**
* Returns the feed ID.
*
* @return the feed ID, null if none.
*
*/
public String getId() {
return _id;
}
/**
* Sets the feed ID.
*
* @param id the feed ID to set, null if none.
*
*/
public void setId(String id) {
_id = id;
}
/**
* Returns the feed generator.
*
* @return the feed generator, null if none.
*
*/
public Generator getGenerator() {
return _generator;
}
/**
* Sets the feed generator.
*
* @param generator the feed generator to set, null if none.
*
*/
public void setGenerator(Generator generator) {
_generator = generator;
}
/**
* Returns the feed copyright (Atom 0.3, maps to {@link #getRights()}).
*
* @return the feed copyright, null if none.
*/
public String getCopyright() {
return _rights;
}
/**
* Sets the feed copyright (Atom 0.3, maps to {@link #setRights(java.lang.String)}).
*
* @param copyright the feed copyright to set, null if none.
*/
public void setCopyright(String copyright) {
_rights = copyright;
}
/**
* Returns the feed info (Atom 0.3 only)
*
* @return the feed info, null if none.
*/
public Content getInfo() {
return _info;
}
/**
* Sets the feed info (Atom 0.3 only)
*
* @param info the feed info to set, null if none.
*/
public void setInfo(Content info) {
_info = info;
}
/**
* Returns the feed modified date (Atom 0.3, maps to {@link #getUpdated()}).
*
* @return the feed modified date, null if none.
*/
public Date getModified() {
return _updated;
}
/**
* Sets the feed modified date (Atom 0.3, maps to {@link #setUpdated(java.util.Date)}).
*
* @param modified the feed modified date to set, null if none.
*/
public void setModified(Date modified) {
_updated = modified;
}
/**
* Returns the feed entries.
*
* @return a list of Entry elements with the feed entries,
* an empty list if none.
*
*/
public List getEntries() {
return (_entries==null) ? (_entries=new ArrayList()) : _entries;
}
/**
* Sets the feed entries.
*
* @param entries the list of Entry elements with the feed entries to set,
* an empty list or null if none.
*
*/
public void setEntries(List entries) {
_entries = entries;
}
/**
* Returns the feed modules.
*
* @return a list of ModuleImpl elements with the feed modules,
* an empty list if none.
*
*/
public List getModules() {
return (_modules==null) ? (_modules=new ArrayList()) : _modules;
}
/**
* Sets the feed moduless.
*
* @param modules the list of ModuleImpl elements with the feed moduless to set,
* an empty list or null if none.
*
*/
@Override
public void setModules(List modules) {
_modules = modules;
}
/**
* Returns the module identified by a given URI.
*
* @param uri the URI of the ModuleImpl.
* @return The module with the given URI, null if none.
*/
@Override
public Module getModule(String uri) {
return ModuleUtils.getModule(_modules,uri);
}
/**
* Returns the categories
*
* @return Returns the categories.
* @since Atom 1.0
*/
public List getCategories() {
return (_categories==null) ? (_categories=new ArrayList()) : _categories;
}
/**
* Set the categories
*
* @param categories The categories to set.
* @since Atom 1.0
*/
public void setCategories(List categories) {
_categories = categories;
}
/**
* Returns the icon
*
* @return Returns the icon.
* @since Atom 1.0
*/
public String getIcon() {
return _icon;
}
/**
* Set the icon
*
* @param icon The icon to set.
* @since Atom 1.0
*/
public void setIcon(String icon) {
_icon = icon;
}
/**
* Returns the logo
*
* @return Returns the logo.
* @since Atom 1.0
*/
public String getLogo() {
return _logo;
}
/**
* Set the logo
*
* @param logo The logo to set.
* @since Atom 1.0
*/
public void setLogo(String logo) {
_logo = logo;
}
/**
* Returns the rights
*
* @return Returns the rights.
* @since Atom 1.0
*/
public String getRights() {
return _rights;
}
/**
* Set the rights
*
* @param rights The rights to set.
* @since Atom 1.0
*/
public void setRights(String rights) {
_rights = rights;
}
/**
* Returns the subtitle
*
* @return Returns the subtitle.
* @since Atom 1.0
*/
public Content getSubtitle() {
return _subtitle;
}
/**
* Set the subtitle
*
* @param subtitle The subtitle to set.
* @since Atom 1.0
*/
public void setSubtitle(Content subtitle) {
_subtitle = subtitle;
}
/**
* Returns the updated
*
* @return Returns the updated.
* @since Atom 1.0
*/
public Date getUpdated() {
return _updated;
}
/**
* Set the updated
*
* @param updated The updated to set.
* @since Atom 1.0
*/
public void setUpdated(Date updated) {
_updated = updated;
}
/**
* Returns the xmlBase
*
* @return Returns the xmlBase.
* @since Atom 1.0
*/
public String getXmlBase() {
return _xmlBase;
}
/**
* Set the xmlBase
*
* @param xmlBase The xmlBase to set.
* @since Atom 1.0
*/
public void setXmlBase(String xmlBase) {
_xmlBase = xmlBase;
}
}