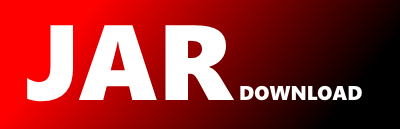
us.ihmc.euclid.referenceFrame.interfaces.FixedFrameRotationMatrixBasics Maven / Gradle / Ivy
package us.ihmc.euclid.referenceFrame.interfaces;
import us.ihmc.euclid.exceptions.NotARotationMatrixException;
import us.ihmc.euclid.matrix.interfaces.Matrix3DReadOnly;
import us.ihmc.euclid.matrix.interfaces.RotationMatrixBasics;
import us.ihmc.euclid.matrix.interfaces.RotationMatrixReadOnly;
import us.ihmc.euclid.orientation.interfaces.Orientation3DReadOnly;
import us.ihmc.euclid.referenceFrame.ReferenceFrame;
import us.ihmc.euclid.referenceFrame.exceptions.ReferenceFrameMismatchException;
/**
* Write and read interface used for a 3-by-3 rotation matrix expressed in a constant reference
* frame, i.e. the reference frame of this object cannot be changed via this interface.
*
* A rotation matrix is used to represent a 3D orientation through its 9 coefficients. A rotation
* matrix has to comply to several constraints:
*
* - each column of the matrix represents a unitary vector,
*
- each row of the matrix represents a unitary vector,
*
- every pair of columns of the matrix represents two orthogonal vectors,
*
- every pair of rows of the matrix represents two orthogonal vectors,
*
- the matrix determinant is equal to {@code 1}.
*
* A rotation matrix has the nice property RT = R-1.
*
*
* @author Sylvain Bertrand
*/
public interface FixedFrameRotationMatrixBasics
extends FrameRotationMatrixReadOnly, RotationMatrixBasics, FixedFrameCommonMatrix3DBasics, FixedFrameOrientation3DBasics
{
/**
* Sets this rotation matrix to equal the given {@code other} and then normalizes {@code this}.
*
* @param other the rotation matrix to copy the values from. Not modified.
* @throws NotARotationMatrixException if the normalization failed.
* @throws ReferenceFrameMismatchException if the argument is not expressed in the same reference
* frame as {@code this}.
*/
default void setAndNormalize(FrameRotationMatrixReadOnly other)
{
checkReferenceFrameMatch(other);
RotationMatrixBasics.super.setAndNormalize(other);
}
/**
* {@inheritDoc}
*
* If the argument implements {@link RotationMatrixReadOnly}, a redirection
* {@link #set(RotationMatrixReadOnly)} is done.
*
*/
@Override
default void set(ReferenceFrame referenceFrame, Orientation3DReadOnly orientation)
{
checkReferenceFrameMatch(referenceFrame);
RotationMatrixBasics.super.set(orientation);
}
/**
* {@inheritDoc}
*
* If the argument implements {@link FrameRotationMatrixReadOnly}, a redirection
* {@link #set(FrameRotationMatrixReadOnly)} is done.
*
*/
@Override
default void set(FrameOrientation3DReadOnly orientation)
{
set(orientation.getReferenceFrame(), orientation);
}
/**
* Sets this rotation matrix to the invert of the given one {@code other}.
*
* This operation uses the property:
* R-1 = RT
* of a rotation matrix preventing to actually compute the inverse of the matrix.
*
*
* @param other the matrix to copy the values from. Not modified.
* @throws ReferenceFrameMismatchException if the argument is not expressed in the same reference
* frame as {@code this}.
*/
default void setAndInvert(FrameRotationMatrixReadOnly other)
{
checkReferenceFrameMatch(other);
RotationMatrixBasics.super.setAndInvert(other);
}
/** {@inheritDoc} */
@Override
default void setAndInvert(FrameOrientation3DReadOnly orientation)
{
FixedFrameOrientation3DBasics.super.setAndInvert(orientation);
}
/**
* Sets this matrix to {@code other}.
*
* If {@code other} is expressed in the frame as {@code this}, then this method is equivalent to
* {@link #set(ReferenceFrame, Matrix3DReadOnly)}.
*
*
* If {@code other} is expressed in a different frame than {@code this}, then {@code this} is set to
* {@code other} and then transformed to be expressed in {@code this.getReferenceFrame()}.
*
*
* If the argument implements {@link RotationMatrixReadOnly}, a redirection
* {@link #set(RotationMatrixReadOnly)} is done.
*
*
* @param referenceFrame the reference frame in which the argument is expressed.
* @param matrix the matrix to copy the values of. Not modified.
* @throws NotARotationMatrixException if the argument is not a rotation matrix.
*/
default void setMatchingFrame(ReferenceFrame referenceFrame, Matrix3DReadOnly matrix)
{
set(matrix);
referenceFrame.transformFromThisToDesiredFrame(getReferenceFrame(), this);
}
/**
* Sets this matrix to {@code other}.
*
* If {@code other} is expressed in the frame as {@code this}, then this method is equivalent to
* {@link #set(FrameMatrix3DReadOnly)}.
*
*
* If {@code other} is expressed in a different frame than {@code this}, then {@code this} is set to
* {@code other} and then transformed to be expressed in {@code this.getReferenceFrame()}.
*
*
* If the argument implements {@link FrameRotationMatrixReadOnly}, a redirection
* {@link #set(FrameRotationMatrixReadOnly)} is done.
*
*
* @param matrix the matrix to copy the values of. Not modified.
* @throws NotARotationMatrixException if the argument is not a rotation matrix.
*/
default void setMatchingFrame(FrameMatrix3DReadOnly matrix)
{
setMatchingFrame(matrix.getReferenceFrame(), matrix);
}
/**
* Sets this rotation matrix to {@code other} and copies the dirty and identity flags from the other
* matrix.
*
* If {@code other} is expressed in the frame as {@code this}, then this method is equivalent to
* {@link #set(RotationMatrixReadOnly)}.
*
*
* If {@code other} is expressed in a different frame than {@code this}, then {@code this} is set to
* {@code other} and then transformed to be expressed in {@code this.getReferenceFrame()}.
*
*
* @param referenceFrame the reference frame in which the argument is expressed.
* @param other the other rotation matrix to copy. Not modified.
*/
default void setMatchingFrame(ReferenceFrame referenceFrame, RotationMatrixReadOnly other)
{
set(other);
referenceFrame.transformFromThisToDesiredFrame(getReferenceFrame(), this);
}
/**
* Sets this rotation matrix to {@code other} and copies the dirty and identity flags from the other
* matrix.
*
* If {@code other} is expressed in the frame as {@code this}, then this method is equivalent to
* {@link #set(FrameRotationMatrixReadOnly)}.
*
*
* If {@code other} is expressed in a different frame than {@code this}, then {@code this} is set to
* {@code other} and then transformed to be expressed in {@code this.getReferenceFrame()}.
*
*
* @param other the other rotation matrix to copy. Not modified.
*/
default void setMatchingFrame(FrameRotationMatrixReadOnly other)
{
setMatchingFrame(other.getReferenceFrame(), other);
}
/**
* Sets this frame orientation to {@code orientation}.
*
* If {@code orientation} is expressed in the frame as {@code this}, then this method is equivalent
* to {@link #set(FrameOrientation3DReadOnly)}.
*
*
* If {@code orientation} is expressed in a different frame than {@code this}, then {@code this} is
* set to {@code orientation} and then transformed to be expressed in
* {@code this.getReferenceFrame()}.
*
*
* @param orientation the other orientation to copy the values from. Not modified.
*/
@Override
default void setMatchingFrame(ReferenceFrame referenceFrame, Orientation3DReadOnly orientation)
{
RotationMatrixBasics.super.set(orientation);
referenceFrame.transformFromThisToDesiredFrame(getReferenceFrame(), this);
}
/**
* Sets this frame orientation to {@code orientation}.
*
* If {@code orientation} is expressed in the frame as {@code this}, then this method is equivalent
* to {@link #set(FrameOrientation3DReadOnly)}.
*
*
* If {@code orientation} is expressed in a different frame than {@code this}, then {@code this} is
* set to {@code orientation} and then transformed to be expressed in
* {@code this.getReferenceFrame()}.
*
*
* @param orientation the other orientation to copy the values from. Not modified.
*/
@Override
default void setMatchingFrame(FrameOrientation3DReadOnly orientation)
{
set((Orientation3DReadOnly) orientation);
orientation.getReferenceFrame().transformFromThisToDesiredFrame(getReferenceFrame(), this);
}
/**
* Performs a matrix multiplication on this.
*
* this = this * other
*
*
* @param other the other matrix to multiply this by. Not modified.
* @throws ReferenceFrameMismatchException if the argument is not expressed in the same reference
* frame as {@code this}.
*/
default void multiply(FrameRotationMatrixReadOnly other)
{
checkReferenceFrameMatch(other);
RotationMatrixBasics.super.multiply(other);
}
/**
* Performs a matrix multiplication on this.
*
* this = thisT * other
*
*
* @param other the other matrix to multiply this by. Not modified.
* @throws ReferenceFrameMismatchException if the argument is not expressed in the same reference
* frame as {@code this}.
*/
default void multiplyTransposeThis(FrameRotationMatrixReadOnly other)
{
checkReferenceFrameMatch(other);
RotationMatrixBasics.super.multiplyTransposeThis(other);
}
/**
* Performs a matrix multiplication on this.
*
* this = this * otherT
*
*
* @param other the other matrix to multiply this by. Not modified.
* @throws ReferenceFrameMismatchException if the argument is not expressed in the same reference
* frame as {@code this}.
*/
default void multiplyTransposeOther(FrameRotationMatrixReadOnly other)
{
checkReferenceFrameMatch(other);
RotationMatrixBasics.super.multiplyTransposeOther(other);
}
/**
* Performs a matrix multiplication on this.
*
* this = thisT * otherT
*
*
* @param other the other matrix to multiply this by. Not modified.
* @throws ReferenceFrameMismatchException if the argument is not expressed in the same reference
* frame as {@code this}.
*/
default void multiplyTransposeBoth(FrameRotationMatrixReadOnly other)
{
checkReferenceFrameMatch(other);
RotationMatrixBasics.super.multiplyTransposeBoth(other);
}
/**
* Performs a matrix multiplication on this.
*
* this = other * this
*
*
* @param other the other matrix to multiply this by. Not modified.
* @throws ReferenceFrameMismatchException if the argument is not expressed in the same reference
* frame as {@code this}.
*/
default void preMultiply(FrameRotationMatrixReadOnly other)
{
checkReferenceFrameMatch(other);
RotationMatrixBasics.super.preMultiply(other);
}
/**
* Performs a matrix multiplication on this.
*
* this = other * thisT
*
*
* @param other the other matrix to multiply this by. Not modified.
* @throws ReferenceFrameMismatchException if the argument is not expressed in the same reference
* frame as {@code this}.
*/
default void preMultiplyTransposeThis(FrameRotationMatrixReadOnly other)
{
checkReferenceFrameMatch(other);
RotationMatrixBasics.super.preMultiplyTransposeThis(other);
}
/**
* Performs a matrix multiplication on this.
*
* this = otherT * this
*
*
* @param other the other matrix to multiply this by. Not modified.
* @throws ReferenceFrameMismatchException if the argument is not expressed in the same reference
* frame as {@code this}.
*/
default void preMultiplyTransposeOther(FrameRotationMatrixReadOnly other)
{
checkReferenceFrameMatch(other);
RotationMatrixBasics.super.preMultiplyTransposeOther(other);
}
/**
* Performs a matrix multiplication on this.
*
* this = otherT * thisT
*
*
* @param other the other matrix to multiply this by. Not modified.
* @throws ReferenceFrameMismatchException if the argument is not expressed in the same reference
* frame as {@code this}.
*/
default void preMultiplyTransposeBoth(FrameRotationMatrixReadOnly other)
{
checkReferenceFrameMatch(other);
RotationMatrixBasics.super.preMultiplyTransposeBoth(other);
}
/**
* Performs a linear interpolation in SO(3) from {@code this} to {@code rf} given the percentage
* {@code alpha}.
*
* This is equivalent to but much more computationally expensive than the Spherical Linear
* Interpolation performed with quaternions.
*
*
* @param rf the other rotation matrix used for the interpolation. Not modified.
* @param alpha the percentage used for the interpolation. A value of 0 will result in not modifying
* this rotation matrix, while a value of 1 is equivalent to setting this rotation
* matrix to {@code rf}.
* @throws ReferenceFrameMismatchException if the argument is not expressed in the same reference
* frame as {@code this}.
*/
default void interpolate(FrameRotationMatrixReadOnly rf, double alpha)
{
checkReferenceFrameMatch(rf);
RotationMatrixBasics.super.interpolate(rf, alpha);
}
/**
* Performs a linear interpolation in SO(3) from {@code r0} to {@code rf} given the percentage
* {@code alpha}.
*
* This is equivalent to but much more computationally expensive than the Spherical Linear
* Interpolation performed with quaternions.
*
*
* @param r0 the first rotation matrix used in the interpolation. Not modified.
* @param rf the second rotation matrix used in the interpolation. Not modified.
* @param alpha the percentage to use for the interpolation. A value of 0 will result in setting
* this rotation matrix to {@code r0}, while a value of 1 is equivalent to setting this
* rotation matrix to {@code rf}.
* @throws ReferenceFrameMismatchException if the frame argument is not expressed in the same
* reference frame as {@code this}.
*/
default void interpolate(RotationMatrixReadOnly r0, FrameRotationMatrixReadOnly rf, double alpha)
{
checkReferenceFrameMatch(rf);
RotationMatrixBasics.super.interpolate(r0, rf, alpha);
}
/**
* Performs a linear interpolation in SO(3) from {@code r0} to {@code rf} given the percentage
* {@code alpha}.
*
* This is equivalent to but much more computationally expensive than the Spherical Linear
* Interpolation performed with quaternions.
*
*
* @param r0 the first rotation matrix used in the interpolation. Not modified.
* @param rf the second rotation matrix used in the interpolation. Not modified.
* @param alpha the percentage to use for the interpolation. A value of 0 will result in setting
* this rotation matrix to {@code r0}, while a value of 1 is equivalent to setting this
* rotation matrix to {@code rf}.
* @throws ReferenceFrameMismatchException if the frame argument is not expressed in the same
* reference frame as {@code this}.
*/
default void interpolate(FrameRotationMatrixReadOnly r0, RotationMatrixReadOnly rf, double alpha)
{
checkReferenceFrameMatch(r0);
RotationMatrixBasics.super.interpolate(r0, rf, alpha);
}
/**
* Performs a linear interpolation in SO(3) from {@code r0} to {@code rf} given the percentage
* {@code alpha}.
*
* This is equivalent to but much more computationally expensive than the Spherical Linear
* Interpolation performed with quaternions.
*
*
* @param r0 the first rotation matrix used in the interpolation. Not modified.
* @param rf the second rotation matrix used in the interpolation. Not modified.
* @param alpha the percentage to use for the interpolation. A value of 0 will result in setting
* this rotation matrix to {@code r0}, while a value of 1 is equivalent to setting this
* rotation matrix to {@code rf}.
* @throws ReferenceFrameMismatchException if either of the frame arguments is not expressed in the
* same reference frame as {@code this}.
*/
default void interpolate(FrameRotationMatrixReadOnly r0, FrameRotationMatrixReadOnly rf, double alpha)
{
checkReferenceFrameMatch(r0, rf);
RotationMatrixBasics.super.interpolate(r0, rf, alpha);
}
}