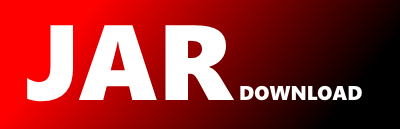
us.ihmc.euclid.referenceFrame.interfaces.FramePoint3DReadOnly Maven / Gradle / Ivy
package us.ihmc.euclid.referenceFrame.interfaces;
import us.ihmc.euclid.referenceFrame.ReferenceFrame;
import us.ihmc.euclid.referenceFrame.exceptions.ReferenceFrameMismatchException;
import us.ihmc.euclid.tuple3D.interfaces.Point3DReadOnly;
/**
* Read-only interface for a 3D point expressed in a given reference frame.
*
* In addition to representing a {@link Point3DReadOnly}, a {@link ReferenceFrame} is associated to
* a {@code FramePoint3DReadOnly}. This allows, for instance, to enforce, at runtime, that
* operations on points occur in the same coordinate system.
*
*
* Because a {@code FramePoint3DReadOnly} extends {@code Point3DReadOnly}, it is compatible with
* methods only requiring {@code Point3DReadOnly}. However, these methods do NOT assert that the
* operation occur in the proper coordinate system. Use this feature carefully and always prefer
* using methods requiring {@code FramePoint3DReadOnly}.
*
*/
public interface FramePoint3DReadOnly extends Point3DReadOnly, FrameTuple3DReadOnly
{
/**
* Calculates and returns the distance between this frame point and {@code other}.
*
* @param other the other frame point used to measure the distance.
* @return the distance between the two frame points.
* @throws ReferenceFrameMismatchException if {@code other} is not expressed in the same frame as
* {@code this}.
*/
default double distance(FramePoint3DReadOnly other)
{
checkReferenceFrameMatch(other);
return Point3DReadOnly.super.distance(other);
}
/**
* Calculates and returns the square of the distance between this frame point and {@code other}.
*
* This method is usually preferred over {@link #distance(FramePoint3DReadOnly)} when calculation
* speed matters and knowledge of the actual distance does not, i.e. when comparing distances
* between several pairs of frame points.
*
*
* @param other the other frame point used to measure the square of the distance.
* @return the square of the distance between the two frame points.
* @throws ReferenceFrameMismatchException if {@code other} is not expressed in the same frame as
* {@code this}.
*/
default double distanceSquared(FramePoint3DReadOnly other)
{
checkReferenceFrameMatch(other);
return Point3DReadOnly.super.distanceSquared(other);
}
/**
* Calculates and returns the distance between this frame point and {@code other} in the XY-plane.
*
* Effectively, this calculates the distance as follows:
* dxy = √((this.x - other.x)2 + (this.y - other.y)2)
*
*
* @param other the other frame point used to measure the distance.
* @return the distance between the two frame points in the XY-plane.
* @throws ReferenceFrameMismatchException if {@code other} is not expressed in the same frame as
* {@code this}.
*/
default double distanceXY(FramePoint3DReadOnly other)
{
checkReferenceFrameMatch(other);
return Point3DReadOnly.super.distanceXY(other);
}
/**
* Calculates and returns the square of the distance between this frame point and {@code other} in
* the XY-plane.
*
* Effectively, this calculates the distance squared as follows:
* dxy2 = (this.x - other.x)2 + (this.y - other.y)2
*
*
* This method is usually preferred over {@link #distanceXY(FramePoint3DReadOnly)} when calculation
* speed matters and knowledge of the actual distance does not, i.e. when comparing distances
* between several pairs of frame points.
*
*
* @param other the other frame point used to measure the square of the distance.
* @return the square of the distance between the two frame points in the XY-plane.
* @throws ReferenceFrameMismatchException if {@code other} is not expressed in the same frame as
* {@code this}.
*/
default double distanceXYSquared(FramePoint3DReadOnly other)
{
checkReferenceFrameMatch(other);
return Point3DReadOnly.super.distanceXYSquared(other);
}
/**
* Calculates and returns the distance between this frame point and {@code framePoint2DReadOnly} in
* the XY-plane.
*
* Effectively, this calculates the distance as follows:
* dxy = √((this.x - framePoint2DReadOnly.x)2 + (this.y -
* framePoint2DReadOnly.y)2)
*
*
* @param framePoint2DReadOnly the other frame point used to measure the distance.
* @return the distance between the two frame points in the XY-plane.
* @throws ReferenceFrameMismatchException if {@code framePoint2DReadOnly} is not expressed in the
* same frame as {@code this}.
*/
default double distanceXY(FramePoint2DReadOnly framePoint2DReadOnly)
{
checkReferenceFrameMatch(framePoint2DReadOnly);
return Point3DReadOnly.super.distanceXY(framePoint2DReadOnly);
}
/**
* Calculates and returns the square of the distance between this frame point and
* {@code framePoint2DReadOnly} in the XY-plane.
*
* Effectively, this calculates the distance squared as follows:
* dxy2 = (this.x - framePoint2DReadOnly.x)2 + (this.y -
* framePoint2DReadOnly.y)2
*
*
* This method is usually preferred over {@link #distanceXY(FramePoint2DReadOnly)} when calculation
* speed matters and knowledge of the actual distance does not, i.e. when comparing distances
* between several pairs of points.
*
*
* @param framePoint2DReadOnly the other frame point used to measure the square of the distance.
* @return the square of the distance between the two frame points in the XY-plane.
* @throws ReferenceFrameMismatchException if {@code framePoint2DReadOnly} is not expressed in the
* same frame as {@code this}.
*/
default double distanceXYSquared(FramePoint2DReadOnly framePoint2DReadOnly)
{
checkReferenceFrameMatch(framePoint2DReadOnly);
return Point3DReadOnly.super.distanceXYSquared(framePoint2DReadOnly);
}
}