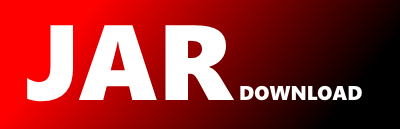
com.sun.syndication.feed.impl.CloneableBean Maven / Gradle / Ivy
/*
* Copyright 2004 Sun Microsystems, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package com.sun.syndication.feed.impl;
import java.beans.PropertyDescriptor;
import java.io.Serializable;
import java.lang.reflect.Array;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.util.*;
/**
* Provides deep Bean clonning support.
*
* It works on all read/write properties, recursively. It support all primitive types, Strings, Collections,
* Cloneable objects and multi-dimensional arrays of any of them.
*
* @author Alejandro Abdelnur
*
*/
public class CloneableBean implements Serializable, Cloneable {
private static final Class[] NO_PARAMS_DEF = new Class[0];
private static final Object[] NO_PARAMS = new Object[0];
private Object _obj;
private Set _ignoreProperties;
/**
* Default constructor.
*
* To be used by classes extending CloneableBean only.
*
*
*/
protected CloneableBean() {
_obj = this;
}
/**
* Creates a CloneableBean to be used in a delegation pattern.
*
* For example:
*
*
* public class Foo implements Cloneable {
* private CloneableBean _cloneableBean;
*
* public Foo() {
* _cloneableBean = new CloneableBean(this);
* }
*
* public Object clone() throws CloneNotSupportedException {
* return _cloneableBean.beanClone();
* }
*
* }
*
*
* @param obj object bean to clone.
*
*/
public CloneableBean(Object obj) {
this(obj,null);
}
/**
* Creates a CloneableBean to be used in a delegation pattern.
*
* The property names in the ignoreProperties Set will not be copied into
* the cloned instance. This is useful for cases where the Bean has convenience
* properties (properties that are actually references to other properties or
* properties of properties). For example SyndFeed and SyndEntry beans have
* convenience properties, publishedDate, author, copyright and categories all
* of them mapped to properties in the DC Module.
*
* @param obj object bean to clone.
* @param ignoreProperties properties to ignore when cloning.
*
*/
public CloneableBean(Object obj,Set ignoreProperties) {
_obj = obj;
_ignoreProperties = (ignoreProperties!=null) ? ignoreProperties : Collections.EMPTY_SET;
}
/**
* Makes a deep bean clone of the object.
*
* To be used by classes extending CloneableBean. Although it works also for classes using
* CloneableBean in a delegation pattern, for correctness those classes should use the
* @see #beanClone() beanClone method.
*
* @return a clone of the object bean.
* @throws CloneNotSupportedException thrown if the object bean could not be cloned.
*
*/
public Object clone() throws CloneNotSupportedException {
return beanClone();
}
/**
* Makes a deep bean clone of the object passed in the constructor.
*
* To be used by classes using CloneableBean in a delegation pattern,
* @see #CloneableBean(Object) constructor.
*
* @return a clone of the object bean.
* @throws CloneNotSupportedException thrown if the object bean could not be cloned.
*
*/
public Object beanClone() throws CloneNotSupportedException {
Object clonedBean;
try {
clonedBean = _obj.getClass().newInstance();
PropertyDescriptor[] pds = BeanIntrospector.getPropertyDescriptors(_obj.getClass());
if (pds!=null) {
for (int i=0;i