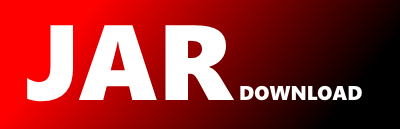
org.geotoolkit.metadata.MetadataFactory Maven / Gradle / Ivy
Show all versions of geotk-metadata Show documentation
/*
* Geotoolkit.org - An Open Source Java GIS Toolkit
* http://www.geotoolkit.org
*
* (C) 2009-2012, Open Source Geospatial Foundation (OSGeo)
* (C) 2009-2012, Geomatys
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation;
* version 2.1 of the License.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package org.geotoolkit.metadata;
import java.util.Set;
import java.util.Map;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedHashSet;
import net.jcip.annotations.ThreadSafe;
import org.opengis.util.FactoryException;
import org.geotoolkit.factory.Factory;
import org.geotoolkit.factory.FactoryFinder;
import org.geotoolkit.resources.Errors;
import static org.geotoolkit.util.collection.XCollections.isNullOrEmpty;
/**
* Create metadata objects of the given {@link Class} using the properties given in a {@link Map}.
* For any given type, this class tries to instantiate the metadata object in two ways:
*
*
* First, if a collection of factories has been given to the constructor,
* then this method will search for a {@code create(Map, ...)} method returning
* an object assignable to the requested one. For example if the user requests a
* {@link org.opengis.referencing.crs.VerticalCRS} and this {@code MetadataFactory} has been
* given a {@link org.opengis.referencing.crs.CRSFactory} at construction time, then the
* {@code CRSFactory.createVerticalCRS(Map, ...)} method will be used.
*
* If no suitable factory is found, then {@code MetadataFactory} will try to instantiate
* a metadata implementation directly using {@link Class#newInstance()}. The class can be an
* interface like {@link org.opengis.metadata.citation.Citation} or its implementation class
* like {@link org.geotoolkit.metadata.iso.citation.DefaultCitation}.
* The keys in the map shall be the {@linkplain KeyNamePolicy#UML_IDENTIFIER UML identifiers}
* or the {@linkplain KeyNamePolicy#JAVABEANS_PROPERTY Java Beans name} of metadata properties,
* for example {@code "title"} for the value to be returned by
* {@link org.opengis.metadata.citation.Citation#getTitle()}.
*
*
* @author Martin Desruisseaux (Geomatys)
* @version 3.03
*
* @since 3.03
* @module
*/
@ThreadSafe
public class MetadataFactory extends Factory {
/**
* An optional set of factories to try before to create the metadata objects directly.
* This is {@code null} if there is no such factory.
*/
private final Object[] factories;
/**
* The standards implemented by this factory.
*/
private final MetadataStandard[] standards;
/**
* The methods to use for instantiating object of the classes in the keys.
* This is {@code null} if {@code factories} is null.
*/
private final Map, FactoryMethod> factoryMethods;
/**
* Creates a new factory implementing the {@linkplain MetadataStandard#ISO_19115 ISO 19115}
* standard and using the referencing factories known to {@link FactoryFinder}.
*/
public MetadataFactory() {
this(factories(), MetadataStandard.ISO_19115);
}
/**
* Creates a new factory for the given metadata standards and the referencing factories
* known to {@link FactoryFinder}.
*
* @param standards The metadata standards implemented by this factory.
*/
public MetadataFactory(final MetadataStandard... standards) {
this(factories(), standards);
}
/**
* Creates a new factory for the given metadata standards. The given collection of
* factories will be used if possible before to instantiate a metadata object directly.
*
* @param factories The factories to try before to instantiate the metadata directly,
* or {@code null} if none.
* @param standards The metadata standards implemented by this factory.
*/
public MetadataFactory(final Set> factories, final MetadataStandard... standards) {
if (!isNullOrEmpty(factories)) {
this.factories = factories.toArray();
factoryMethods = new HashMap, FactoryMethod>();
} else {
this.factories = null;
factoryMethods = null;
}
this.standards = standards.clone();
}
/**
* Returns the factories declared in {@link FactoryFinder}.
*/
private static Set> factories() {
final Set