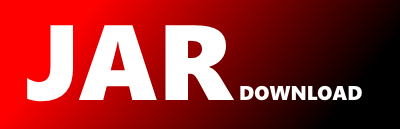
us.ihmc.pubsub.attributes.DDSConversionTools Maven / Gradle / Ivy
package us.ihmc.pubsub.attributes;
import com.eprosima.xmlschemas.fastrtps_profiles.DurationType;
import us.ihmc.pubsub.common.Time;
import us.ihmc.pubsub.impl.fastRTPS.FastRTPSDomain;
import jakarta.xml.bind.JAXBElement;
import javax.xml.namespace.QName;
/**
* Helper class to convert between different formats inside the DDS layer
*
* Mostly used for Time
*
* @author jesper
*/
public class DDSConversionTools
{
/**
* Convert Time to the XML durationType
* @param Time
* @return DurationType
*/
public static DurationType timeToDurationType(Time time)
{
DurationType dt = new DurationType();
JAXBElement nanosec = new JAXBElement<>(new QName(FastRTPSDomain.FAST_DDS_XML_NAMESPACE, FastRTPSDomain.FAST_DDS_NANOSEC), String.class, Long.toString(time.getNanoseconds()));
JAXBElement sec = new JAXBElement<>(new QName(FastRTPSDomain.FAST_DDS_XML_NAMESPACE, FastRTPSDomain.FAST_DDS_SEC), String.class, Integer.toString(time.getSeconds()));
dt.getSecOrNanosec().add(nanosec);
dt.getSecOrNanosec().add(sec);
return dt;
}
/**
* Convert an XML duration type to Time
*
* @param duration
* @return Time
*/
public static Time durationTypeToTime(DurationType duration)
{
if(duration == null)
{
return null;
}
Time time = new Time();
for (JAXBElement> e : duration.getSecOrNanosec())
{
switch (e.getName().getLocalPart())
{
case FastRTPSDomain.FAST_DDS_NANOSEC -> time.setNanoseconds(Long.parseLong(e.getValue().toString()));
case FastRTPSDomain.FAST_DDS_SEC -> time.setSeconds(Integer.parseInt(e.getValue().toString()));
}
}
return time;
}
/**
* Create a time element based on time in seconds
*
* @param timeInSeconds
* @return Time for DDS
*/
public static Time createTime(double timeInSeconds)
{
Time time = new Time();
int seconds = (int) timeInSeconds;
double remainder = timeInSeconds - seconds;
time.setSeconds((int) timeInSeconds);
time.setNanoseconds((long)(remainder * 1e9));
return time;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy