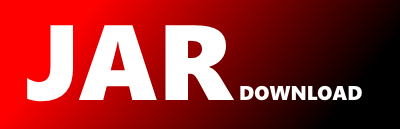
org.geotoolkit.internal.jaxb.geometry.ObjectFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of geotk-metadata Show documentation
Show all versions of geotk-metadata Show documentation
Implementations of metadata derived from ISO 19115. This module provides both an implementation
of the metadata interfaces defined in GeoAPI, and a framework for handling those metadata through
Java reflection.
The newest version!
/*
* Geotoolkit.org - An Open Source Java GIS Toolkit
* http://www.geotoolkit.org
*
* (C) 2010-2012, Open Source Geospatial Foundation (OSGeo)
* (C) 2010-2012, Geomatys
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation;
* version 2.1 of the License.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package org.geotoolkit.internal.jaxb.geometry;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
import org.geotoolkit.xml.Namespaces;
/**
* A minimalist XML object factory for getting JAXB to work without throwing exceptions when
* there is no GML module in the classpath. This factory is extended with more complete methods
* if the GML module.
*
* @author Guilhem Legal (Geomatys)
* @version 3.15
*
* @since 3.15
* @module
*/
@XmlRegistry
public class ObjectFactory {
/**
* The qualified name of {@code }.
*/
protected static final QName AbstractGeometry_QNAME = new QName(Namespaces.GML, "AbstractGeometry");
/**
* The qualified name of {@code }.
*/
protected static final QName AbstractGML_QNAME = new QName(Namespaces.GML, "AbstractGML");
/**
* The qualified name of {@code }.
*/
protected static final QName AbstractObject_QNAME = new QName(Namespaces.GML, "AbstractObject");
/**
* Creates an instance of {@code JAXBElement
© 2015 - 2025 Weber Informatics LLC | Privacy Policy