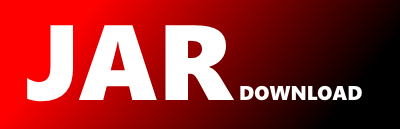
org.opencb.cellbase.lib.managers.ClinicalManager Maven / Gradle / Ivy
/*
* Copyright 2015-2020 OpenCB
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.opencb.cellbase.lib.managers;
import org.opencb.biodata.models.core.Gene;
import org.opencb.biodata.models.variant.Variant;
import org.opencb.biodata.models.variant.avro.*;
import org.opencb.cellbase.core.api.ClinicalVariantQuery;
import org.opencb.cellbase.core.api.query.CellBaseQueryOptions;
import org.opencb.cellbase.core.api.query.QueryException;
import org.opencb.cellbase.core.config.CellBaseConfiguration;
import org.opencb.cellbase.core.exception.CellBaseException;
import org.opencb.cellbase.core.result.CellBaseDataResult;
import org.opencb.cellbase.lib.impl.core.CellBaseCoreDBAdaptor;
import org.opencb.cellbase.lib.impl.core.ClinicalMongoDBAdaptor;
import org.opencb.cellbase.lib.iterator.CellBaseIterator;
import org.opencb.cellbase.core.api.key.ApiKeyLicensedDataUtils;
import org.opencb.cellbase.lib.iterator.ApiKeyFilteredVariantIterator;
import org.opencb.commons.datastore.core.Query;
import org.opencb.commons.datastore.core.QueryOptions;
import java.util.*;
import java.util.stream.Collectors;
import static org.opencb.commons.datastore.core.QueryOptions.EXCLUDE;
import static org.opencb.commons.datastore.core.QueryOptions.INCLUDE;
public class ClinicalManager extends AbstractManager implements AggregationApi {
private ClinicalMongoDBAdaptor clinicalDBAdaptor;
public ClinicalManager(String species, CellBaseConfiguration configuration) throws CellBaseException {
this(species, null, configuration);
}
public ClinicalManager(String species, String assembly, CellBaseConfiguration configuration) throws CellBaseException {
super(species, assembly, configuration);
this.init();
}
private void init() throws CellBaseException {
clinicalDBAdaptor = dbAdaptorFactory.getClinicalDBAdaptor(new GenomeManager(species, assembly, configuration));
}
@Override
public CellBaseCoreDBAdaptor getDBAdaptor() {
return clinicalDBAdaptor;
}
@Override
public CellBaseDataResult search(ClinicalVariantQuery query) throws QueryException, IllegalAccessException, CellBaseException {
query.setDefaults();
query.validate();
CellBaseDataResult results = getDBAdaptor().query(query);
Set validSources = apiKeyManager.getValidSources(query.getApiKey(), ApiKeyLicensedDataUtils.UNLICENSED_CLINICAL_DATA);
// Check if is necessary to use the API key licensed variant iterator
if (ApiKeyLicensedDataUtils.needFiltering(validSources, ApiKeyLicensedDataUtils.LICENSED_CLINICAL_DATA)) {
return ApiKeyLicensedDataUtils.filterDataSources(results, validSources);
} else {
return results;
}
}
@Override
public List> search(List queries) throws QueryException, IllegalAccessException,
CellBaseException {
List> results = new ArrayList<>();
for (ClinicalVariantQuery query : queries) {
results.add(search(query));
}
return results;
}
@Override
public List> info(List ids, CellBaseQueryOptions queryOptions, int dataRelease, String apiKey)
throws CellBaseException {
List> results = getDBAdaptor().info(ids, queryOptions, dataRelease, apiKey);
Set validSources = apiKeyManager.getValidSources(apiKey, ApiKeyLicensedDataUtils.UNLICENSED_CLINICAL_DATA);
// Check if is necessary to use the API key licensed variant iterator
if (ApiKeyLicensedDataUtils.needFiltering(validSources, ApiKeyLicensedDataUtils.LICENSED_CLINICAL_DATA)) {
return ApiKeyLicensedDataUtils.filterDataSources(results, validSources);
} else {
return results;
}
}
@Override
public CellBaseIterator iterator(ClinicalVariantQuery query) throws CellBaseException {
Set validSources = apiKeyManager.getValidSources(query.getApiKey(), ApiKeyLicensedDataUtils.UNLICENSED_CLINICAL_DATA);
// Check if is necessary to use the API key licensed variant iterator
if (ApiKeyLicensedDataUtils.needFiltering(validSources, ApiKeyLicensedDataUtils.LICENSED_CLINICAL_DATA)) {
return new ApiKeyFilteredVariantIterator(getDBAdaptor().iterator(query), validSources);
} else {
return getDBAdaptor().iterator(query);
}
}
public CellBaseDataResult search(Query query, QueryOptions queryOptions) throws CellBaseException {
CellBaseDataResult result = clinicalDBAdaptor.nativeGet(query, queryOptions);
Set validSources = apiKeyManager.getValidSources(getApiKey(queryOptions), ApiKeyLicensedDataUtils.UNLICENSED_CLINICAL_DATA);
List includes = null;
if (queryOptions.containsKey(INCLUDE)) {
includes = Arrays.asList(queryOptions.getString(INCLUDE).split(","));
}
List excludes = null;
if (queryOptions.containsKey(EXCLUDE)) {
includes = Arrays.asList(queryOptions.getString(EXCLUDE).split(","));
}
// Check if is necessary to use the API key licensed variant iterator
if (ApiKeyLicensedDataUtils.needFiltering(validSources, ApiKeyLicensedDataUtils.LICENSED_CLINICAL_DATA)) {
return ApiKeyLicensedDataUtils.filterDataSources(result, validSources);
} else {
return result;
}
}
public CellBaseDataResult getAlleleOriginLabels() {
List alleleOriginLabels = Arrays.stream(AlleleOrigin.values())
.map((value) -> value.name()).collect(Collectors.toList());
return new CellBaseDataResult("allele_origin_labels", 0, Collections.emptyList(),
alleleOriginLabels.size(), alleleOriginLabels, alleleOriginLabels.size());
}
public CellBaseDataResult getModeInheritanceLabels() {
List modeInheritanceLabels = Arrays.stream(ModeOfInheritance.values())
.map((value) -> value.name()).collect(Collectors.toList());
return new CellBaseDataResult("mode_inheritance_labels", 0, Collections.emptyList(),
modeInheritanceLabels.size(), modeInheritanceLabels, modeInheritanceLabels.size());
}
public CellBaseDataResult getClinsigLabels() {
List clinsigLabels = Arrays.stream(ClinicalSignificance.values())
.map((value) -> value.name()).collect(Collectors.toList());
return new CellBaseDataResult("clinsig_labels", 0, Collections.emptyList(),
clinsigLabels.size(), clinsigLabels, clinsigLabels.size());
}
public CellBaseDataResult getConsistencyLabels() {
List consistencyLabels = Arrays.stream(ConsistencyStatus.values())
.map((value) -> value.name()).collect(Collectors.toList());
return new CellBaseDataResult("consistency_labels", 0, Collections.emptyList(),
consistencyLabels.size(), consistencyLabels, consistencyLabels.size());
}
public CellBaseDataResult getVariantTypes() {
List variantTypes = Arrays.stream(VariantType.values())
.map((value) -> value.name()).collect(Collectors.toList());
return new CellBaseDataResult("variant_types", 0, Collections.emptyList(),
variantTypes.size(), variantTypes, variantTypes.size());
}
public List> getByVariant(List variants, List geneList,
QueryOptions queryOptions, int dataRelease) throws CellBaseException {
List> results = clinicalDBAdaptor.getByVariant(variants, geneList, queryOptions, dataRelease);
Set validSources = apiKeyManager.getValidSources(getApiKey(queryOptions), ApiKeyLicensedDataUtils.UNLICENSED_CLINICAL_DATA);
// TODO: take into account includes and excludes
List includes = null;
if (queryOptions.containsKey(INCLUDE)) {
includes = Arrays.asList(queryOptions.getString(INCLUDE).split(","));
}
List excludes = null;
if (queryOptions.containsKey(EXCLUDE)) {
includes = Arrays.asList(queryOptions.getString(EXCLUDE).split(","));
}
// Check if is necessary to use the API key licensed variant iterator
if (ApiKeyLicensedDataUtils.needFiltering(validSources, ApiKeyLicensedDataUtils.LICENSED_CLINICAL_DATA)) {
return ApiKeyLicensedDataUtils.filterDataSources(results, validSources);
} else {
return results;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy