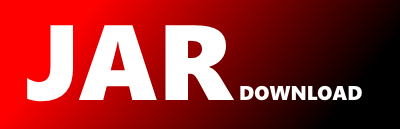
us.ihmc.euclid.referenceFrame.interfaces.FramePoint2DReadOnly Maven / Gradle / Ivy
package us.ihmc.euclid.referenceFrame.interfaces;
import us.ihmc.euclid.referenceFrame.ReferenceFrame;
import us.ihmc.euclid.referenceFrame.exceptions.ReferenceFrameMismatchException;
import us.ihmc.euclid.tuple2D.interfaces.Point2DReadOnly;
/**
* Read-only interface for a 2D point expressed in a given reference frame.
*
* In addition to representing a {@link Point2DReadOnly}, a {@link ReferenceFrame} is associated to
* a {@code FramePoint2DReadOnly}. This allows, for instance, to enforce, at runtime, that
* operations on points occur in the same coordinate system.
*
*
* Because a {@code FramePoint2DReadOnly} extends {@code Point2DReadOnly}, it is compatible with
* methods only requiring {@code Point2DReadOnly}. However, these methods do NOT assert that the
* operation occur in the proper coordinate system. Use this feature carefully and always prefer
* using methods requiring {@code FramePoint2DReadOnly}.
*
*/
public interface FramePoint2DReadOnly extends Point2DReadOnly, FrameTuple2DReadOnly
{
/**
* Calculates and returns the distance between this frame point and {@code other}.
*
* @param other the other frame point used to measure the distance. Not modified.
* @return the distance between the two frame points.
* @throws ReferenceFrameMismatchException if {@code other} is not expressed in the same frame as
* {@code this}.
*/
default double distance(FramePoint2DReadOnly other)
{
checkReferenceFrameMatch(other);
return Point2DReadOnly.super.distance(other);
}
/**
* Calculates and returns the square of the distance between this frame point and {@code other}.
*
* This method is usually preferred over {@link #distance(FramePoint2DReadOnly)} when calculation
* speed matters and knowledge of the actual distance does not, i.e. when comparing distances
* between several pairs of frame points.
*
*
* @param other the other frame point used to measure the square of the distance. Not modified.
* @return the square of the distance between the two frame points.
* @throws ReferenceFrameMismatchException if {@code other} is not expressed in the same frame as
* {@code this}.
*/
default double distanceSquared(FramePoint2DReadOnly other)
{
checkReferenceFrameMatch(other);
return Point2DReadOnly.super.distanceSquared(other);
}
/**
* Calculates and returns the distance between this frame point and {@code framePoint3DReadOnly} in
* the XY-plane.
*
* Effectively, this calculates the distance as follows:
* dxy = √((this.x - framePoint3DReadOnly.x)2 + (this.y -
* framePoint3DReadOnly.y)2)
*
*
* @param framePoint3DReadOnly the other frame point used to measure the distance.
* @return the distance between the two frame points in the XY-plane.
* @throws ReferenceFrameMismatchException if {@code framePoint3DReadOnly} is not expressed in the
* same frame as {@code this}.
*/
default double distanceXY(FramePoint3DReadOnly framePoint3DReadOnly)
{
checkReferenceFrameMatch(framePoint3DReadOnly);
return Point2DReadOnly.super.distanceXY(framePoint3DReadOnly);
}
/**
* Calculates and returns the square of the distance between this frame point and
* {@code framePoint3DReadOnly} in the XY-plane.
*
* Effectively, this calculates the distance squared as follows:
* dxy2 = (this.x - framePoint3DReadOnly.x)2 + (this.y -
* framePoint3DReadOnly.y)2
*
*
* This method is usually preferred over {@link #distanceXY(FramePoint3DReadOnly)} when calculation
* speed matters and knowledge of the actual distance does not, i.e. when comparing distances
* between several pairs of points.
*
*
* @param framePoint3DReadOnly the frame other point used to measure the square of the distance.
* @return the square of the distance between the two frame points in the XY-plane.
* @throws ReferenceFrameMismatchException if {@code framePoint3DReadOnly} is not expressed in the
* same frame as {@code this}.
*/
default double distanceXYSquared(FramePoint3DReadOnly framePoint3DReadOnly)
{
checkReferenceFrameMatch(framePoint3DReadOnly);
return Point2DReadOnly.super.distanceXYSquared(framePoint3DReadOnly);
}
}