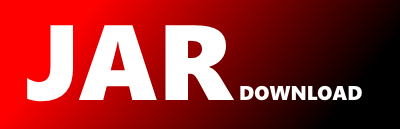
com.eprosima.xmlschemas.fastrtps_profiles.ObjectFactory Maven / Gradle / Ivy
package com.eprosima.xmlschemas.fastrtps_profiles;
import java.util.List;
import javax.xml.namespace.QName;
import jakarta.xml.bind.JAXBElement;
import jakarta.xml.bind.annotation.XmlElementDecl;
import jakarta.xml.bind.annotation.XmlRegistry;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.eprosima.xmlschemas.fastrtps_profiles package.
* An ObjectFactory allows you to programmatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private static final QName _Profiles_QNAME = new QName("http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", "profiles");
private static final QName _Types_QNAME = new QName("http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", "types");
private static final QName _Log_QNAME = new QName("http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", "log");
private static final QName _LibrarySettings_QNAME = new QName("http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", "library_settings");
private static final QName _DurationTypeSec_QNAME = new QName("http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", "sec");
private static final QName _DurationTypeNanosec_QNAME = new QName("http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", "nanosec");
private static final QName _TransportDescriptorTypeInterfaceWhiteListAddress_QNAME = new QName("http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", "address");
private static final QName _TransportDescriptorTypeInterfaceWhiteListInterface_QNAME = new QName("http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", "interface");
private static final QName _RemoteServerAttributesTypeMetatrafficUnicastLocatorList_QNAME = new QName("http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", "metatrafficUnicastLocatorList");
private static final QName _RemoteServerAttributesTypeMetatrafficMulticastLocatorList_QNAME = new QName("http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", "metatrafficMulticastLocatorList");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.eprosima.xmlschemas.fastrtps_profiles
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link PartitionQosPolicyType }
*
* @return
* the new instance of {@link PartitionQosPolicyType }
*/
public PartitionQosPolicyType createPartitionQosPolicyType() {
return new PartitionQosPolicyType();
}
/**
* Create an instance of {@link DataSharingQosPolicyType }
*
* @return
* the new instance of {@link DataSharingQosPolicyType }
*/
public DataSharingQosPolicyType createDataSharingQosPolicyType() {
return new DataSharingQosPolicyType();
}
/**
* Create an instance of {@link PropertyPolicyType }
*
* @return
* the new instance of {@link PropertyPolicyType }
*/
public PropertyPolicyType createPropertyPolicyType() {
return new PropertyPolicyType();
}
/**
* Create an instance of {@link LocatorListType }
*
* @return
* the new instance of {@link LocatorListType }
*/
public LocatorListType createLocatorListType() {
return new LocatorListType();
}
/**
* Create an instance of {@link TlsConfigType }
*
* @return
* the new instance of {@link TlsConfigType }
*/
public TlsConfigType createTlsConfigType() {
return new TlsConfigType();
}
/**
* Create an instance of {@link BlocklistType }
*
* @return
* the new instance of {@link BlocklistType }
*/
public BlocklistType createBlocklistType() {
return new BlocklistType();
}
/**
* Create an instance of {@link AllowlistType }
*
* @return
* the new instance of {@link AllowlistType }
*/
public AllowlistType createAllowlistType() {
return new AllowlistType();
}
/**
* Create an instance of {@link TransportDescriptorType }
*
* @return
* the new instance of {@link TransportDescriptorType }
*/
public TransportDescriptorType createTransportDescriptorType() {
return new TransportDescriptorType();
}
/**
* Create an instance of {@link RtpsParticipantAllocationAttributesType }
*
* @return
* the new instance of {@link RtpsParticipantAllocationAttributesType }
*/
public RtpsParticipantAllocationAttributesType createRtpsParticipantAllocationAttributesType() {
return new RtpsParticipantAllocationAttributesType();
}
/**
* Create an instance of {@link BitmaskDcl }
*
* @return
* the new instance of {@link BitmaskDcl }
*/
public BitmaskDcl createBitmaskDcl() {
return new BitmaskDcl();
}
/**
* Create an instance of {@link BitsetDcl }
*
* @return
* the new instance of {@link BitsetDcl }
*/
public BitsetDcl createBitsetDcl() {
return new BitsetDcl();
}
/**
* Create an instance of {@link UnionDcl }
*
* @return
* the new instance of {@link UnionDcl }
*/
public UnionDcl createUnionDcl() {
return new UnionDcl();
}
/**
* Create an instance of {@link UnionDcl.Case }
*
* @return
* the new instance of {@link UnionDcl.Case }
*/
public UnionDcl.Case createUnionDclCase() {
return new UnionDcl.Case();
}
/**
* Create an instance of {@link EnumDcl }
*
* @return
* the new instance of {@link EnumDcl }
*/
public EnumDcl createEnumDcl() {
return new EnumDcl();
}
/**
* Create an instance of {@link TopicElementType }
*
* @return
* the new instance of {@link TopicElementType }
*/
public TopicElementType createTopicElementType() {
return new TopicElementType();
}
/**
* Create an instance of {@link TopicProfileType }
*
* @return
* the new instance of {@link TopicProfileType }
*/
public TopicProfileType createTopicProfileType() {
return new TopicProfileType();
}
/**
* Create an instance of {@link ParticipantProfileType }
*
* @return
* the new instance of {@link ParticipantProfileType }
*/
public ParticipantProfileType createParticipantProfileType() {
return new ParticipantProfileType();
}
/**
* Create an instance of {@link ParticipantProfileType.Rtps }
*
* @return
* the new instance of {@link ParticipantProfileType.Rtps }
*/
public ParticipantProfileType.Rtps createParticipantProfileTypeRtps() {
return new ParticipantProfileType.Rtps();
}
/**
* Create an instance of {@link Dds }
*
* @return
* the new instance of {@link Dds }
*/
public Dds createDds() {
return new Dds();
}
/**
* Create an instance of {@link ProfilesType }
*
* @return
* the new instance of {@link ProfilesType }
*/
public ProfilesType createProfilesType() {
return new ProfilesType();
}
/**
* Create an instance of {@link TypesType }
*
* @return
* the new instance of {@link TypesType }
*/
public TypesType createTypesType() {
return new TypesType();
}
/**
* Create an instance of {@link LogType }
*
* @return
* the new instance of {@link LogType }
*/
public LogType createLogType() {
return new LogType();
}
/**
* Create an instance of {@link LibrarySettingsType }
*
* @return
* the new instance of {@link LibrarySettingsType }
*/
public LibrarySettingsType createLibrarySettingsType() {
return new LibrarySettingsType();
}
/**
* Create an instance of {@link DomainParticipantFactoryProfileType }
*
* @return
* the new instance of {@link DomainParticipantFactoryProfileType }
*/
public DomainParticipantFactoryProfileType createDomainParticipantFactoryProfileType() {
return new DomainParticipantFactoryProfileType();
}
/**
* Create an instance of {@link BuiltinTransportsType }
*
* @return
* the new instance of {@link BuiltinTransportsType }
*/
public BuiltinTransportsType createBuiltinTransportsType() {
return new BuiltinTransportsType();
}
/**
* Create an instance of {@link PublisherProfileType }
*
* @return
* the new instance of {@link PublisherProfileType }
*/
public PublisherProfileType createPublisherProfileType() {
return new PublisherProfileType();
}
/**
* Create an instance of {@link SubscriberProfileType }
*
* @return
* the new instance of {@link SubscriberProfileType }
*/
public SubscriberProfileType createSubscriberProfileType() {
return new SubscriberProfileType();
}
/**
* Create an instance of {@link TransportDescriptorListType }
*
* @return
* the new instance of {@link TransportDescriptorListType }
*/
public TransportDescriptorListType createTransportDescriptorListType() {
return new TransportDescriptorListType();
}
/**
* Create an instance of {@link TypeType }
*
* @return
* the new instance of {@link TypeType }
*/
public TypeType createTypeType() {
return new TypeType();
}
/**
* Create an instance of {@link TypedefDcl }
*
* @return
* the new instance of {@link TypedefDcl }
*/
public TypedefDcl createTypedefDcl() {
return new TypedefDcl();
}
/**
* Create an instance of {@link StructDcl }
*
* @return
* the new instance of {@link StructDcl }
*/
public StructDcl createStructDcl() {
return new StructDcl();
}
/**
* Create an instance of {@link MemberDcl }
*
* @return
* the new instance of {@link MemberDcl }
*/
public MemberDcl createMemberDcl() {
return new MemberDcl();
}
/**
* Create an instance of {@link LogConsumerType }
*
* @return
* the new instance of {@link LogConsumerType }
*/
public LogConsumerType createLogConsumerType() {
return new LogConsumerType();
}
/**
* Create an instance of {@link LogConsumerPropertyType }
*
* @return
* the new instance of {@link LogConsumerPropertyType }
*/
public LogConsumerPropertyType createLogConsumerPropertyType() {
return new LogConsumerPropertyType();
}
/**
* Create an instance of {@link BuiltinAttributesType }
*
* @return
* the new instance of {@link BuiltinAttributesType }
*/
public BuiltinAttributesType createBuiltinAttributesType() {
return new BuiltinAttributesType();
}
/**
* Create an instance of {@link RemoteServerAttributesType }
*
* @return
* the new instance of {@link RemoteServerAttributesType }
*/
public RemoteServerAttributesType createRemoteServerAttributesType() {
return new RemoteServerAttributesType();
}
/**
* Create an instance of {@link DiscoveryServersListType }
*
* @return
* the new instance of {@link DiscoveryServersListType }
*/
public DiscoveryServersListType createDiscoveryServersListType() {
return new DiscoveryServersListType();
}
/**
* Create an instance of {@link SimpleEDPType }
*
* @return
* the new instance of {@link SimpleEDPType }
*/
public SimpleEDPType createSimpleEDPType() {
return new SimpleEDPType();
}
/**
* Create an instance of {@link InitialAnnouncementsType }
*
* @return
* the new instance of {@link InitialAnnouncementsType }
*/
public InitialAnnouncementsType createInitialAnnouncementsType() {
return new InitialAnnouncementsType();
}
/**
* Create an instance of {@link DiscoverySettingsType }
*
* @return
* the new instance of {@link DiscoverySettingsType }
*/
public DiscoverySettingsType createDiscoverySettingsType() {
return new DiscoverySettingsType();
}
/**
* Create an instance of {@link TypelookupSettingsType }
*
* @return
* the new instance of {@link TypelookupSettingsType }
*/
public TypelookupSettingsType createTypelookupSettingsType() {
return new TypelookupSettingsType();
}
/**
* Create an instance of {@link PortType }
*
* @return
* the new instance of {@link PortType }
*/
public PortType createPortType() {
return new PortType();
}
/**
* Create an instance of {@link WriterTimesType }
*
* @return
* the new instance of {@link WriterTimesType }
*/
public WriterTimesType createWriterTimesType() {
return new WriterTimesType();
}
/**
* Create an instance of {@link ReaderTimesType }
*
* @return
* the new instance of {@link ReaderTimesType }
*/
public ReaderTimesType createReaderTimesType() {
return new ReaderTimesType();
}
/**
* Create an instance of {@link InterfacesType }
*
* @return
* the new instance of {@link InterfacesType }
*/
public InterfacesType createInterfacesType() {
return new InterfacesType();
}
/**
* Create an instance of {@link ExternalLocatorListType }
*
* @return
* the new instance of {@link ExternalLocatorListType }
*/
public ExternalLocatorListType createExternalLocatorListType() {
return new ExternalLocatorListType();
}
/**
* Create an instance of {@link DurationType }
*
* @return
* the new instance of {@link DurationType }
*/
public DurationType createDurationType() {
return new DurationType();
}
/**
* Create an instance of {@link DomainParticipantFactoryQosPoliciesType }
*
* @return
* the new instance of {@link DomainParticipantFactoryQosPoliciesType }
*/
public DomainParticipantFactoryQosPoliciesType createDomainParticipantFactoryQosPoliciesType() {
return new DomainParticipantFactoryQosPoliciesType();
}
/**
* Create an instance of {@link DataWriterQosPoliciesType }
*
* @return
* the new instance of {@link DataWriterQosPoliciesType }
*/
public DataWriterQosPoliciesType createDataWriterQosPoliciesType() {
return new DataWriterQosPoliciesType();
}
/**
* Create an instance of {@link DataReaderQosPoliciesType }
*
* @return
* the new instance of {@link DataReaderQosPoliciesType }
*/
public DataReaderQosPoliciesType createDataReaderQosPoliciesType() {
return new DataReaderQosPoliciesType();
}
/**
* Create an instance of {@link AllocationConfigType }
*
* @return
* the new instance of {@link AllocationConfigType }
*/
public AllocationConfigType createAllocationConfigType() {
return new AllocationConfigType();
}
/**
* Create an instance of {@link Udpv4LocatorType }
*
* @return
* the new instance of {@link Udpv4LocatorType }
*/
public Udpv4LocatorType createUdpv4LocatorType() {
return new Udpv4LocatorType();
}
/**
* Create an instance of {@link Udpv6LocatorType }
*
* @return
* the new instance of {@link Udpv6LocatorType }
*/
public Udpv6LocatorType createUdpv6LocatorType() {
return new Udpv6LocatorType();
}
/**
* Create an instance of {@link Tcpv4LocatorType }
*
* @return
* the new instance of {@link Tcpv4LocatorType }
*/
public Tcpv4LocatorType createTcpv4LocatorType() {
return new Tcpv4LocatorType();
}
/**
* Create an instance of {@link Tcpv6LocatorType }
*
* @return
* the new instance of {@link Tcpv6LocatorType }
*/
public Tcpv6LocatorType createTcpv6LocatorType() {
return new Tcpv6LocatorType();
}
/**
* Create an instance of {@link Udpv4ExternalLocatorType }
*
* @return
* the new instance of {@link Udpv4ExternalLocatorType }
*/
public Udpv4ExternalLocatorType createUdpv4ExternalLocatorType() {
return new Udpv4ExternalLocatorType();
}
/**
* Create an instance of {@link Udpv6ExternalLocatorType }
*
* @return
* the new instance of {@link Udpv6ExternalLocatorType }
*/
public Udpv6ExternalLocatorType createUdpv6ExternalLocatorType() {
return new Udpv6ExternalLocatorType();
}
/**
* Create an instance of {@link DeadlineQosPolicyType }
*
* @return
* the new instance of {@link DeadlineQosPolicyType }
*/
public DeadlineQosPolicyType createDeadlineQosPolicyType() {
return new DeadlineQosPolicyType();
}
/**
* Create an instance of {@link DestinationOrderQosPolicyType }
*
* @return
* the new instance of {@link DestinationOrderQosPolicyType }
*/
public DestinationOrderQosPolicyType createDestinationOrderQosPolicyType() {
return new DestinationOrderQosPolicyType();
}
/**
* Create an instance of {@link DisablePositiveAcksQosPolicyType }
*
* @return
* the new instance of {@link DisablePositiveAcksQosPolicyType }
*/
public DisablePositiveAcksQosPolicyType createDisablePositiveAcksQosPolicyType() {
return new DisablePositiveAcksQosPolicyType();
}
/**
* Create an instance of {@link DurabilityQosPolicyType }
*
* @return
* the new instance of {@link DurabilityQosPolicyType }
*/
public DurabilityQosPolicyType createDurabilityQosPolicyType() {
return new DurabilityQosPolicyType();
}
/**
* Create an instance of {@link DurabilityServiceQosPolicyType }
*
* @return
* the new instance of {@link DurabilityServiceQosPolicyType }
*/
public DurabilityServiceQosPolicyType createDurabilityServiceQosPolicyType() {
return new DurabilityServiceQosPolicyType();
}
/**
* Create an instance of {@link EntityFactoryQosPolicyType }
*
* @return
* the new instance of {@link EntityFactoryQosPolicyType }
*/
public EntityFactoryQosPolicyType createEntityFactoryQosPolicyType() {
return new EntityFactoryQosPolicyType();
}
/**
* Create an instance of {@link HistoryQosPolicyType }
*
* @return
* the new instance of {@link HistoryQosPolicyType }
*/
public HistoryQosPolicyType createHistoryQosPolicyType() {
return new HistoryQosPolicyType();
}
/**
* Create an instance of {@link LatencyBudgetQosPolicyType }
*
* @return
* the new instance of {@link LatencyBudgetQosPolicyType }
*/
public LatencyBudgetQosPolicyType createLatencyBudgetQosPolicyType() {
return new LatencyBudgetQosPolicyType();
}
/**
* Create an instance of {@link LifespanQosPolicyType }
*
* @return
* the new instance of {@link LifespanQosPolicyType }
*/
public LifespanQosPolicyType createLifespanQosPolicyType() {
return new LifespanQosPolicyType();
}
/**
* Create an instance of {@link LivelinessQosPolicyType }
*
* @return
* the new instance of {@link LivelinessQosPolicyType }
*/
public LivelinessQosPolicyType createLivelinessQosPolicyType() {
return new LivelinessQosPolicyType();
}
/**
* Create an instance of {@link OwnershipQosPolicyType }
*
* @return
* the new instance of {@link OwnershipQosPolicyType }
*/
public OwnershipQosPolicyType createOwnershipQosPolicyType() {
return new OwnershipQosPolicyType();
}
/**
* Create an instance of {@link OwnershipStrengthQosPolicyType }
*
* @return
* the new instance of {@link OwnershipStrengthQosPolicyType }
*/
public OwnershipStrengthQosPolicyType createOwnershipStrengthQosPolicyType() {
return new OwnershipStrengthQosPolicyType();
}
/**
* Create an instance of {@link PresentationQosPolicyType }
*
* @return
* the new instance of {@link PresentationQosPolicyType }
*/
public PresentationQosPolicyType createPresentationQosPolicyType() {
return new PresentationQosPolicyType();
}
/**
* Create an instance of {@link PublishModeQosPolicyType }
*
* @return
* the new instance of {@link PublishModeQosPolicyType }
*/
public PublishModeQosPolicyType createPublishModeQosPolicyType() {
return new PublishModeQosPolicyType();
}
/**
* Create an instance of {@link ReliabilityQosPolicyType }
*
* @return
* the new instance of {@link ReliabilityQosPolicyType }
*/
public ReliabilityQosPolicyType createReliabilityQosPolicyType() {
return new ReliabilityQosPolicyType();
}
/**
* Create an instance of {@link TimeBasedFilterQosPolicyType }
*
* @return
* the new instance of {@link TimeBasedFilterQosPolicyType }
*/
public TimeBasedFilterQosPolicyType createTimeBasedFilterQosPolicyType() {
return new TimeBasedFilterQosPolicyType();
}
/**
* Create an instance of {@link OctectVectorQosPolicyType }
*
* @return
* the new instance of {@link OctectVectorQosPolicyType }
*/
public OctectVectorQosPolicyType createOctectVectorQosPolicyType() {
return new OctectVectorQosPolicyType();
}
/**
* Create an instance of {@link PropertyType }
*
* @return
* the new instance of {@link PropertyType }
*/
public PropertyType createPropertyType() {
return new PropertyType();
}
/**
* Create an instance of {@link BinaryPropertyType }
*
* @return
* the new instance of {@link BinaryPropertyType }
*/
public BinaryPropertyType createBinaryPropertyType() {
return new BinaryPropertyType();
}
/**
* Create an instance of {@link FlowControllerDescriptorListType }
*
* @return
* the new instance of {@link FlowControllerDescriptorListType }
*/
public FlowControllerDescriptorListType createFlowControllerDescriptorListType() {
return new FlowControllerDescriptorListType();
}
/**
* Create an instance of {@link FlowControllerDescriptorType }
*
* @return
* the new instance of {@link FlowControllerDescriptorType }
*/
public FlowControllerDescriptorType createFlowControllerDescriptorType() {
return new FlowControllerDescriptorType();
}
/**
* Create an instance of {@link ThreadSettingsType }
*
* @return
* the new instance of {@link ThreadSettingsType }
*/
public ThreadSettingsType createThreadSettingsType() {
return new ThreadSettingsType();
}
/**
* Create an instance of {@link ThreadSettingsWithPortType }
*
* @return
* the new instance of {@link ThreadSettingsWithPortType }
*/
public ThreadSettingsWithPortType createThreadSettingsWithPortType() {
return new ThreadSettingsWithPortType();
}
/**
* Create an instance of {@link ReceptionThreadsListType }
*
* @return
* the new instance of {@link ReceptionThreadsListType }
*/
public ReceptionThreadsListType createReceptionThreadsListType() {
return new ReceptionThreadsListType();
}
/**
* Create an instance of {@link PartitionQosPolicyType.Names }
*
* @return
* the new instance of {@link PartitionQosPolicyType.Names }
*/
public PartitionQosPolicyType.Names createPartitionQosPolicyTypeNames() {
return new PartitionQosPolicyType.Names();
}
/**
* Create an instance of {@link DataSharingQosPolicyType.DomainIds }
*
* @return
* the new instance of {@link DataSharingQosPolicyType.DomainIds }
*/
public DataSharingQosPolicyType.DomainIds createDataSharingQosPolicyTypeDomainIds() {
return new DataSharingQosPolicyType.DomainIds();
}
/**
* Create an instance of {@link PropertyPolicyType.Properties }
*
* @return
* the new instance of {@link PropertyPolicyType.Properties }
*/
public PropertyPolicyType.Properties createPropertyPolicyTypeProperties() {
return new PropertyPolicyType.Properties();
}
/**
* Create an instance of {@link PropertyPolicyType.BinaryProperties }
*
* @return
* the new instance of {@link PropertyPolicyType.BinaryProperties }
*/
public PropertyPolicyType.BinaryProperties createPropertyPolicyTypeBinaryProperties() {
return new PropertyPolicyType.BinaryProperties();
}
/**
* Create an instance of {@link LocatorListType.Locator }
*
* @return
* the new instance of {@link LocatorListType.Locator }
*/
public LocatorListType.Locator createLocatorListTypeLocator() {
return new LocatorListType.Locator();
}
/**
* Create an instance of {@link TlsConfigType.VerifyMode }
*
* @return
* the new instance of {@link TlsConfigType.VerifyMode }
*/
public TlsConfigType.VerifyMode createTlsConfigTypeVerifyMode() {
return new TlsConfigType.VerifyMode();
}
/**
* Create an instance of {@link TlsConfigType.VerifyPaths }
*
* @return
* the new instance of {@link TlsConfigType.VerifyPaths }
*/
public TlsConfigType.VerifyPaths createTlsConfigTypeVerifyPaths() {
return new TlsConfigType.VerifyPaths();
}
/**
* Create an instance of {@link TlsConfigType.Options }
*
* @return
* the new instance of {@link TlsConfigType.Options }
*/
public TlsConfigType.Options createTlsConfigTypeOptions() {
return new TlsConfigType.Options();
}
/**
* Create an instance of {@link BlocklistType.Interface }
*
* @return
* the new instance of {@link BlocklistType.Interface }
*/
public BlocklistType.Interface createBlocklistTypeInterface() {
return new BlocklistType.Interface();
}
/**
* Create an instance of {@link AllowlistType.Interface }
*
* @return
* the new instance of {@link AllowlistType.Interface }
*/
public AllowlistType.Interface createAllowlistTypeInterface() {
return new AllowlistType.Interface();
}
/**
* Create an instance of {@link TransportDescriptorType.InterfaceWhiteList }
*
* @return
* the new instance of {@link TransportDescriptorType.InterfaceWhiteList }
*/
public TransportDescriptorType.InterfaceWhiteList createTransportDescriptorTypeInterfaceWhiteList() {
return new TransportDescriptorType.InterfaceWhiteList();
}
/**
* Create an instance of {@link TransportDescriptorType.ListeningPorts }
*
* @return
* the new instance of {@link TransportDescriptorType.ListeningPorts }
*/
public TransportDescriptorType.ListeningPorts createTransportDescriptorTypeListeningPorts() {
return new TransportDescriptorType.ListeningPorts();
}
/**
* Create an instance of {@link RtpsParticipantAllocationAttributesType.RemoteLocators }
*
* @return
* the new instance of {@link RtpsParticipantAllocationAttributesType.RemoteLocators }
*/
public RtpsParticipantAllocationAttributesType.RemoteLocators createRtpsParticipantAllocationAttributesTypeRemoteLocators() {
return new RtpsParticipantAllocationAttributesType.RemoteLocators();
}
/**
* Create an instance of {@link RtpsParticipantAllocationAttributesType.SendBuffers }
*
* @return
* the new instance of {@link RtpsParticipantAllocationAttributesType.SendBuffers }
*/
public RtpsParticipantAllocationAttributesType.SendBuffers createRtpsParticipantAllocationAttributesTypeSendBuffers() {
return new RtpsParticipantAllocationAttributesType.SendBuffers();
}
/**
* Create an instance of {@link BitmaskDcl.BitValue }
*
* @return
* the new instance of {@link BitmaskDcl.BitValue }
*/
public BitmaskDcl.BitValue createBitmaskDclBitValue() {
return new BitmaskDcl.BitValue();
}
/**
* Create an instance of {@link BitsetDcl.Bitfield }
*
* @return
* the new instance of {@link BitsetDcl.Bitfield }
*/
public BitsetDcl.Bitfield createBitsetDclBitfield() {
return new BitsetDcl.Bitfield();
}
/**
* Create an instance of {@link UnionDcl.Discriminator }
*
* @return
* the new instance of {@link UnionDcl.Discriminator }
*/
public UnionDcl.Discriminator createUnionDclDiscriminator() {
return new UnionDcl.Discriminator();
}
/**
* Create an instance of {@link UnionDcl.Case.CaseDiscriminator }
*
* @return
* the new instance of {@link UnionDcl.Case.CaseDiscriminator }
*/
public UnionDcl.Case.CaseDiscriminator createUnionDclCaseCaseDiscriminator() {
return new UnionDcl.Case.CaseDiscriminator();
}
/**
* Create an instance of {@link EnumDcl.Enumerator }
*
* @return
* the new instance of {@link EnumDcl.Enumerator }
*/
public EnumDcl.Enumerator createEnumDclEnumerator() {
return new EnumDcl.Enumerator();
}
/**
* Create an instance of {@link TopicElementType.ResourceLimitsQos }
*
* @return
* the new instance of {@link TopicElementType.ResourceLimitsQos }
*/
public TopicElementType.ResourceLimitsQos createTopicElementTypeResourceLimitsQos() {
return new TopicElementType.ResourceLimitsQos();
}
/**
* Create an instance of {@link TopicProfileType.ResourceLimitsQos }
*
* @return
* the new instance of {@link TopicProfileType.ResourceLimitsQos }
*/
public TopicProfileType.ResourceLimitsQos createTopicProfileTypeResourceLimitsQos() {
return new TopicProfileType.ResourceLimitsQos();
}
/**
* Create an instance of {@link ParticipantProfileType.Rtps.UserTransports }
*
* @return
* the new instance of {@link ParticipantProfileType.Rtps.UserTransports }
*/
public ParticipantProfileType.Rtps.UserTransports createParticipantProfileTypeRtpsUserTransports() {
return new ParticipantProfileType.Rtps.UserTransports();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ProfilesType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ProfilesType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", name = "profiles")
public JAXBElement createProfiles(ProfilesType value) {
return new JAXBElement<>(_Profiles_QNAME, ProfilesType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link TypesType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link TypesType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", name = "types")
public JAXBElement createTypes(TypesType value) {
return new JAXBElement<>(_Types_QNAME, TypesType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LogType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LogType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", name = "log")
public JAXBElement createLog(LogType value) {
return new JAXBElement<>(_Log_QNAME, LogType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LibrarySettingsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LibrarySettingsType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", name = "library_settings")
public JAXBElement createLibrarySettings(LibrarySettingsType value) {
return new JAXBElement<>(_LibrarySettings_QNAME, LibrarySettingsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", name = "sec", scope = DurationType.class)
public JAXBElement createDurationTypeSec(String value) {
return new JAXBElement<>(_DurationTypeSec_QNAME, String.class, DurationType.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", name = "nanosec", scope = DurationType.class)
public JAXBElement createDurationTypeNanosec(String value) {
return new JAXBElement<>(_DurationTypeNanosec_QNAME, String.class, DurationType.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link List }{@code <}{@link String }{@code >}{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link List }{@code <}{@link String }{@code >}{@code >}
*/
@XmlElementDecl(namespace = "http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", name = "address", scope = TransportDescriptorType.InterfaceWhiteList.class)
public JAXBElement> createTransportDescriptorTypeInterfaceWhiteListAddress(List value) {
return new JAXBElement<>(_TransportDescriptorTypeInterfaceWhiteListAddress_QNAME, ((Class) List.class), TransportDescriptorType.InterfaceWhiteList.class, ((List ) value));
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", name = "interface", scope = TransportDescriptorType.InterfaceWhiteList.class)
public JAXBElement createTransportDescriptorTypeInterfaceWhiteListInterface(String value) {
return new JAXBElement<>(_TransportDescriptorTypeInterfaceWhiteListInterface_QNAME, String.class, TransportDescriptorType.InterfaceWhiteList.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocatorListType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocatorListType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", name = "metatrafficUnicastLocatorList", scope = RemoteServerAttributesType.class)
public JAXBElement createRemoteServerAttributesTypeMetatrafficUnicastLocatorList(LocatorListType value) {
return new JAXBElement<>(_RemoteServerAttributesTypeMetatrafficUnicastLocatorList_QNAME, LocatorListType.class, RemoteServerAttributesType.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocatorListType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocatorListType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.eprosima.com/XMLSchemas/fastRTPS_Profiles", name = "metatrafficMulticastLocatorList", scope = RemoteServerAttributesType.class)
public JAXBElement createRemoteServerAttributesTypeMetatrafficMulticastLocatorList(LocatorListType value) {
return new JAXBElement<>(_RemoteServerAttributesTypeMetatrafficMulticastLocatorList_QNAME, LocatorListType.class, RemoteServerAttributesType.class, value);
}
}