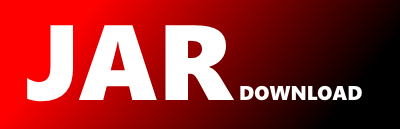
us.ihmc.convexOptimization.quadraticProgram.ActiveSetQPSolver Maven / Gradle / Ivy
package us.ihmc.convexOptimization.quadraticProgram;
import org.ejml.data.DMatrix;
import org.ejml.data.DMatrixRMaj;
import us.ihmc.matrixlib.NativeMatrix;
/**
* General interface for a QP solver using under the hood an active set approach, see:
*
* - General reference for quadratic programming:
* Wikipedia QP.
*
- Active-set method: Wikipedia
* Active-set.
*
*
* Problem formulation:
*
*
* min f(x) = 0.5 * xT H x + fTx + c
* s.t.
* Ain x ≤ bin
* Aeq x = beq
* x ≥ xmin
* x ≤ xmax
*
*
* where:
*
* - N, Min, and Meq are the number of variables, the number of inequality
* constraints, and the number of equality constraints, respectively.
*
- x is the N-by-1 variable vector to find the solution for.
*
- H is the N-by-N (typically positive semi-definite, preferably positive definite)
* Hessian matrix.
*
- f is the N-by-1 gradient vector.
*
- c is a scalar constant, that is typically equal to zero.
*
- Ain and bin are respectively a Min-by-N
* matrix and Min-by-1 vector used to formulate Min inequality constraints.
*
- Aeq and beq are respectively a Meq-by-N
* matrix and Meq-by-1 vector used to formulate Meq equality constraints.
*
- xmin is the N-by-1 vector used for enforcing a lower bound on each
* element of x.
*
- xmax is the N-by-1 vector used for enforcing an upper bound on each
* element of x.
*
*
*/
public interface ActiveSetQPSolver
{
/**
* Threshold used for triggering changes to the active-set. Internal usage is subject to the
* implementation of the solver.
*
* @param convergenceThreshold the threshold for modifying the internal active-set when solving.
*/
void setConvergenceThreshold(double convergenceThreshold);
/**
* Sets the maximum number of times the active-set can be modified before considering the problem as
* unsolvable.
*
* @param maxNumberOfIterations the upper limit on the number of iterations before failing when
* solving.
*/
void setMaxNumberOfIterations(int maxNumberOfIterations);
/**
* Sets whether to use a warm-start or not.
*
* When warm-start is enabled, the last active-set from the last solve is used as an initial guess
* for the next solve initial active-set. This cuts down considerably the number of iterations when
* the active-set from one solve to the next is expected to not change or only change a little.
*
*
* @param useWarmStart {@code true} to use warm-start, {@code false} otherwise.
*/
void setUseWarmStart(boolean useWarmStart);
/**
* Indicates that for the next solve, the active-set should be reset, i.e. not start from the last
* solve's active-set.
*
* This method is only effective when warm-start is enabled.
*
*/
void resetActiveSet();
/**
* Clears the internal data but does not reset the active-set.
*/
void clear();
/**
* Sets the N-by-1 vector used to enforce a lower bound on the problem's variables:
*
*
* x ≥ xmin
*
*
* where N is the number of variables in x.
*
* @param xMin the lower bound for the problem's variables. Not modified.
* @see ActiveSetQPSolver
*/
void setLowerBounds(DMatrix xMin);
/**
* Sets the N-by-1 vector used to enforce an upper bound on the problem's variables:
*
*
* x ≥ xmax
*
*
* where N is the number of variables in x.
*
* @param xMax the upper bound for the problem's variables. Not modified.
* @see ActiveSetQPSolver
*/
void setUpperBounds(DMatrix xMax);
/**
* Sets the N-by-1 vectors used to enforce a lower and upper bounds on the problem's variables:
*
*
* x ≥ xmin
* x ≥ xmax
*
*
* where N is the number of variables in x.
*
* @param xMin the lower bound for the problem's variables. Not modified.
* @param xMax the upper bound for the problem's variables. Not modified.
* @see ActiveSetQPSolver
*/
default void setVariableBounds(DMatrix xMin, DMatrix xMax)
{
setLowerBounds(xMin);
setUpperBounds(xMax);
}
/**
* Configures the function to minimize:
*
*
* f(x) = 0.5 * xT H x + fTx
*
*
* where x is the N-by-1 vector of variables to solve for.
*
* @param H is the N-by-N (typically positive semi-definite, preferably positive definite) Hessian
* matrix. Not modified.
* @param f is the N-by-1 gradient vector.
* @see ActiveSetQPSolver
*/
default void setQuadraticCostFunction(DMatrix H, DMatrix f)
{
setQuadraticCostFunction(H, f, 0.0);
}
/**
* Configures the function to minimize:
*
*
* f(x) = 0.5 * xT H x + fTx + c
*
*
* where x is the N-by-1 vector of variables to solve for.
*
* @param H is the N-by-N (typically positive semi-definite, preferably positive definite) Hessian
* matrix. Not modified.
* @param f is the N-by-1 gradient vector. Not modified.
* @param c is a scalar constant, that is typically equal to zero.
* @see ActiveSetQPSolver
*/
void setQuadraticCostFunction(DMatrix H, DMatrix f, double c);
/**
* Configures the linear equality constraints:
*
*
* Aeq x = beq
*
*
* where:
*
* - x is the N-by-1 vector of variables to solve for.
*
- Meq is the number of equality constraints.
*
*
* @param Aeq is a Meq-by-N matrix. Not modified.
* @param beq is a Meq-by-1 vector. Not modified.
* @see ActiveSetQPSolver
*/
void setLinearEqualityConstraints(DMatrix Aeq, DMatrix beq);
/**
* Configures the linear inequality constraints:
*
*
* Ain x ≤ bin
*
*
* where:
*
* - x is the N-by-1 vector of variables to solve for.
*
- Min is the number of inequality constraints.
*
*
* @param Ain is a Min-by-N matrix. Not modified.
* @param bin is a Min-by-1 vector. Not modified.
* @see ActiveSetQPSolver
*/
void setLinearInequalityConstraints(DMatrix Ain, DMatrix bin);
/**
* With the problem previously formulated, solves the objective function for {@code x}:
*
*
* min f(x) = 0.5 * xT H x + fTx + c
* s.t.
* Ain x ≤ bin
* Aeq x = beq
* x ≥ xmin
* x ≤ xmax
*
*
* where:
*
* - N, Min, and Meq are the number of variables, the number of inequality
* constraints, and the number of equality constraints, respectively.
*
- x is the N-by-1 variable vector to find the solution for.
*
- H is the N-by-N (typically positive semi-definite, preferably positive definite)
* Hessian matrix.
*
- f is the N-by-1 gradient vector.
*
- c is a scalar constant, that is typically equal to zero.
*
- Ain and bin are respectively a Min-by-N
* matrix and Min-by-1 vector used to formulate Min inequality constraints.
*
- Aeq and beq are respectively a Meq-by-N
* matrix and Meq-by-1 vector used to formulate Meq equality constraints.
*
- xmin is the N-by-1 vector used for enforcing a lower bound on each
* element of x.
*
- xmax is the N-by-1 vector used for enforcing an upper bound on each
* element of x.
*
*
* If this method fails, {@code xToPack} is filled with {@link Double#NaN}.
*
*
* @param xToPack the N-by-1 vector in which the solution is stored, or filled with
* {@link Double#NaN} if this method failed, i.e. the maximum number of iteration has
* been reached before finding a solution to the problem. Modified.
* @return the number of iterations it took to find the solution. An iteration is defined as a
* change in the active-set.
* @see #setQuadraticCostFunction(DMatrix, DMatrix)
* @see #setLinearInequalityConstraints(DMatrix, DMatrix)
* @see #setLinearEqualityConstraints(DMatrix, DMatrix)
* @see #setLowerBounds(DMatrix)
* @see #setUpperBounds(DMatrix)
* @see ActiveSetQPSolver
*/
int solve(DMatrix xToPack);
/**
* Calculates the cost from the objective function given value for {@code x}:
*
*
* f(x) = 0.5 * xT H x + fTx
*
*
* @param x is a N-by-1 vector. Not modified.
* @return the cost value.
*/
double getObjectiveCost(DMatrixRMaj x);
/**
* This is for advanced users or testing only.
*
* Packs the Lagrange multipliers corresponding to the linear equality constraints.
*
*
* @param multipliersMatrixToPack the Meq-by-1 vector in which the Lagrange multipliers
* resulting from the last solve are stored, where Meq is
* the number of equality constraints. Modified.
*/
void getLagrangeEqualityConstraintMultipliers(DMatrixRMaj multipliersMatrixToPack);
/**
* This is for advanced users or testing only.
*
* Packs the Lagrange multipliers corresponding to the linear inequality constraints.
*
*
* @param multipliersMatrixToPack the M*in-by-1 vector in which the Lagrange multipliers
* resulting from the last solve are stored, where M*in is
* the number of active inequality constraints. Modified.
*/
void getLagrangeInequalityConstraintMultipliers(DMatrixRMaj multipliersMatrixToPack);
/**
* This is for advanced users or testing only.
*
* Packs the Lagrange multipliers corresponding to the lower bound constraints.
*
*
* @param multipliersMatrixToPack the N*-by-1 vector in which the Lagrange multipliers resulting
* from the last solve are stored, where N* is the number of active
* lower bound constraints. Modified.
*/
void getLagrangeLowerBoundsMultipliers(DMatrixRMaj multipliersMatrixToPack);
/**
* This is for advanced users or testing only.
*
* Packs the Lagrange multipliers corresponding to the upper bound constraints.
*
*
* @param multipliersMatrixToPack the N*-by-1 vector in which the Lagrange multipliers resulting
* from the last solve are stored, where N* is the number of active
* upper bound constraints. Modified.
*/
void getLagrangeUpperBoundsMultipliers(DMatrixRMaj multipliersMatrixToPack);
}