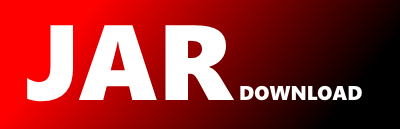
us.ihmc.scs2.simulation.parameters.ContactParametersBasics Maven / Gradle / Ivy
package us.ihmc.scs2.simulation.parameters;
import us.ihmc.scs2.simulation.physicsEngine.impulseBased.ImpulseBasedPhysicsEngine;
/**
* Write and read interface for accessing/modifying a set of parameters used for resolving contacts
* between pairs of collidables, see {@link ImpulseBasedPhysicsEngine}.
*
* @author Sylvain Bertrand
*/
public interface ContactParametersBasics extends ContactParametersReadOnly, ConstraintParametersBasics
{
default void set(ContactParametersReadOnly other)
{
ConstraintParametersBasics.super.set(other);
setMinimumPenetration(other.getMinimumPenetration());
setCoefficientOfFriction(other.getCoefficientOfFriction());
setComputeFrictionMoment(other.getComputeFrictionMoment());
setCoulombMomentFrictionRatio(other.getCoulombMomentFrictionRatio());
}
/**
* Sets the minimum distance by which two collidable should be penetrating each other before
* resolving the contact.
*
* Ideally the contact should be resolved when the collidables are touching. However, when only
* touching, it is impossible to estimate the contact normal which is essential to solving the
* problem. By letting the collidables penetrate a little, this allows to estimate the contact
* normal. A larger minimum penetration implies greater robustness to numerical errors when
* estimating the normal.
*
*
* @param minimumPenetration the distance before resolving the contact, recommended ~1.0e-5.
*/
void setMinimumPenetration(double minimumPenetration);
/**
* Sets the coefficient of friction.
*
* Assuming the Coulomb friction model is used, the coefficient of friction μ defines
* the relationship between normal contact force Fn and maximum achievable
* tangential contact force Ft:
*
*
* |Ft| ≤ μ Fn
*
*
*
* The value of the coefficient of friction is commonly in [0, 1]:
*
* - μ = 0: frictionless contacts, zero resistance to sliding is added when resolving
* contact between two collidables.
*
- μ ∈ [0, 1]: "real-world" friction, the amount of the resistance to sliding is
* non-negligible but still limited.
*
*
*
* Note that the same coefficient is used for both static and sliding contacts.
*
*
* @param coefficientOfFriction the coefficient of friction, recommended 0.7.
*/
void setCoefficientOfFriction(double coefficientOfFriction);
/**
* Sets whether a moment-impulse of friction should be calculated alongside the usual linear
* impulse.
*
* When enabled, only a moment around the normal axis of an active contact is computed with the goal
* of canceling the angular velocity around the normal axis.
*
*
* @param computeFrictionMoment {@code true} to enable the friction moment calculation,
* {@code false} to disable it.
*/
void setComputeFrictionMoment(boolean computeFrictionMoment);
/**
* When computing the moment-impulse of friction for a contact, then Coulomb friction is replaced by
* an elliptic law as follows:
*
*
* Fx2/ex2 + Fy2/ey2 + Tz2/ezz2 ≤ μFz2
*
*
* where Fx and Fy are the tangential forces,
* Tz the normal moment, Fz the normal force, and
* μ the coefficient of friction. ei are positive constants defined by
* the user.
*
* In the current implementation of the solver, ex = ey = 1 and only ezz
* is the ratio set by this method.
*
*
* @param coulombFrictionMomentRatio the value to use for ezz, default is
* {@code 0.3}.
*/
void setCoulombMomentFrictionRatio(double coulombFrictionMomentRatio);
}