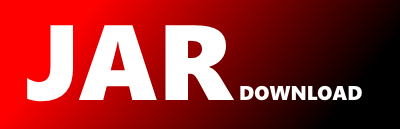
us.ihmc.simulationconstructionset.gui.SwingWorker Maven / Gradle / Ivy
package us.ihmc.simulationconstructionset.gui;
//import com.sun.java.swing.SwingUtilities; //old package name
import javax.swing.SwingUtilities;
/**
* An abstract class that you subclass to perform GUI-related work in a dedicated thread. For
* instructions on using this class, see
* http://java.sun.com/products/jfc/swingdoc-current/threads2.html
*/
public abstract class SwingWorker
{
private Object value; // see getValue(), setValue()
@SuppressWarnings("unused")
private Thread thread;
/**
* Class to maintain reference to current worker thread under separate synchronization control.
*/
private static class ThreadVar
{
private Thread thread;
ThreadVar(Thread t)
{
thread = t;
}
synchronized Thread get()
{
return thread;
}
synchronized void clear()
{
thread = null;
}
}
private ThreadVar threadVar;
/**
* Get the value produced by the worker thread, or null if it hasn't been constructed yet.
*/
protected synchronized Object getValue()
{
return value;
}
/**
* Set the value produced by worker thread
*/
private synchronized void setValue(Object x)
{
value = x;
}
/**
* Compute the value to be returned by the get
method.
*/
public abstract Object construct();
/**
* Called on the event dispatching thread (not on the worker thread) after the
* construct
method has returned.
*/
public void finished()
{
}
/**
* A new method that interrupts the worker thread. Call this method to force the worker to abort
* what it's doing.
*/
public void interrupt()
{
Thread t = threadVar.get();
if (t != null)
{
t.interrupt();
}
threadVar.clear();
}
/**
* Return the value created by the construct
method. Returns null if either the
* constructing thread or the current thread was interrupted before a value was produced.
*
* @return the value created by the construct
method
*/
public Object get()
{
while (true)
{
Thread t = threadVar.get();
if (t == null)
{
return getValue();
}
try
{
t.join();
}
catch (InterruptedException e)
{
Thread.currentThread().interrupt(); // propagate
return null;
}
}
}
/**
* Start a thread that will call the construct
method and then exit.
*/
public SwingWorker()
{
final Runnable doFinished = new Runnable()
{
@Override
public void run()
{
finished();
}
};
Runnable doConstruct = new Runnable()
{
@Override
public void run()
{
try
{
setValue(construct());
}
finally
{
threadVar.clear();
}
SwingUtilities.invokeLater(doFinished);
}
};
Thread t = new Thread(doConstruct, "SCSSwingWorkerConstruct");
threadVar = new ThreadVar(t);
t.start();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy