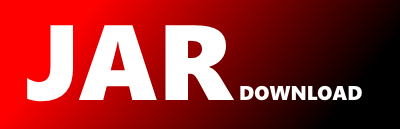
com.sun.syndication.feed.rss.Channel Maven / Gradle / Ivy
/*
* Copyright 2004 Sun Microsystems, Inc.
* Copyright 2011 The ROME Team
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package com.sun.syndication.feed.rss;
import com.sun.syndication.feed.WireFeed;
import com.sun.syndication.feed.module.Module;
import com.sun.syndication.feed.module.impl.ModuleUtils;
import java.util.*;
/**
* Bean for RSS feeds.
*
* It handles all RSS versions (0.9, 0.91, 0.92, 0.93, 0.94, 1.0 and 2.0)
* without losing information.
*
* @author Alejandro Abdelnur
*
*/
public class Channel extends WireFeed {
public static final String SUNDAY = "sunday";
public static final String MONDAY = "monday";
public static final String TUESDAY = "tuesday";
public static final String WEDNESDAY = "wednesday";
public static final String THURSDAY = "thursday";
public static final String FRIDAY = "friday";
public static final String SATURDAY = "saturday";
private static final Set DAYS;
static {
HashSet days = new HashSet();
days.add(SUNDAY );
days.add(MONDAY );
days.add(TUESDAY );
days.add(WEDNESDAY);
days.add(THURSDAY );
days.add(FRIDAY );
days.add(SATURDAY );
DAYS = Collections.unmodifiableSet(days);
}
private String _title;
private String _description;
private String _link;
private String _uri;
private Image _image;
private List _items;
private TextInput _textInput;
private String _language;
private String _rating;
private String _copyright;
private Date _pubDate;
private Date _lastBuildDate;
private String _docs;
private String _managingEditor;
private String _webMaster;
private List _skipHours;
private List _skipDays;
private Cloud _cloud;
private List _categories;
private String _generator;
private int _ttl = -1;
private List _modules;
/**
* Default constructor, for bean cloning purposes only.
*
*/
public Channel() {
}
/**
* Channel Constructor. All properties, except the type, are set to null.
*
* @param type the type of the RSS feed.
*
*/
public Channel(String type) {
super(type);
}
/**
* Returns the channel title.
*
* @return the channel title, null if none.
*
*/
public String getTitle() {
return _title;
}
/**
* Sets the channel title.
*
* @param title the channel title to set, null if none.
*
*/
public void setTitle(String title) {
_title = title;
}
/**
* Returns the channel description.
*
* @return the channel description, null if none.
*
*/
public String getDescription() {
return _description;
}
/**
* Sets the channel description.
*
* @param description the channel description to set, null if none.
*
*/
public void setDescription(String description) {
_description = description;
}
/**
* Returns the channel link.
*
* @return the channel link, null if none.
*
*/
public String getLink() {
return _link;
}
/**
* Sets the channel link.
*
* @param link the channel link to set, null if none.
*
*/
public void setLink(String link) {
_link = link;
}
/**
* Returns the channel uri.
*
* @return the channel uri, null if none.
*/
public String getUri() {
return _uri;
}
/**
* Sets the channel uri.
*
* @param uri the channel uri, null if none.
*/
public void setUri(String uri) {
_uri = uri;
}
/**
* Returns the channel image.
*
* @return the channel image, null if none.
*
*/
public Image getImage() {
return _image;
}
/**
* Sets the channel image.
*
* @param image the channel image to set, null if none.
*
*/
public void setImage(Image image) {
_image = image;
}
/**
* Returns the channel items.
*
* @return a list of Item elements with the channel items,
* an empty list if none.
*
*/
public List- getItems() {
return (_items==null) ? (_items=new ArrayList
- ()) : _items;
}
/**
* Sets the channel items.
*
* @param items the list of Item elements with the channel items to set,
* an empty list or null if none.
*
*/
public void setItems(List- items) {
_items = items;
}
/**
* Returns the channel text input.
*
* @return the channel text input, null if none.
*
*/
public TextInput getTextInput() {
return _textInput;
}
/**
* Sets the channel text input.
*
* @param textInput the channel text input to set, null if none.
*
*/
public void setTextInput(TextInput textInput) {
_textInput = textInput;
}
/**
* Returns the channel language.
*
* @return the channel language, null if none.
*
*/
public String getLanguage() {
return _language;
}
/**
* Sets the channel language.
*
* @param language the channel language to set, null if none.
*
*/
public void setLanguage(String language) {
_language = language;
}
/**
* Returns the channel rating.
*
* @return the channel rating, null if none.
*
*/
public String getRating() {
return _rating;
}
/**
* Sets the channel rating.
*
* @param rating the channel rating to set, null if none.
*
*/
public void setRating(String rating) {
_rating = rating;
}
/**
* Returns the channel copyright.
*
* @return the channel copyright, null if none.
*
*/
public String getCopyright() {
return _copyright;
}
/**
* Sets the channel copyright.
*
* @param copyright the channel copyright to set, null if none.
*
*/
public void setCopyright(String copyright) {
_copyright = copyright;
}
/**
* Returns the channel publishing date.
*
* @return the channel publishing date, null if none.
*
*/
public Date getPubDate() {
return _pubDate == null ? null : new Date(_pubDate.getTime());
}
/**
* Sets the channel publishing date.
*
* @param pubDate the channel publishing date to set, null if none.
*
*/
public void setPubDate(Date pubDate) {
_pubDate = pubDate == null ? null : new Date( pubDate.getTime());
}
/**
* Returns the channel last build date.
*
* @return the channel last build date, null if none.
*
*/
public Date getLastBuildDate() {
return _lastBuildDate == null ? null : new Date(_lastBuildDate.getTime());
}
/**
* Sets the channel last build date.
*
* @param lastBuildDate the channel last build date to set, null if none.
*
*/
public void setLastBuildDate(Date lastBuildDate) {
_lastBuildDate = lastBuildDate == null ? null : new Date( lastBuildDate.getTime());
}
/**
* Returns the channel docs.
*
* @return the channel docs, null if none.
*
*/
public String getDocs() {
return _docs;
}
/**
* Sets the channel docs.
*
* @param docs the channel docs to set, null if none.
*
*/
public void setDocs(String docs) {
_docs = docs;
}
/**
* Returns the channel managing editor.
*
* @return the channel managing editor, null if none.
*
*/
public String getManagingEditor() {
return _managingEditor;
}
/**
* Sets the channel managing editor.
*
* @param managingEditor the channel managing editor to set, null if none.
*
*/
public void setManagingEditor(String managingEditor) {
_managingEditor = managingEditor;
}
/**
* Returns the channel web master.
*
* @return the channel web master, null if none.
*
*/
public String getWebMaster() {
return _webMaster;
}
/**
* Sets the channel web master.
*
* @param webMaster the channel web master to set, null if none.
*
*/
public void setWebMaster(String webMaster) {
_webMaster = webMaster;
}
/**
* Returns the channel skip hours.
*
* @return a list of Integer elements with the channel skip hours,
* an empty list if none.
*
*/
public List getSkipHours() {
return (_skipHours!=null) ? _skipHours : new ArrayList();
}
/**
* Sets the channel skip hours.
*
* @param skipHours the list of Integer elements with the channel skip hours to set,
* an empty list or null if none.
*
*/
public void setSkipHours(List skipHours) {
if (skipHours!=null) {
for (int i=0;i24) {
throw new IllegalArgumentException("Invalid hour ["+hour+"]");
}
}
else {
throw new IllegalArgumentException("Invalid hour [null]");
}
}
}
_skipHours = skipHours;
}
/**
* Returns the channel skip days.
*
* @return a list of Day elements with the channel skip days,
* an empty list if none.
*
*/
public List getSkipDays() {
return (_skipDays!=null) ? _skipDays : new ArrayList();
}
/**
* Sets the channel skip days.
*
* @param skipDays the list of Day elements with the channel skip days to set,
* an empty list or null if none.
*
*/
public void setSkipDays(List skipDays) {
if (skipDays!=null) {
for (int i=0;i
* @return the channel cloud, null if none.
*
*/
public Cloud getCloud() {
return _cloud;
}
/**
* Sets the channel cloud.
*
* @param cloud the channel cloud to set, null if none.
*
*/
public void setCloud(Cloud cloud) {
_cloud = cloud;
}
/**
* Returns the channel categories.
*
* @return a list of Category elements with the channel categories,
* an empty list if none.
*
*/
public List getCategories() {
return (_categories==null) ? (_categories=new ArrayList()) : _categories;
}
/**
* Sets the channel categories.
*
* @param categories the list of Category elements with the channel categories to set,
* an empty list or null if none.
*
*/
public void setCategories(List categories) {
_categories = categories;
}
/**
* Returns the channel generator.
*
* @return the channel generator, null if none.
*
*/
public String getGenerator() {
return _generator;
}
/**
* Sets the channel generator.
*
* @param generator the channel generator to set, null if none.
*
*/
public void setGenerator(String generator) {
_generator = generator;
}
/**
* Returns the channel time to live.
*
* @return the channel time to live, null if none.
*
*/
public int getTtl() {
return _ttl;
}
/**
* Sets the channel time to live.
*
* @param ttl the channel time to live to set, null if none.
*
*/
public void setTtl(int ttl) {
_ttl = ttl;
}
/**
* Returns the channel modules.
*
* @return a list of ModuleImpl elements with the channel modules,
* an empty list if none.
*
*/
@Override
public List getModules() {
return (_modules==null) ? (_modules=new ArrayList()) : _modules;
}
/**
* Sets the channel modules.
*
* @param modules the list of ModuleImpl elements with the channel modules to set,
* an empty list or null if none.
*
*/
@Override
public void setModules(List modules) {
_modules = modules;
}
/**
* Returns the module identified by a given URI.
*
* @param uri the URI of the ModuleImpl.
* @return The module with the given URI, null if none.
*/
@Override
public Module getModule(String uri) {
return ModuleUtils.getModule(_modules,uri);
}
}