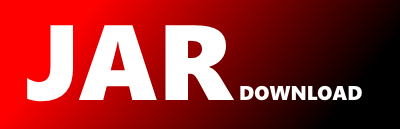
org.geotoolkit.referencing.operation.provider.ObliqueStereographic Maven / Gradle / Ivy
/*
* Geotoolkit.org - An Open Source Java GIS Toolkit
* http://www.geotoolkit.org
*
* (C) 1999-2012, Open Source Geospatial Foundation (OSGeo)
* (C) 2009-2012, Geomatys
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation;
* version 2.1 of the License.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package org.geotoolkit.referencing.operation.provider;
import net.jcip.annotations.Immutable;
import org.opengis.parameter.ParameterDescriptor;
import org.opengis.parameter.ParameterDescriptorGroup;
import org.opengis.referencing.ReferenceIdentifier;
import org.geotoolkit.referencing.NamedIdentifier;
import org.geotoolkit.metadata.iso.citation.Citations;
/**
* The provider for "Oblique Stereographic" projection using EPSG equations (EPSG:9809).
* The math transform implementations instantiated by this provider may be any of the following classes:
*
*
* - {@link org.geotoolkit.referencing.operation.projection.ObliqueStereographic}
*
*
*
* The following table summarizes the parameters recognized by this provider.
* For a more detailed parameter list, see the {@link #PARAMETERS} constant.
* Operation name: {@code Oblique_Stereographic}
*
Area of use: (union of CRS domains of validity in EPSG database)
*
* in latitudes: 32°18.6′N to 54°52.2′N
* in longitudes: 69°03.0′W to 42°22.8′E
*
*
* Parameter name Default value
* {@code semi_major}
* {@code semi_minor}
* {@code roll_longitude} false
* {@code central_meridian} 0°
* {@code latitude_of_origin} 0°
* {@code scale_factor} 1
* {@code false_easting} 0 metres
* {@code false_northing} 0 metres
*
*
*
* @author Rueben Schulz (UBC)
* @author Martin Desruisseaux (Geomatys)
* @version 3.20
*
* @see Oblique Stereographic on RemoteSensing.org
* @see Geotk coordinate operations matrix
*
* @since 2.4
* @module
*/
@Immutable
public class ObliqueStereographic extends Stereographic {
/**
* For compatibility with different versions during deserialization.
*/
private static final long serialVersionUID = 6505988910141381354L;
/**
* The operation parameter descriptor for the {@linkplain
* org.geotoolkit.referencing.operation.projection.UnitaryProjection.Parameters#centralMeridian
* central meridian} parameter value.
*
* This parameter is mandatory.
* Valid values range is [-180 … 180]° and default value is 0°.
*
* @deprecated Invoke {@linkplain #PARAMETERS}.{@linkplain ParameterDescriptorGroup#descriptor(String)
* descriptor(String)}
instead.
*/
@Deprecated
public static final ParameterDescriptor CENTRAL_MERIDIAN;
/**
* The operation parameter descriptor for the {@linkplain
* org.geotoolkit.referencing.operation.projection.UnitaryProjection.Parameters#latitudeOfOrigin
* latitude of origin} parameter value.
*
* This parameter is mandatory.
* Valid values range is [-90 … 90]° and default value is 0°.
*
* @deprecated Invoke {@linkplain #PARAMETERS}.{@linkplain ParameterDescriptorGroup#descriptor(String)
* descriptor(String)}
instead.
*/
@Deprecated
public static final ParameterDescriptor LATITUDE_OF_ORIGIN;
/**
* The group of all parameters expected by this coordinate operation.
* The following table lists the operation names and the parameters recognized by Geotk:
*
*
*
*
*
* Name: OGC
:Oblique_Stereographic
* Alias: EPSG
:Oblique Stereographic
* EPSG
:Roussilhe
* ESRI
:Double_Stereographic
* GeoTIFF
:CT_ObliqueStereographic
* PROJ4
:sterea
* Geotk
:Stereographic projection
* Identifier: EPSG
:9809
* GeoTIFF
:16
*
*
*
*
* Name: OGC
:semi_major
* Alias: EPSG
:Semi-major axis
* ESRI
:Semi_Major
* GeoTIFF
:SemiMajor
* PROJ4
:a
*
*
*
* Type: {@code Double}
* Obligation: mandatory
* Value range: [0…∞) metres
*
*
*
*
* Name: OGC
:semi_minor
* Alias: EPSG
:Semi-minor axis
* ESRI
:Semi_Minor
* GeoTIFF
:SemiMinor
* PROJ4
:b
*
*
*
* Type: {@code Double}
* Obligation: mandatory
* Value range: [0…∞) metres
*
*
*
*
* Name: Geotk
:roll_longitude
*
*
*
* Type: {@code Boolean}
* Obligation: optional
* Default value: false
*
*
*
*
* Name: OGC
:central_meridian
* Alias: EPSG
:Longitude of natural origin
* ESRI
:Central_Meridian
* GeoTIFF
:NatOriginLong
* PROJ4
:lon_0
*
*
*
* Type: {@code Double}
* Obligation: mandatory
* Value range: [-180 … 180]°
* Default value: 0°
*
*
*
*
* Name: OGC
:latitude_of_origin
* Alias: EPSG
:Latitude of natural origin
* ESRI
:Latitude_Of_Origin
* GeoTIFF
:NatOriginLat
* PROJ4
:lat_0
*
*
*
* Type: {@code Double}
* Obligation: mandatory
* Value range: [-90 … 90]°
* Default value: 0°
*
*
*
*
* Name: OGC
:scale_factor
* Alias: EPSG
:Scale factor at natural origin
* ESRI
:Scale_Factor
* GeoTIFF
:ScaleAtNatOrigin
* PROJ4
:k
*
*
*
* Type: {@code Double}
* Obligation: optional
* Value range: [0…∞)
* Default value: 1
*
*
*
*
* Name: OGC
:false_easting
* Alias: EPSG
:False easting
* ESRI
:False_Easting
* GeoTIFF
:FalseEasting
* PROJ4
:x_0
*
*
*
* Type: {@code Double}
* Obligation: mandatory
* Value range: (-∞ … ∞) metres
* Default value: 0 metres
*
*
*
*
* Name: OGC
:false_northing
* Alias: EPSG
:False northing
* ESRI
:False_Northing
* GeoTIFF
:FalseNorthing
* PROJ4
:y_0
*
*
*
* Type: {@code Double}
* Obligation: mandatory
* Value range: (-∞ … ∞) metres
* Default value: 0 metres
*
*
*
*/
@SuppressWarnings("hiding")
public static final ParameterDescriptorGroup PARAMETERS;
static {
CENTRAL_MERIDIAN = CassiniSoldner.CENTRAL_MERIDIAN;
LATITUDE_OF_ORIGIN = CassiniSoldner.LATITUDE_OF_ORIGIN;
PARAMETERS = UniversalParameters.createDescriptorGroup(new ReferenceIdentifier[] {
new NamedIdentifier(Citations.OGC, "Oblique_Stereographic"),
new NamedIdentifier(Citations.EPSG, "Oblique Stereographic"),
new NamedIdentifier(Citations.EPSG, "Roussilhe"),
new IdentifierCode (Citations.EPSG, 9809),
new NamedIdentifier(Citations.ESRI, "Double_Stereographic"),
new NamedIdentifier(Citations.GEOTIFF, "CT_ObliqueStereographic"),
new IdentifierCode (Citations.GEOTIFF, 16),
new NamedIdentifier(Citations.PROJ4, "sterea"),
sameNameAs(Citations.GEOTOOLKIT, Stereographic.PARAMETERS)
}, null, new ParameterDescriptor>[] {
sameParameterAs(EquidistantCylindrical.PARAMETERS, "semi_major"),
sameParameterAs(EquidistantCylindrical.PARAMETERS, "semi_minor"),
ROLL_LONGITUDE,
CENTRAL_MERIDIAN, LATITUDE_OF_ORIGIN,
Orthographic.SCALE_FACTOR,
EquidistantCylindrical.FALSE_EASTING,
EquidistantCylindrical.FALSE_NORTHING
}, MapProjectionDescriptor.ADD_EARTH_RADIUS);
}
/**
* Constructs a new provider.
*/
public ObliqueStereographic() {
super(PARAMETERS);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy