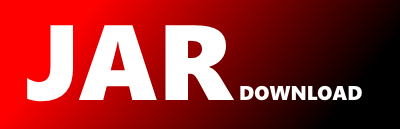
org.geotoolkit.referencing.operation.matrix.Matrix3 Maven / Gradle / Ivy
/*
* Geotoolkit.org - An Open Source Java GIS Toolkit
* http://www.geotoolkit.org
*
* (C) 2005-2012, Open Source Geospatial Foundation (OSGeo)
* (C) 2009-2012, Geomatys
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation;
* version 2.1 of the License.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package org.geotoolkit.referencing.operation.matrix;
import javax.vecmath.Matrix3d;
import java.awt.geom.AffineTransform;
import org.opengis.referencing.operation.Matrix;
import org.geotoolkit.math.XMath;
import org.geotoolkit.resources.Errors;
import org.geotoolkit.util.Utilities;
import org.geotoolkit.util.ComparisonMode;
/**
* A matrix of fixed {@value #SIZE}×{@value #SIZE} size. This specialized matrix provides
* better accuracy than {@link GeneralMatrix} for matrix inversion and multiplication.
*
* @author Martin Desruisseaux (IRD, Geomatys)
* @version 3.20
*
* @since 2.2
* @module
*/
public class Matrix3 extends Matrix3d implements XMatrix {
/**
* Serial number for inter-operability with different versions.
*/
private static final long serialVersionUID = 8902061778871586611L;
/**
* The matrix size, which is {@value}.
*/
public static final int SIZE = 3;
/**
* Creates a new identity matrix.
*/
public Matrix3() {
setIdentity();
}
/**
* Creates a new matrix initialized to the specified values.
*
* @param m00 The first matrix element in the first row.
* @param m01 The second matrix element in the first row.
* @param m02 The third matrix element in the first row.
* @param m10 The first matrix element in the second row.
* @param m11 The second matrix element in the second row.
* @param m12 The third matrix element in the second row.
* @param m20 The first matrix element in the third row.
* @param m21 The second matrix element in the third row.
* @param m22 The third matrix element in the third row.
*/
public Matrix3(double m00, double m01, double m02,
double m10, double m11, double m12,
double m20, double m21, double m22)
{
super(m00, m01, m02,
m10, m11, m12,
m20, m21, m22);
}
/**
* Creates a new matrix initialized to the specified values.
* The length of the given array must be 9 and the values in
* the same order than the above constructor.
*
* @param elements Elements of the matrix. Column indices vary fastest.
*
* @since 3.00
*/
public Matrix3(final double[] elements) {
super(elements[0], elements[1], elements[2],
elements[3], elements[4], elements[5],
elements[6], elements[7], elements[8]);
/*
* Should have been first if Sun fixed RFE #4093999 in their bug database
* ("Relax constraint on placement of this()/super() call in constructors").
*/
if (elements.length != (SIZE*SIZE)) {
throw new IllegalArgumentException(Errors.format(Errors.Keys.MISMATCHED_ARRAY_LENGTH));
}
}
/**
* Constructs a 3×3 matrix from the specified affine transform.
*
* @param transform The affine transform to copy.
*
* @see #toAffineTransform
*/
public Matrix3(final AffineTransform transform) {
setMatrix(transform);
}
/**
* Creates a new matrix initialized to the same value than the specified one.
* The specified matrix size must be {@value #SIZE}×{@value #SIZE}.
*
* @param matrix The matrix to copy.
* @throws IllegalArgumentException if the given matrix is not of the expected size.
*/
public Matrix3(final Matrix matrix) throws IllegalArgumentException {
if (matrix.getNumRow() != SIZE || matrix.getNumCol() != SIZE) {
throw new IllegalArgumentException(Errors.format(Errors.Keys.ILLEGAL_MATRIX_SIZE));
}
for (int j=0; j
© 2015 - 2025 Weber Informatics LLC | Privacy Policy