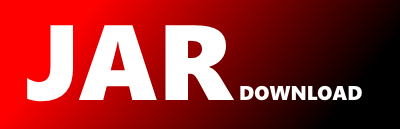
javadoc.com.google.common.math.LongMath.html Maven / Gradle / Ivy
LongMath (Guava: Google Core Libraries for Java 11.0.1 API)
Overview
Package
Class
Use
Tree
Deprecated
Index
Help
PREV CLASS
NEXT CLASS
FRAMES
NO FRAMES
SUMMARY: NESTED | FIELD | CONSTR | METHOD
DETAIL: FIELD | CONSTR | METHOD
com.google.common.math
Class LongMath
java.lang.Object
com.google.common.math.LongMath
A class for arithmetic on values of type long
. Where possible, methods are defined and
named analogously to their BigInteger
counterparts.
The implementations of many methods in this class are based on material from Henry S. Warren, Jr.'s Hacker's Delight, (Addison Wesley, 2002).
Similar functionality for int
and for BigInteger
can be found in
IntMath
and BigIntegerMath
respectively. For other common operations on
long
values, see Longs
.
- Since:
- 11.0
- Author:
- Louis Wasserman
Method Summary | |
---|---|
static long |
binomial(int n,
int k)
Returns n choose k , also known as the binomial coefficient of n and
k , or Long.MAX_VALUE if the result does not fit in a long . |
static long |
checkedAdd(long a,
long b)
Returns the sum of a and b , provided it does not overflow. |
static long |
checkedMultiply(long a,
long b)
Returns the product of a and b , provided it does not overflow. |
static long |
checkedPow(long b,
int k)
Returns the b to the k th power, provided it does not overflow. |
static long |
checkedSubtract(long a,
long b)
Returns the difference of a and b , provided it does not overflow. |
static long |
divide(long p,
long q,
RoundingMode mode)
Returns the result of dividing p by q , rounding using the specified
RoundingMode . |
static long |
factorial(int n)
Returns n! , that is, the product of the first n positive
integers, 1 if n == 0 , or Long.MAX_VALUE if the
result does not fit in a long . |
static long |
gcd(long a,
long b)
Returns the greatest common divisor of a, b . |
static boolean |
isPowerOfTwo(long x)
Returns true if x represents a power of two. |
static int |
log10(long x,
RoundingMode mode)
Returns the base-10 logarithm of x , rounded according to the specified rounding mode. |
static int |
log2(long x,
RoundingMode mode)
Returns the base-2 logarithm of x , rounded according to the specified rounding mode. |
static int |
mod(long x,
int m)
Returns x mod m . |
static long |
mod(long x,
long m)
Returns x mod m . |
static long |
pow(long b,
int k)
Returns b to the k th power. |
static long |
sqrt(long x,
RoundingMode mode)
Returns the square root of x , rounded with the specified rounding mode. |
Methods inherited from class java.lang.Object |
---|
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait |
Method Detail |
---|
isPowerOfTwo
public static boolean isPowerOfTwo(long x)
- Returns
true
ifx
represents a power of two.This differs from
Long.bitCount(x) == 1
, becauseLong.bitCount(Long.MIN_VALUE) == 1
, butLong.MIN_VALUE
is not a power of two.
log2
public static int log2(long x, RoundingMode mode)
- Returns the base-2 logarithm of
x
, rounded according to the specified rounding mode.- Throws:
IllegalArgumentException
- ifx <= 0
ArithmeticException
- ifmode
isRoundingMode.UNNECESSARY
andx
is not a power of two
log10
public static int log10(long x, RoundingMode mode)
- Returns the base-10 logarithm of
x
, rounded according to the specified rounding mode.- Throws:
IllegalArgumentException
- ifx <= 0
ArithmeticException
- ifmode
isRoundingMode.UNNECESSARY
andx
is not a power of ten
pow
public static long pow(long b, int k)
- Returns
b
to thek
th power. Even if the result overflows, it will be equal toBigInteger.valueOf(b).pow(k).longValue()
. This implementation runs inO(log k)
time.- Throws:
IllegalArgumentException
- ifk < 0
sqrt
public static long sqrt(long x, RoundingMode mode)
- Returns the square root of
x
, rounded with the specified rounding mode.- Throws:
IllegalArgumentException
- ifx < 0
ArithmeticException
- ifmode
isRoundingMode.UNNECESSARY
andsqrt(x)
is not an integer
divide
public static long divide(long p, long q, RoundingMode mode)
- Returns the result of dividing
p
byq
, rounding using the specifiedRoundingMode
.- Throws:
ArithmeticException
- ifq == 0
, or ifmode == UNNECESSARY
anda
is not an integer multiple ofb
mod
public static int mod(long x, int m)
- Returns
x mod m
. This differs fromx % m
in that it always returns a non-negative result.For example:
mod(7, 4) == 3 mod(-7, 4) == 1 mod(-1, 4) == 3 mod(-8, 4) == 0 mod(8, 4) == 0
- Throws:
ArithmeticException
- ifm <= 0
mod
public static long mod(long x, long m)
- Returns
x mod m
. This differs fromx % m
in that it always returns a non-negative result.For example:
mod(7, 4) == 3 mod(-7, 4) == 1 mod(-1, 4) == 3 mod(-8, 4) == 0 mod(8, 4) == 0
- Throws:
ArithmeticException
- ifm <= 0
gcd
public static long gcd(long a, long b)
- Returns the greatest common divisor of
a, b
. Returns0
ifa == 0 && b == 0
.- Throws:
IllegalArgumentException
- ifa < 0
orb < 0
checkedAdd
public static long checkedAdd(long a, long b)
- Returns the sum of
a
andb
, provided it does not overflow.- Throws:
ArithmeticException
- ifa + b
overflows in signedlong
arithmetic
checkedSubtract
public static long checkedSubtract(long a, long b)
- Returns the difference of
a
andb
, provided it does not overflow.- Throws:
ArithmeticException
- ifa - b
overflows in signedlong
arithmetic
checkedMultiply
public static long checkedMultiply(long a, long b)
- Returns the product of
a
andb
, provided it does not overflow.- Throws:
ArithmeticException
- ifa * b
overflows in signedlong
arithmetic
checkedPow
public static long checkedPow(long b, int k)
- Returns the
b
to thek
th power, provided it does not overflow.- Throws:
ArithmeticException
- ifb
to thek
th power overflows in signedlong
arithmetic
factorial
public static long factorial(int n)
- Returns
n!
, that is, the product of the firstn
positive integers,1
ifn == 0
, orLong.MAX_VALUE
if the result does not fit in along
.- Throws:
IllegalArgumentException
- ifn < 0
binomial
public static long binomial(int n, int k)
- Returns
n
choosek
, also known as the binomial coefficient ofn
andk
, orLong.MAX_VALUE
if the result does not fit in along
.- Throws:
IllegalArgumentException
- ifn < 0
,k < 0
, ork > n
|
||||||||||
PREV CLASS NEXT CLASS | FRAMES NO FRAMES | |||||||||
SUMMARY: NESTED | FIELD | CONSTR | METHOD | DETAIL: FIELD | CONSTR | METHOD |
Copyright © 2010-2012. All Rights Reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy