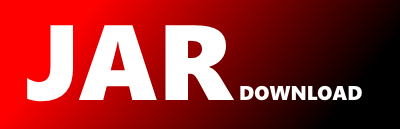
javadoc.com.google.common.math.IntMath.html Maven / Gradle / Ivy
IntMath (Guava: Google Core Libraries for Java 11.0.1 API)
Overview
Package
Class
Use
Tree
Deprecated
Index
Help
PREV CLASS
NEXT CLASS
FRAMES
NO FRAMES
SUMMARY: NESTED | FIELD | CONSTR | METHOD
DETAIL: FIELD | CONSTR | METHOD
com.google.common.math
Class IntMath
java.lang.Object
com.google.common.math.IntMath
@Beta
@GwtCompatible(emulated=true)
public final class IntMath
- extends Object
A class for arithmetic on values of type int
. Where possible, methods are defined and
named analogously to their BigInteger
counterparts.
The implementations of many methods in this class are based on material from Henry S. Warren, Jr.'s Hacker's Delight, (Addison Wesley, 2002).
Similar functionality for long
and for BigInteger
can be found in
LongMath
and BigIntegerMath
respectively. For other common operations on
int
values, see Ints
.
- Since:
- 11.0
- Author:
- Louis Wasserman
Method Summary | |
---|---|
static int |
binomial(int n,
int k)
Returns n choose k , also known as the binomial coefficient of n and
k , or Integer.MAX_VALUE if the result does not fit in an int . |
static int |
checkedAdd(int a,
int b)
Returns the sum of a and b , provided it does not overflow. |
static int |
checkedMultiply(int a,
int b)
Returns the product of a and b , provided it does not overflow. |
static int |
checkedPow(int b,
int k)
Returns the b to the k th power, provided it does not overflow. |
static int |
checkedSubtract(int a,
int b)
Returns the difference of a and b , provided it does not overflow. |
static int |
divide(int p,
int q,
RoundingMode mode)
Returns the result of dividing p by q , rounding using the specified
RoundingMode . |
static int |
factorial(int n)
Returns n! , that is, the product of the first n positive
integers, 1 if n == 0 , or Integer.MAX_VALUE if the
result does not fit in a int . |
static int |
gcd(int a,
int b)
Returns the greatest common divisor of a, b . |
static boolean |
isPowerOfTwo(int x)
Returns true if x represents a power of two. |
static int |
log10(int x,
RoundingMode mode)
Returns the base-10 logarithm of x , rounded according to the specified rounding mode. |
static int |
log2(int x,
RoundingMode mode)
Returns the base-2 logarithm of x , rounded according to the specified rounding mode. |
static int |
mod(int x,
int m)
Returns x mod m . |
static int |
pow(int b,
int k)
Returns b to the k th power. |
static int |
sqrt(int x,
RoundingMode mode)
Returns the square root of x , rounded with the specified rounding mode. |
Methods inherited from class java.lang.Object |
---|
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait |
Method Detail |
---|
isPowerOfTwo
public static boolean isPowerOfTwo(int x)
- Returns
true
ifx
represents a power of two.This differs from
Integer.bitCount(x) == 1
, becauseInteger.bitCount(Integer.MIN_VALUE) == 1
, butInteger.MIN_VALUE
is not a power of two.
log2
@GwtIncompatible(value="need BigIntegerMath to adequately test") public static int log2(int x, RoundingMode mode)
- Returns the base-2 logarithm of
x
, rounded according to the specified rounding mode.- Throws:
IllegalArgumentException
- ifx <= 0
ArithmeticException
- ifmode
isRoundingMode.UNNECESSARY
andx
is not a power of two
log10
@GwtIncompatible(value="need BigIntegerMath to adequately test") public static int log10(int x, RoundingMode mode)
- Returns the base-10 logarithm of
x
, rounded according to the specified rounding mode.- Throws:
IllegalArgumentException
- ifx <= 0
ArithmeticException
- ifmode
isRoundingMode.UNNECESSARY
andx
is not a power of ten
pow
@GwtIncompatible(value="failing tests") public static int pow(int b, int k)
- Returns
b
to thek
th power. Even if the result overflows, it will be equal toBigInteger.valueOf(b).pow(k).intValue()
. This implementation runs inO(log k)
time.Compare
checkedPow(int, int)
, which throws anArithmeticException
upon overflow.- Throws:
IllegalArgumentException
- ifk < 0
sqrt
@GwtIncompatible(value="need BigIntegerMath to adequately test") public static int sqrt(int x, RoundingMode mode)
- Returns the square root of
x
, rounded with the specified rounding mode.- Throws:
IllegalArgumentException
- ifx < 0
ArithmeticException
- ifmode
isRoundingMode.UNNECESSARY
andsqrt(x)
is not an integer
divide
@GwtIncompatible(value="failing tests") public static int divide(int p, int q, RoundingMode mode)
- Returns the result of dividing
p
byq
, rounding using the specifiedRoundingMode
.- Throws:
ArithmeticException
- ifq == 0
, or ifmode == UNNECESSARY
anda
is not an integer multiple ofb
mod
public static int mod(int x, int m)
- Returns
x mod m
. This differs fromx % m
in that it always returns a non-negative result.For example:
mod(7, 4) == 3 mod(-7, 4) == 1 mod(-1, 4) == 3 mod(-8, 4) == 0 mod(8, 4) == 0
- Throws:
ArithmeticException
- ifm <= 0
gcd
public static int gcd(int a, int b)
- Returns the greatest common divisor of
a, b
. Returns0
ifa == 0 && b == 0
.- Throws:
IllegalArgumentException
- ifa < 0
orb < 0
checkedAdd
public static int checkedAdd(int a, int b)
- Returns the sum of
a
andb
, provided it does not overflow.- Throws:
ArithmeticException
- ifa + b
overflows in signedint
arithmetic
checkedSubtract
public static int checkedSubtract(int a, int b)
- Returns the difference of
a
andb
, provided it does not overflow.- Throws:
ArithmeticException
- ifa - b
overflows in signedint
arithmetic
checkedMultiply
public static int checkedMultiply(int a, int b)
- Returns the product of
a
andb
, provided it does not overflow.- Throws:
ArithmeticException
- ifa * b
overflows in signedint
arithmetic
checkedPow
@GwtIncompatible(value="failing tests") public static int checkedPow(int b, int k)
- Returns the
b
to thek
th power, provided it does not overflow.pow(int, int)
may be faster, but does not check for overflow.- Throws:
ArithmeticException
- ifb
to thek
th power overflows in signedint
arithmetic
factorial
@GwtIncompatible(value="need BigIntegerMath to adequately test") public static int factorial(int n)
- Returns
n!
, that is, the product of the firstn
positive integers,1
ifn == 0
, orInteger.MAX_VALUE
if the result does not fit in aint
.- Throws:
IllegalArgumentException
- ifn < 0
binomial
@GwtIncompatible(value="need BigIntegerMath to adequately test") public static int binomial(int n, int k)
- Returns
n
choosek
, also known as the binomial coefficient ofn
andk
, orInteger.MAX_VALUE
if the result does not fit in anint
.- Throws:
IllegalArgumentException
- ifn < 0
,k < 0
ork > n
|
||||||||||
PREV CLASS NEXT CLASS | FRAMES NO FRAMES | |||||||||
SUMMARY: NESTED | FIELD | CONSTR | METHOD | DETAIL: FIELD | CONSTR | METHOD |
Copyright © 2010-2012. All Rights Reserved.